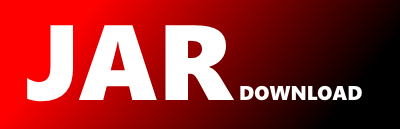
pascal.taie.analysis.pta.toolkit.PointerAnalysisResultEx Maven / Gradle / Ivy
Show all versions of tai-e Show documentation
/*
* Tai-e: A Static Analysis Framework for Java
*
* Copyright (C) 2022 Tian Tan
* Copyright (C) 2022 Yue Li
*
* This file is part of Tai-e.
*
* Tai-e is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3
* of the License, or (at your option) any later version.
*
* Tai-e is distributed in the hope that it will be useful,but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Tai-e. If not, see .
*/
package pascal.taie.analysis.pta.toolkit;
import pascal.taie.analysis.pta.PointerAnalysisResult;
import pascal.taie.analysis.pta.core.heap.Obj;
import pascal.taie.language.classes.JMethod;
import pascal.taie.language.type.Type;
import java.util.Set;
/**
* Extended version {@link PointerAnalysisResult}.
*
* Unlike {@link PointerAnalysisResult} which only provides results directly
* computed from pointer analysis, this class provides more commonly-used results
* that are indirectly derived from original pointer analysis result.
*/
public interface PointerAnalysisResultEx {
/**
* @return the base pointer analysis result.
*/
PointerAnalysisResult getBase();
/**
* @return the methods whose receiver objects contain obj.
*/
Set getMethodsInvokedOn(Obj obj);
/**
* @return the receiver objects of given method.
*/
Set getReceiverObjectsOf(JMethod method);
/**
* @return the objects that are allocated in given method.
*/
Set getObjectsAllocatedIn(JMethod method);
/**
* @return the objects of given type.
*/
Set getObjectsOf(Type type);
/**
* @return types of all reachable objects in pointer analysis.
*/
Set getObjectTypes();
}