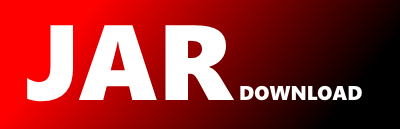
net.pechorina.kairos.automat.builder.StateConfigurer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kairos-automat Show documentation
Show all versions of kairos-automat Show documentation
Kairos Automat is a finite-state machine library
The newest version!
package net.pechorina.kairos.automat.builder
import net.pechorina.kairos.automat.Action
class StateConfigurer {
internal var states: MutableList> = arrayListOf()
private fun findState(state: S): StateConfig? {
return this.states.find { it.state == state }
}
fun initial(state: S): StateConfigurer {
val existingState = findState(state)
if (existingState != null) {
existingState.initialState = true
} else {
states.add(
StateConfig(state = state, initialState = true)
)
}
return this
}
fun initial(state: S, exitAction: Action?): StateConfigurer {
val existingState = findState(state)
existingState?.let {
it.initialState = true
it.exitAction = exitAction
}
if (existingState == null) {
states.add(
StateConfig(state = state, initialState = true, exitAction = exitAction)
)
}
return this
}
fun end(state: S): StateConfigurer {
val existingState = findState(state)
existingState?.let {
it.finalState = true
}
if (existingState == null) {
states.add(StateConfig(state = state, finalState = true))
}
return this
}
fun end(state: S, entryAction: Action?): StateConfigurer {
val existingState = findState(state)
existingState?.let {
it.finalState = true
it.entryAction = entryAction
}
if (existingState == null) {
states.add(StateConfig(state = state, finalState = true, entryAction = entryAction))
}
return this
}
fun state(state: S): StateConfigurer {
val existingState = findState(state)
if (existingState == null) {
this.states.add(StateConfig(state))
}
return this
}
fun state(state: S, parent: S?): StateConfigurer {
val existingState = findState(state)
existingState?.let {
it.parent = parent
}
if (existingState == null)
this.states.add(StateConfig(state = state, parent = parent))
return this
}
fun state(state: S, parent: S?, entryAction: Action?, exitAction: Action?): StateConfigurer {
val existingState = findState(state)
existingState?.let {
it.parent = parent
it.entryAction = entryAction
it.exitAction = exitAction
}
if (existingState == null)
this.states.add(
StateConfig(
state = state,
parent = parent,
entryAction = entryAction,
exitAction = exitAction)
)
return this
}
fun state(state: S, entryAction: Action?, exitAction: Action?): StateConfigurer {
val existingState = findState(state)
existingState?.let {
it.entryAction = entryAction
it.exitAction = exitAction
}
if (existingState == null)
this.states.add(
StateConfig(
state = state,
entryAction = entryAction,
exitAction = exitAction)
)
return this
}
fun state(
state: S,
parent: S?,
initialState: Boolean = true,
finalState: Boolean = true,
entryAction: Action?,
exitAction: Action?
): StateConfigurer {
val existingState = findState(state)
existingState?.let { stateConfig ->
entryAction?.let { stateConfig.entryAction = entryAction }
exitAction?.let { stateConfig.exitAction = exitAction }
parent?.let { stateConfig.parent = it }
existingState.initialState = initialState
existingState.finalState = finalState
}
if (existingState == null)
this.states.add(
StateConfig(
state = state,
parent = parent,
initialState = initialState,
finalState = finalState,
entryAction = entryAction,
exitAction = exitAction)
)
return this
}
fun stateEntry(state: S, entryAction: Action): StateConfigurer {
val existingState = findState(state)
existingState?.let {
it.entryAction = entryAction
}
if (existingState == null)
this.states.add(
StateConfig(
state = state,
entryAction = entryAction
)
)
return this
}
fun stateExit(state: S, exitAction: Action): StateConfigurer {
val existingState = findState(state)
existingState?.let {
it.exitAction = exitAction
}
if (existingState == null)
this.states.add(
StateConfig(
state = state,
exitAction = exitAction
)
)
return this
}
private fun stateExists(state: S): Boolean {
return findState(state) != null
}
fun states(states: Collection): StateConfigurer {
val statesToAdd = states.filterNot { stateExists(it) }
.map { StateConfig(state = it) }
this.states.addAll(statesToAdd)
return this
}
}