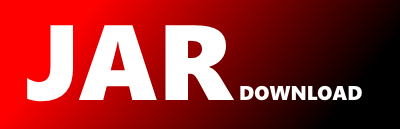
net.projectmonkey.object.mapper.ObjectMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of object-mapper Show documentation
Show all versions of object-mapper Show documentation
Object mapping implementation written as an alternative to modelmapper which is able to support inheritance, handles flattening / expanding in a precise way, and is extensible / configurable
The newest version!
package net.projectmonkey.object.mapper;
import net.projectmonkey.object.mapper.context.ConversionConfiguration;
import net.projectmonkey.object.mapper.context.ExecutionContext;
/*
*
* * Copyright 2012 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
/**
* @author Andy Moody
*/
public class ObjectMapper
{
private ObjectMappingService mappingService;
private ConversionConfiguration defaultConfiguration;
public ObjectMapper()
{
this(new ObjectMappingService(), new ConversionConfiguration());
}
public ObjectMapper(ConversionConfiguration conversionConfiguration)
{
this(new ObjectMappingService(), conversionConfiguration);
}
ObjectMapper(final ObjectMappingService mappingService)
{
this(mappingService, new ConversionConfiguration());
}
ObjectMapper(final ObjectMappingService mappingService, final ConversionConfiguration conversionConfiguration)
{
this.mappingService = mappingService;
this.defaultConfiguration = conversionConfiguration;
}
/**
* Map the source to the destination type.
*
* A new instance of destinationType will be created using the default constructor
* and matching fields will be populated as per the contents in the source object and the configured mappings.
*
* To customise the mapping process use {@link #map(Object, Class, net.projectmonkey.object.mapper.context.ConversionConfiguration)}
*
* @param source
* @param destinationType
* @return the constructed and populated destination
*/
public T map(final K source, final Class destinationType) {
return map(source, destinationType, defaultConfiguration);
}
/**
* Map the source to the destination type using the provided group .
*
* A new instance of destinationType will be created using the default constructor
* and matching fields will be populated as per the contents in source and the configured mappings.
*
* @param source
* @param destinationType
* @param group
* @return the constructed and populated destination
*/
public T map(final K source, final Class destinationType, final Class> group)
{
return map(source, destinationType, new ConversionConfiguration(defaultConfiguration).setGroup(group));
}
/**
* Map the source to the destination type using the provided {@link net.projectmonkey.object.mapper.context.ConversionConfiguration}
*
* A new instance of destinationType will be created using the default constructor
* and matching fields will be populated as per the contents in source and the configured mappings.
*
* @param source
* @param destinationType
* @param configuration
* @return the constructed and populated destination
*/
public T map(final K source, final Class destinationType, final ConversionConfiguration configuration)
{
T toReturn = null;
try
{
ExecutionContext.set(new ExecutionContext(configuration, mappingService));
toReturn = mappingService.map(source, destinationType);
}
finally
{
ExecutionContext.clear();
}
return toReturn;
}
/**
* Merge the contents of the source object into the destination object.
* Merging impacts all properties which have a mapping. The affected
* properties will be updated / overwritten with the source values as per the
* configured converters.
*
* New objects will only be created in the destination where they are
* currently null in the destination and non-null in the source object.
*
*
* To customise the merging process use {@link #merge(Object, Object, net.projectmonkey.object.mapper.context.ConversionConfiguration)}
*
* @param source
* @param destination
*/
public void merge(final K source, final T destination) {
merge(source, destination, defaultConfiguration);
}
/**
* Merge the contents of the source object into the destination object using the provided group
*
* @param source
* @param destination
* @param group
*/
public void merge(final K source, final T destination, final Class> group)
{
merge(source, destination, new ConversionConfiguration(defaultConfiguration).setGroup(group));
}
/**
* Merge the contents of the source object into the destination object using the provided {@link net.projectmonkey.object.mapper.context.ConversionConfiguration}
*
* @param source
* @param destination
* @param configuration
*/
public void merge(final K source, final T destination, final ConversionConfiguration configuration)
{
try
{
ExecutionContext.set(new ExecutionContext(configuration, mappingService));
mappingService.merge(source, destination);
}
finally
{
ExecutionContext.clear();
}
}
public ConversionConfiguration getDefaultConfiguration()
{
return defaultConfiguration;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy