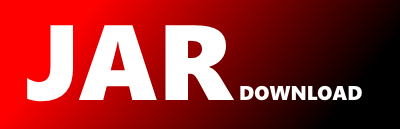
net.projectmonkey.object.mapper.analysis.result.PropertyPathElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of object-mapper Show documentation
Show all versions of object-mapper Show documentation
Object mapping implementation written as an alternative to modelmapper which is able to support inheritance, handles flattening / expanding in a precise way, and is extensible / configurable
The newest version!
package net.projectmonkey.object.mapper.analysis.result;
import net.projectmonkey.object.mapper.annotations.group.Group;
import net.projectmonkey.object.mapper.annotations.group.GroupBehaviour;
import net.projectmonkey.object.mapper.annotations.group.Groups;
import java.lang.reflect.AnnotatedElement;
import java.lang.reflect.Member;
import java.lang.reflect.Type;
import java.util.*;
/*
*
* * Copyright 2012 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
/**
* @author Andy Moody
*
* Represents a step in the path to a property from the root.
* Note that concrete implementations of this should define hashcode and equals
* based on the properties and classes they represent.
*/
public abstract class PropertyPathElement
{
private final String name;
private Map, PropertyConfiguration> configurationsByGroup;
public PropertyPathElement(final String name, final Map, PropertyConfiguration> configurationsByGroup)
{
this.name = name;
this.configurationsByGroup = configurationsByGroup;
}
public String getName()
{
return name;
}
public abstract Class> getType();
public abstract Type getGenericType();
public abstract Object getValue(final Object source);
public abstract void setValue(final Object value, final Object destination);
public PropertyConfiguration getConfigurationForGroup(Class> group)
{
return configurationsByGroup.get(group);
}
public boolean isIncludedInGroup(Class> group)
{
PropertyConfiguration propertyConfiguration = getConfigurationForGroup(group);
boolean included = false;
if(propertyConfiguration != null)
{
included = propertyConfiguration.isIncluded();
}
else if(group.isAnnotationPresent(GroupBehaviour.class))
{
included = group.getAnnotation(GroupBehaviour.class).inclusive();
}
return included;
}
protected static Map,PropertyConfiguration> calculateGroups(final Class> memberType, final M... members)
{
Map, PropertyConfiguration> toReturn = new HashMap, PropertyConfiguration>();
List groups = new ArrayList();
Set types = new LinkedHashSet();
for (M member : members)
{
types.add(member);
}
types.add(memberType);
for (AnnotatedElement type : types)
{
if(type.isAnnotationPresent(Groups.class))
{
groups.addAll(Arrays.asList(type.getAnnotation(Groups.class).value()));
}
if(type.isAnnotationPresent(Group.class))
{
groups.add(type.getAnnotation(Group.class));
}
}
for (Group group : groups)
{
PropertyConfiguration config = new PropertyConfiguration(group);
Class> groupClass = group.value();
if(toReturn.containsKey(groupClass))
{
toReturn.get(groupClass).merge(config);
}
else
{
toReturn.put(groupClass, config);
}
}
return toReturn;
}
@Override
public String toString()
{
return "PropertyPathElement{" +
"name='" + name + '\'' +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy