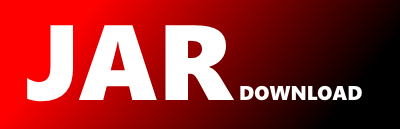
net.projectmonkey.object.mapper.analysis.result.PropertyPath Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of object-mapper Show documentation
Show all versions of object-mapper Show documentation
Object mapping implementation written as an alternative to modelmapper which is able to support inheritance, handles flattening / expanding in a precise way, and is extensible / configurable
The newest version!
package net.projectmonkey.object.mapper.analysis.result;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/*
*
* * Copyright 2012 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
/**
* @author Andy Moody
* Representation of the context of a property.
*/
public class PropertyPath
{
private final PropertyPath parent;
private final PropertyPathElement property;
private List children;
private final List completePath;
/**
* Constructor for properties on the root object - i.e. those without a parent
*/
public PropertyPath(final PropertyPathElement property)
{
this(null, property);
}
/**
* Constructor for properties which have a parent in the hierarchy
* @param parent
* @param property
*/
public PropertyPath(final PropertyPath parent, final PropertyPathElement property)
{
this.parent = parent;
this.property = property;
this.children = new ArrayList();
this.completePath = calculateCompletePath(parent);
}
public PropertyPathElement getProperty()
{
return property;
}
public List getChildren()
{
return Collections.unmodifiableList(children);
}
public void setChildren(final List children)
{
this.children = children;
}
public PropertyPath getParent()
{
return parent;
}
/**
* @return The complete path to a property from the object root.
* The first entry in the list represents a field in the root object
* and each subsequent entry represents a property in the preceeding
* object in the hierarchy.
*/
public List getCompletePath()
{
return completePath;
}
@Override
public boolean equals(final Object o)
{
if(this == o)
{
return true;
}
if(o == null || getClass() != o.getClass())
{
return false;
}
PropertyPath that = (PropertyPath) o;
if(parent != null ? !parent.equals(that.parent) : that.parent != null)
{
return false;
}
if(property != null ? !property.equals(that.property) : that.property != null)
{
return false;
}
return true;
}
@Override
public int hashCode()
{
int result = parent != null ? parent.hashCode() : 0;
result = 31 * result + (property != null ? property.hashCode() : 0);
return result;
}
@Override
public String toString()
{
StringBuilder builder = new StringBuilder("PropertyPath{");
builder.append("property=");
builder.append(property);
builder.append("}");
return builder.toString();
}
private List calculateCompletePath(final PropertyPath parent)
{
List toReturn = new ArrayList();
if(parent != null)
{
toReturn.addAll(parent.getCompletePath());
}
toReturn.add(property);
return toReturn;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy