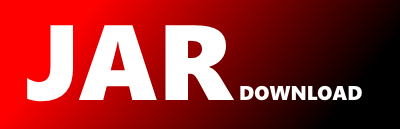
pascal.taie.util.collection.AbstractTwoKeyMultiMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tai-e Show documentation
Show all versions of tai-e Show documentation
An easy-to-learn/use static analysis framework for Java
The newest version!
/*
* Tai-e: A Static Analysis Framework for Java
*
* Copyright (C) 2022 Tian Tan
* Copyright (C) 2022 Yue Li
*
* This file is part of Tai-e.
*
* Tai-e is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3
* of the License, or (at your option) any later version.
*
* Tai-e is distributed in the hope that it will be useful,but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Tai-e. If not, see .
*/
package pascal.taie.util.collection;
import javax.annotation.Nonnull;
import java.io.Serializable;
import java.util.AbstractSet;
import java.util.Collections;
import java.util.Iterator;
import java.util.Set;
import java.util.StringJoiner;
public abstract class AbstractTwoKeyMultiMap implements
TwoKeyMultiMap, Serializable {
protected static final String NULL_KEY = "TwoKeyMultiMap does not permit null keys";
protected static final String NULL_VALUE = "TwoKeyMultiMap does not permit null values";
private transient Set> entrySet;
@Override
public Set> entrySet() {
var es = entrySet;
if (es == null) {
es = Collections.unmodifiableSet(new EntrySet());
entrySet = es;
}
return es;
}
private final class EntrySet extends AbstractSet> {
@Override
public boolean contains(Object o) {
if (o instanceof TwoKeyMap.Entry, ?, ?> entry) {
//noinspection unchecked
return AbstractTwoKeyMultiMap.this.contains(
(K1) entry.key1(), (K2) entry.key2(), (V) entry.value());
}
return false;
}
@Override
@Nonnull
public Iterator> iterator() {
return entryIterator();
}
@Override
public int size() {
return AbstractTwoKeyMultiMap.this.size();
}
}
protected abstract Iterator> entryIterator();
@Override
public boolean isEmpty() {
return size() == 0;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!(obj instanceof TwoKeyMultiMap)) {
return false;
}
@SuppressWarnings("unchecked")
TwoKeyMultiMap that = (TwoKeyMultiMap) obj;
if (size() != that.size()) {
return false;
}
try {
for (Pair twoKey : twoKeySet()) {
K1 key1 = twoKey.first();
K2 key2 = twoKey.second();
if (!get(key1, key2).equals(that.get(key1, key2))) {
return false;
}
}
} catch (ClassCastException | NullPointerException ignored) {
return false;
}
return true;
}
@Override
public String toString() {
StringJoiner joiner = new StringJoiner(", ", "{", "}");
for (Pair twoKey : twoKeySet()) {
K1 key1 = twoKey.first();
K2 key2 = twoKey.second();
joiner.add(key1 + "," + key2 + "=" + get(key1, key2));
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy