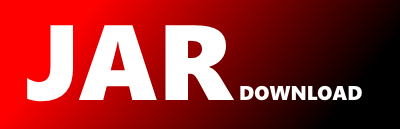
net.pincette.util.AsyncBuilder Maven / Gradle / Ivy
package net.pincette.util;
import static java.util.concurrent.CompletableFuture.completedFuture;
import static net.pincette.util.Util.tryToGetRethrow;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import net.pincette.function.FunctionWithException;
import net.pincette.function.SupplierWithException;
/**
* Chains asynchronous updates to mutable objects.
*
* @param the object type.
* @author Werner Donn\u00e9
* @since 1.9
*/
public class AsyncBuilder {
private final CompletionStage> object;
private AsyncBuilder(final CompletionStage> object) {
this.object = object;
}
public static AsyncBuilder create(final SupplierWithException supplier) {
return new AsyncBuilder<>(
tryToGetRethrow(supplier)
.map(Optional::of)
.map(CompletableFuture::completedFuture)
.orElse(null));
}
private static CompletionStage> next(
final CompletionStage> object,
final FunctionWithException>> set) {
return object.thenComposeAsync(
obj ->
obj.flatMap(o -> tryToGetRethrow(() -> set.apply(o)))
.orElseGet(() -> completedFuture(Optional.empty())));
}
public CompletionStage> build() {
return object;
}
/**
* Calls set
when previous updates have completed, only when the latter didn't return
* an empty result.
*
* @param set the function that updates the mutable object.
* @return A builder with the additional update completion stage.
*/
public AsyncBuilder update(final FunctionWithException>> set) {
return new AsyncBuilder<>(next(object, set));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy