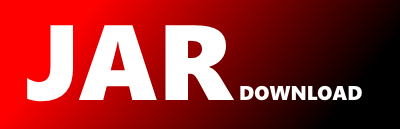
pascal.taie.language.generics.MethodGSignatureBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tai-e Show documentation
Show all versions of tai-e Show documentation
An easy-to-learn/use static analysis framework for Java
The newest version!
/*
* Tai-e: A Static Analysis Framework for Java
*
* Copyright (C) 2022 Tian Tan
* Copyright (C) 2022 Yue Li
*
* This file is part of Tai-e.
*
* Tai-e is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3
* of the License, or (at your option) any later version.
*
* Tai-e is distributed in the hope that it will be useful,but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Tai-e. If not, see .
*/
package pascal.taie.language.generics;
import org.objectweb.asm.signature.SignatureVisitor;
import java.util.ArrayList;
import java.util.List;
/**
* Builds a {@link MethodGSignature}.
*/
final class MethodGSignatureBuilder extends TypeParameterAwareGSignatureBuilder {
private MethodGSignature gSig;
private final List params = new ArrayList<>();
private TypeGSignature returnType;
private final List exceptionTypes = new ArrayList<>();
public MethodGSignature get() {
endExceptionType();
endReturnType();
if (gSig == null) {
gSig = new MethodGSignature(typeParams, params,
returnType, exceptionTypes);
}
return gSig;
}
@Override
public SignatureVisitor visitParameterType() {
endInterfaceBound();
endClassBound();
endTypeParam();
endParameterType();
stack.push(State.VISIT_PARAMETER_TYPE);
return newTypeGSignatureBuilder();
}
/**
* The valid automaton states for ending a {@link #visitParameterType()} method call are:
*
* - {@link #visitParameterType()}
* - {@link #visitReturnType()}
*
* When the automaton state is in one of these above states,
* it is time to collect the parameter type.
*/
private void endParameterType() {
if (stack.peek() == State.VISIT_PARAMETER_TYPE) {
stack.pop();
params.add(getTypeGSignature());
}
}
@Override
public SignatureVisitor visitReturnType() {
endInterfaceBound();
endClassBound();
endTypeParam();
endParameterType();
stack.push(State.VISIT_RETURN_TYPE);
return newTypeGSignatureBuilder();
}
/**
* The valid automaton states for ending a {@link #visitReturnType()} method call are:
*
* - {@link #visitExceptionType()}
* - {@link #get()}
*
* When the automaton state is in one of these above states,
* it is time to collect the return type.
*/
private void endReturnType() {
if (stack.peek() == State.VISIT_RETURN_TYPE) {
stack.pop();
returnType = getTypeGSignature();
}
}
@Override
public SignatureVisitor visitExceptionType() {
endReturnType();
endExceptionType();
stack.push(State.VISIT_EXCEPTION_TYPE);
return newTypeGSignatureBuilder();
}
/**
* The valid automaton states for ending a {@link #visitExceptionType()} method call are:
*
* - {@link #visitExceptionType()}
* - {@link #get()}
*
* When the automaton state is in one of these above states,
* it is time to collect the exception type.
*/
private void endExceptionType() {
if (stack.peek() == State.VISIT_EXCEPTION_TYPE) {
stack.pop();
exceptionTypes.add(getTypeGSignature());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy