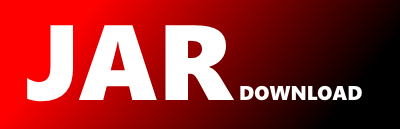
net.projectmonkey.Conditions Maven / Gradle / Ivy
/*
* Copyright 2011 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.projectmonkey;
import java.io.Serializable;
import net.projectmonkey.internal.util.Assert;
import net.projectmonkey.spi.MappingContext;
/**
* {@link Condition} utilities and implementations. This class can be extended by a PropertyMap to
* provide convenient access to methods.
*
* @author Jonathan Halterman
*/
public class Conditions {
private static final Condition, ?> IS_NULL = new AbstractCondition
© 2015 - 2025 Weber Informatics LLC | Privacy Policy