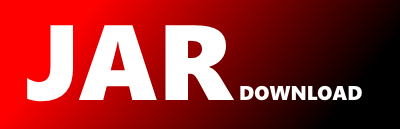
pascal.taie.util.ClassNameExtractor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tai-e Show documentation
Show all versions of tai-e Show documentation
An easy-to-learn/use static analysis framework for Java
The newest version!
/*
* Tai-e: A Static Analysis Framework for Java
*
* Copyright (C) 2022 Tian Tan
* Copyright (C) 2022 Yue Li
*
* This file is part of Tai-e.
*
* Tai-e is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3
* of the License, or (at your option) any later version.
*
* Tai-e is distributed in the hope that it will be useful,but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Tai-e. If not, see .
*/
package pascal.taie.util;
import pascal.taie.util.collection.Lists;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.PrintStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.jar.JarFile;
import java.util.stream.Stream;
/**
* Utility class for extracting names of all classes inside
* given JAR files or directories.
*/
public class ClassNameExtractor {
private static final String JAR = ".jar";
private static final String CLASS = ".class";
private static final String JAVA = ".java";
public static void main(String[] args) {
if (args.length == 0) {
System.out.println("Options: ...");
System.out.println(" can be a path to a JAR file or" +
" a directory containing classes");
return;
}
File outFile = new File(args[0]);
System.out.printf("Dumping extracted class names to %s%n",
outFile.getAbsolutePath());
String[] jars = Arrays.copyOfRange(args, 1, args.length);
try (PrintStream out = new PrintStream(new FileOutputStream(outFile))) {
for (String arg : jars) {
extract(arg).forEach(out::println);
}
} catch (FileNotFoundException e) {
throw new RuntimeException(e);
}
}
/**
* Extracts names of all classes in given path.
*/
public static List extract(String path) {
return path.endsWith(JAR) ? extractJar(path) : extractDir(path);
}
private static List extractJar(String jarPath) {
File file = new File(jarPath);
try (JarFile jar = new JarFile(file)) {
System.out.printf("Scanning %s ... ", file.getAbsolutePath());
List classNames = jar.stream()
.filter(e -> !e.getName().startsWith("META-INF"))
.filter(e -> e.getName().endsWith(CLASS))
.map(e -> {
String name = e.getName();
return name.replaceAll("/", ".")
.substring(0, name.length() - CLASS.length());
})
.toList();
System.out.printf("%d classes%n", classNames.size());
return classNames;
} catch (IOException e) {
throw new RuntimeException("Failed to read jar file: " +
file.getAbsolutePath(), e);
}
}
private static List extractDir(String dirPath) {
Path dir = Path.of(dirPath);
if (!dir.toFile().isDirectory()) {
throw new RuntimeException(dir + " is not a directory");
}
try (Stream paths = Files.walk(dir)) {
System.out.printf("Scanning %s ... ", dir.toAbsolutePath());
List classNames = new ArrayList<>();
paths.map(dir::relativize).forEach(path -> {
String fileName = path.getFileName().toString();
int suffix;
if (fileName.endsWith(CLASS)) {
suffix = CLASS.length();
} else if (fileName.endsWith(JAVA)) {
suffix = JAVA.length();
} else {
return;
}
String name = String.join(".",
Lists.map(Lists.asList(path), Path::toString));
String className = name.substring(0, name.length() - suffix);
classNames.add(className);
});
System.out.printf("%d classes%n", classNames.size());
return classNames;
} catch (IOException e) {
throw new RuntimeException("Failed to read directory: " + dirPath, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy