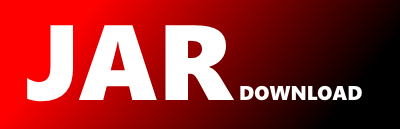
pascal.taie.analysis.pta.plugin.taint.SinkHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tai-e Show documentation
Show all versions of tai-e Show documentation
An easy-to-learn/use static analysis framework for Java
The newest version!
/*
* Tai-e: A Static Analysis Framework for Java
*
* Copyright (C) 2022 Tian Tan
* Copyright (C) 2022 Yue Li
*
* This file is part of Tai-e.
*
* Tai-e is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3
* of the License, or (at your option) any later version.
*
* Tai-e is distributed in the hope that it will be useful,but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Tai-e. If not, see .
*/
package pascal.taie.analysis.pta.plugin.taint;
import pascal.taie.analysis.graph.callgraph.CallKind;
import pascal.taie.analysis.graph.callgraph.Edge;
import pascal.taie.analysis.pta.PointerAnalysisResult;
import pascal.taie.analysis.pta.core.cs.element.ArrayIndex;
import pascal.taie.analysis.pta.core.cs.element.CSObj;
import pascal.taie.analysis.pta.core.cs.element.InstanceField;
import pascal.taie.analysis.pta.core.cs.element.Pointer;
import pascal.taie.analysis.pta.core.heap.Obj;
import pascal.taie.analysis.pta.plugin.util.InvokeUtils;
import pascal.taie.ir.exp.Var;
import pascal.taie.ir.stmt.Invoke;
import pascal.taie.language.classes.JMethod;
import pascal.taie.util.collection.MultiMap;
import pascal.taie.util.collection.MultiMapCollector;
import pascal.taie.util.collection.Sets;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
/**
* Handles sinks in taint analysis.
*/
class SinkHandler extends Handler {
private final List sinks;
SinkHandler(HandlerContext context) {
super(context);
sinks = context.config().sinks();
}
Set collectTaintFlows() {
PointerAnalysisResult result = solver.getResult();
Set taintFlows = Sets.newOrderedSet();
for (Sink sink : sinks) {
result.getCallGraph()
.edgesInTo(sink.method())
// TODO: handle other call edges
.filter(e -> e.getKind() != CallKind.OTHER)
.map(Edge::getCallSite)
.map(sinkCall -> collectTaintFlows(sinkCall, sink))
.forEach(taintFlows::addAll);
}
if (callSiteMode) {
MultiMap sinkMap = sinks.stream()
.collect(MultiMapCollector.get(Sink::method, s -> s));
// scan all reachable call sites to search sink calls
result.getCallGraph()
.reachableMethods()
.flatMap(m -> m.getIR().invokes(false))
.forEach(callSite -> {
JMethod callee = callSite.getMethodRef().resolveNullable();
if (callee != null) {
for (Sink sink : sinkMap.get(callee)) {
taintFlows.addAll(collectTaintFlows(callSite, sink));
}
}
});
}
return taintFlows;
}
private Set collectTaintFlows(
Invoke sinkCall, Sink sink) {
IndexRef indexRef = sink.indexRef();
Var arg = InvokeUtils.getVar(sinkCall, indexRef.index());
SinkPoint sinkPoint = new SinkPoint(sinkCall, indexRef, sink);
// obtain objects to check for different IndexRef.Kind
Set objs = switch (indexRef.kind()) {
case VAR -> csManager.getCSVarsOf(arg)
.stream()
.flatMap(Pointer::objects)
.map(CSObj::getObject)
.collect(Collectors.toUnmodifiableSet());
case ARRAY -> csManager.getCSVarsOf(arg)
.stream()
.flatMap(Pointer::objects)
.map(csManager::getArrayIndex)
.flatMap(ArrayIndex::objects)
.map(CSObj::getObject)
.collect(Collectors.toUnmodifiableSet());
case FIELD -> csManager.getCSVarsOf(arg)
.stream()
.flatMap(Pointer::objects)
.map(o -> csManager.getInstanceField(o, indexRef.field()))
.flatMap(InstanceField::objects)
.map(CSObj::getObject)
.collect(Collectors.toUnmodifiableSet());
};
return objs.stream()
.filter(manager::isTaint)
.map(manager::getSourcePoint)
.map(sourcePoint -> new TaintFlow(sourcePoint, sinkPoint))
.collect(Collectors.toSet());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy