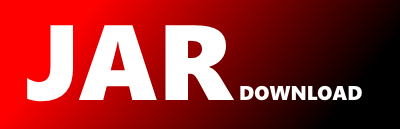
pascal.taie.analysis.pta.plugin.taint.TFGDumper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tai-e Show documentation
Show all versions of tai-e Show documentation
An easy-to-learn/use static analysis framework for Java
The newest version!
/*
* Tai-e: A Static Analysis Framework for Java
*
* Copyright (C) 2022 Tian Tan
* Copyright (C) 2022 Yue Li
*
* This file is part of Tai-e.
*
* Tai-e is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3
* of the License, or (at your option) any later version.
*
* Tai-e is distributed in the hope that it will be useful,but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Tai-e. If not, see .
*/
package pascal.taie.analysis.pta.plugin.taint;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import pascal.taie.analysis.graph.flowgraph.FlowEdge;
import pascal.taie.analysis.graph.flowgraph.InstanceFieldNode;
import pascal.taie.analysis.graph.flowgraph.Node;
import pascal.taie.analysis.graph.flowgraph.OtherFlowEdge;
import pascal.taie.analysis.graph.flowgraph.VarNode;
import pascal.taie.util.collection.Sets;
import pascal.taie.util.graph.DotAttributes;
import pascal.taie.util.graph.DotDumper;
import pascal.taie.util.graph.Edge;
import javax.annotation.Nullable;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Set;
import java.util.function.Function;
/**
* Taint flow graph dumper.
*/
class TFGDumper extends DotDumper {
private static final Logger logger = LogManager.getLogger(TFGDumper.class);
private final Set highlightNodes;
private TaintFlowGraph tfg;
TFGDumper() {
this(null);
}
TFGDumper(@Nullable String highlightPath) {
highlightNodes = Sets.newSet();
try {
if (highlightPath != null) {
highlightNodes.addAll(Files.readAllLines(Path.of(highlightPath)));
}
} catch (IOException e) {
logger.warn("Failed to read highlight nodes from {}",
highlightPath, e);
}
}
void dump(TaintFlowGraph tfg, File output) {
logger.info("Dumping {}", output.getAbsolutePath());
setNodeAttributer(this::nodeAttributer);
setEdgeAttributer(this::edgeAttributer);
this.tfg = tfg;
super.dump(tfg, output);
}
@Override
protected void dumpOthers() {
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy