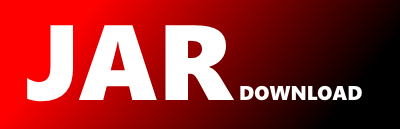
pascal.taie.analysis.pta.toolkit.mahjong.DFA Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tai-e Show documentation
Show all versions of tai-e Show documentation
An easy-to-learn/use static analysis framework for Java
The newest version!
/*
* Tai-e: A Static Analysis Framework for Java
*
* Copyright (C) 2022 Tian Tan
* Copyright (C) 2022 Yue Li
*
* This file is part of Tai-e.
*
* Tai-e is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3
* of the License, or (at your option) any later version.
*
* Tai-e is distributed in the hope that it will be useful,but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Tai-e. If not, see .
*/
package pascal.taie.analysis.pta.toolkit.mahjong;
import pascal.taie.language.type.Type;
import pascal.taie.util.collection.Sets;
import java.util.ArrayDeque;
import java.util.Map;
import java.util.Queue;
import java.util.Set;
class DFA {
private static final DFAState DEAD_STATE = new DFAState(Set.of(), Set.of());
private final DFAState q0;
private Set states, allStates;
DFA(DFAState q0) {
this.q0 = q0;
}
/**
* @return Set of states (excluding dead state).
*/
Set getStates() {
if (states == null) {
computeStates();
}
return states;
}
/**
* @return Set of all states (including dead state).
*/
Set getAllStates() {
if (allStates == null) {
computeStates();
}
return allStates;
}
private void computeStates() {
Queue queue = new ArrayDeque<>();
queue.add(q0);
states = Sets.newSet();
while (!queue.isEmpty()) {
DFAState s = queue.poll();
if (!states.contains(s)) {
states.add(s);
queue.addAll(s.getNextMap().values());
}
}
allStates = Sets.newSet(states);
allStates.add(DEAD_STATE);
}
DFAState getStartState() {
return q0;
}
DFAState getDeadState() {
return DEAD_STATE;
}
boolean isDeadState(DFAState s) {
return DEAD_STATE == s;
}
DFAState nextState(DFAState s, Field f) {
if (isDeadState(s)) {
return getDeadState();
}
Map nextMap = s.getNextMap();
if (nextMap.containsKey(f)) {
return nextMap.get(f);
}
return getDeadState();
}
Set outputOf(DFAState s) {
return s.getOutput();
}
Set outEdgesOf(DFAState s) {
Map nextMap = s.getNextMap();
return nextMap.keySet();
}
boolean containsState(DFAState s) {
return getAllStates().contains(s);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy