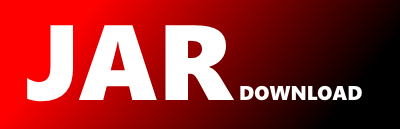
net.projectmonkey.internal.MappingContextImpl Maven / Gradle / Ivy
/*
* Copyright 2011 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.projectmonkey.internal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import net.projectmonkey.TypeMap;
import net.projectmonkey.Provider.ProvisionRequest;
import net.projectmonkey.internal.util.Assert;
import net.projectmonkey.internal.util.Types;
import net.projectmonkey.spi.Mapping;
import net.projectmonkey.spi.MappingContext;
import net.projectmonkey.spi.MappingEngine;
/**
* MappingContext implementation that caches destination values by their corresponding Mutator.
*
* @author Jonathan Halterman
*/
public class MappingContextImpl implements MappingContext, ProvisionRequest {
final Map destinationCache;
final Errors errors;
private final Set> currentlyMapping;
private D destination;
private final Class destinationType;
private Mapping mapping;
private final MappingEngine mappingEngine;
private final S source;
private Object parentSource;
private final Class sourceType;
private TypeMap typeMap;
/** Tracks destination hierarchy paths that were shaded by a condition */
private final List shadedPaths;
/**
* Create initial MappingContext.
*/
public MappingContextImpl(final S source, final Class sourceType, final D destination, final Class destinationType,
final MappingEngine mappingEngine) {
this.source = source;
this.sourceType = sourceType;
this.destination = destination;
this.destinationType = destinationType;
this.mappingEngine = mappingEngine;
currentlyMapping = new HashSet>();
errors = new Errors();
destinationCache = new HashMap();
shadedPaths = new ArrayList();
}
/**
* Create child MappingContext. The mapping is no longer mapped to S and D in this scope.
*/
MappingContextImpl(final MappingContextImpl, ?> context, final S source, final Class sourceType,
final D destination, final Class destinationType, final Mapping mapping) {
this.source = source;
this.sourceType = sourceType;
this.destination = destination;
this.destinationType = destinationType;
this.typeMap = null;
this.mapping = mapping;
parentSource = context.getParentSource();
mappingEngine = context.mappingEngine;
currentlyMapping = context.currentlyMapping;
errors = context.errors;
destinationCache = context.destinationCache;
shadedPaths = context.shadedPaths;
}
@Override
public MappingContext create(final CS source, final Class destinationType) {
Assert.notNull(source, "source");
Assert.notNull(destinationType, "destinationType");
return new MappingContextImpl(this, source, Types.deProxy(source.getClass()), null,
destinationType, mapping);
}
@Override
public boolean equals(final Object obj) {
if (this == obj)
return true;
if (obj == null || getClass() != obj.getClass())
return false;
MappingContextImpl, ?> other = (MappingContextImpl, ?>) obj;
if (!source.equals(other.source))
return false;
if (!sourceType.equals(other.sourceType))
return false;
if (!destinationType.equals(other.destinationType))
return false;
return true;
}
public void finishedMapping(final Class> type) {
currentlyMapping.remove(type);
}
@Override
public D getDestination() {
return destination;
}
@Override
public Class getDestinationType() {
return destinationType;
}
@Override
public Mapping getMapping() {
return mapping;
}
@Override
public MappingEngine getMappingEngine() {
return mappingEngine;
}
@Override
public Class getRequestedType() {
return destinationType;
}
@Override
public S getSource() {
return source;
}
@Override
public Class getSourceType() {
return sourceType;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + source.hashCode();
result = prime * result + sourceType.hashCode();
result = prime * result + destinationType.hashCode();
return result;
}
@Override
public String toString() {
return String.format("MappingContext[%s -> %s]", source, Types.toString(destinationType));
}
@Override
public TypeMap typeMap() {
return typeMap;
}
/**
* Marks {@code type} as currently being mapped.
*
* @param type to mark as being mapped
* @return boolean true if {@code type} is currently being mapped
*/
boolean currentlyMapping(final Class> type) {
return !currentlyMapping.add(type);
}
/**
* Determines whether the {@code subpath} is shaded.
*/
boolean isShaded(final String subpath) {
for (String shadedPath : shadedPaths)
if (subpath.startsWith(shadedPath))
return true;
return false;
}
void setDestination(final D destination) {
this.destination = destination;
}
void setTypeMap(final TypeMap typeMap) {
this.typeMap = typeMap;
}
/**
* Shades the {@code path} such that subsequent subpaths can be skipped during the mapping
* process.
*/
void shadePath(final String path) {
shadedPaths.add(path);
}
public Object getParentSource() {
return parentSource;
}
public void setParentSource(final Object parentSource) {
this.parentSource = parentSource;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy