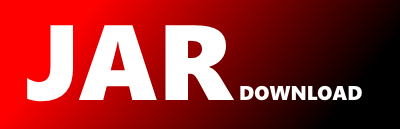
pascal.taie.language.classes.Reflections Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tai-e Show documentation
Show all versions of tai-e Show documentation
An easy-to-learn/use static analysis framework for Java
The newest version!
/*
* Tai-e: A Static Analysis Framework for Java
*
* Copyright (C) 2022 Tian Tan
* Copyright (C) 2022 Yue Li
*
* This file is part of Tai-e.
*
* Tai-e is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3
* of the License, or (at your option) any later version.
*
* Tai-e is distributed in the hope that it will be useful,but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Tai-e. If not, see .
*/
package pascal.taie.language.classes;
import pascal.taie.util.collection.Sets;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import java.util.stream.Stream;
/**
* Static utility methods for modeling the behaviors of reflection APIs.
*/
public final class Reflections {
private Reflections() {
}
public static Stream getDeclaredConstructors(JClass jclass) {
return jclass.getDeclaredMethods()
.stream()
.filter(JMethod::isConstructor);
}
public static Stream getConstructors(JClass jclass) {
return getDeclaredConstructors(jclass).filter(ClassMember::isPublic);
}
public static Stream getDeclaredMethods(JClass jclass, String methodName) {
return jclass.getDeclaredMethods()
.stream()
.filter(m -> m.getName().equals(methodName));
}
public static Stream getDeclaredMethods(JClass jclass) {
return jclass.getDeclaredMethods()
.stream()
.filter(m -> !m.isConstructor() && !m.isStaticInitializer());
}
public static Stream getMethods(JClass jclass, String methodName) {
List methods = new ArrayList<>();
Set subSignatures = Sets.newHybridSet();
while (jclass != null) {
jclass.getDeclaredMethods()
.stream()
.filter(m -> m.isPublic() && m.getName().equals(methodName))
.filter(m -> !subSignatures.contains(m.getSubsignature()))
.forEach(m -> {
methods.add(m);
subSignatures.add(m.getSubsignature());
});
jclass = jclass.getSuperClass();
}
return methods.stream();
}
public static Stream getMethods(JClass jclass) {
List methods = new ArrayList<>();
Set subSignatures = Sets.newHybridSet();
while (jclass != null) {
jclass.getDeclaredMethods()
.stream()
.filter(JMethod::isPublic)
.filter(m -> !m.isConstructor() && !m.isStaticInitializer())
.filter(m -> !subSignatures.contains(m.getSubsignature()))
.forEach(m -> {
methods.add(m);
subSignatures.add(m.getSubsignature());
});
jclass = jclass.getSuperClass();
}
return methods.stream();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy