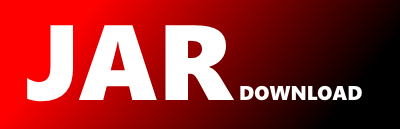
net.projectmonkey.object.mapper.analysis.resolver.FieldBasedPropertyResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of object-mapper Show documentation
Show all versions of object-mapper Show documentation
Object mapping implementation written as an alternative to modelmapper which is able to support inheritance, handles flattening / expanding in a precise way, and is extensible / configurable
The newest version!
package net.projectmonkey.object.mapper.analysis.resolver;
import net.projectmonkey.object.mapper.analysis.result.PropertyPath;
import net.projectmonkey.object.mapper.analysis.result.PropertyPathElement;
import net.projectmonkey.object.mapper.context.ExecutionContext;
import net.projectmonkey.object.mapper.util.Types;
import net.projectmonkey.object.mapper.analysis.result.FieldBasedPropertyPathElement;
import net.projectmonkey.object.mapper.util.ReflectionUtil;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.util.ArrayList;
import java.util.List;
/*
*
* * Copyright 2012 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
/**
* @author Andy Moody
*/
public final class FieldBasedPropertyResolver implements PropertyResolver
{
public static final FieldBasedPropertyResolver INSTANCE = new FieldBasedPropertyResolver();
private FieldBasedPropertyResolver(){}
@Override
public List resolvePropertyPaths(Class> clazz)
{
return getPropertyPaths(clazz, null);
}
private List getPropertyPaths(final Class> clazz, final PropertyPath parent)
{
List propertyPaths = ExecutionContext.getPaths(clazz);
if(propertyPaths == null)
{
propertyPaths = new ArrayList();
ExecutionContext.registerPaths(clazz, propertyPaths); //register as soon as we create the paths list.
if(!Types.isKnownType(clazz))
{
Field[] declaredFields = ReflectionUtil.getAllFields(clazz);
for(Field declaredField : declaredFields)
{
if(!Modifier.isStatic(declaredField.getModifiers()))
{
PropertyPath path = resolvePath(clazz, parent, declaredField);
propertyPaths.add(path);
}
}
}
}
return propertyPaths;
}
private PropertyPath resolvePath(final Class> clazz, final PropertyPath parent, final Field field)
{
PropertyPathElement property = new FieldBasedPropertyPathElement(clazz, field);
Class> fieldType = property.getType();
PropertyPath toReturn = new PropertyPath(parent, property);
List children = getPropertyPaths(fieldType, toReturn);
toReturn.setChildren(children);
return toReturn;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy