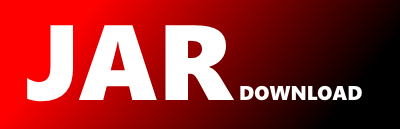
net.pincette.util.Pair Maven / Gradle / Ivy
package net.pincette.util;
import static java.util.Objects.hash;
import java.util.Objects;
import java.util.function.Function;
/**
* An immutable pair of elements.
*
* @author Werner Donn\u00e9
*/
public final class Pair {
public final T first;
public final U second;
public Pair(final T first, final U second) {
this.first = first;
this.second = second;
}
public static Pair pair(final T first, final U second) {
return new Pair<>(first, second);
}
/**
* Creates a pair with the first two elements. If the array has less than two elements the
* corresponding elements in the pair will be null
.
*
* @param array the given array.
* @param map the function that is applied to the array value and the result of which is put in
* the pair.
* @param the array element type.
* @param the pair element type.
* @return The new pair.
*/
public static Pair toPair(final T[] array, final Function map) {
return new Pair<>(
array.length > 0 ? map.apply(array[0]) : null,
array.length > 1 ? map.apply(array[1]) : null);
}
@SuppressWarnings("unchecked")
public boolean equals(final Object o) {
return o instanceof Pair
&& getClass().isAssignableFrom(o.getClass())
&& Objects.equals(first, ((Pair) o).first)
&& Objects.equals(second, ((Pair) o).second);
}
public int hashCode() {
return hash(first, second);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy