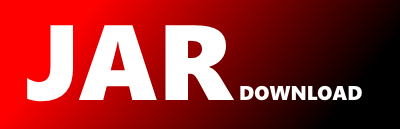
net.projectmonkey.object.mapper.construction.ConfigurationResolutionUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of object-mapper Show documentation
Show all versions of object-mapper Show documentation
Object mapping implementation written as an alternative to modelmapper which is able to support inheritance, handles flattening / expanding in a precise way, and is extensible / configurable
The newest version!
package net.projectmonkey.object.mapper.construction;
/*
*
* * Copyright 2012 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
import net.projectmonkey.object.mapper.context.ConversionConfiguration;
import net.projectmonkey.object.mapper.context.ExecutionContext;
import net.projectmonkey.object.mapper.analysis.result.PropertyConfiguration;
import net.projectmonkey.object.mapper.context.All;
import net.projectmonkey.object.mapper.util.ReflectionUtil;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
* @author Andy Moody
*/
public class ConfigurationResolutionUtil
{
public static final ConfigurationResolutionUtil INSTANCE = new ConfigurationResolutionUtil();
private ConfigurationResolutionUtil(){}
public T getFirstAppropriate(final PopulationContext, ?> context, ConfiguredTypeRetriever retriever)
{
ConversionConfiguration configuration = ExecutionContext.getConfiguration();
Class extends T> converterClass = resolveClass(context, configuration.getGroup(), retriever);
Class topLevelType = retriever.getTopLevelType();
if(converterClass == topLevelType)
{
converterClass = resolveClass(context, All.class, retriever);
}
T toReturn = null;
if(converterClass != topLevelType)
{
toReturn = ReflectionUtil.createOrRetrieve(converterClass);
if(!retriever.isApplicable(toReturn, context))
{
throw new IllegalStateException(toReturn+" is configured but is not applicable for context "+context);
}
}
else
{
List configuredItems = new ArrayList(retriever.getConfigured(configuration, context));
for(T item : configuredItems)
{
if(retriever.isApplicable(item, context))
{
toReturn = item;
break;
}
}
}
return toReturn;
}
private Class extends T> resolveClass(final PopulationContext, ?> context, final Class> group, ConfiguredTypeRetriever retriever)
{
List groupConfigurations = Arrays.asList(context.getSourceConfiguration(group), context.getDestinationConfiguration(group));
Class topLevelType = retriever.getTopLevelType();
Class extends T> currentClass = topLevelType;
for (PropertyConfiguration groupConfiguration : groupConfigurations)
{
if(groupConfiguration != null)
{
Class extends T> configuredClass = retriever.retrieveType(groupConfiguration);
if(currentClass != topLevelType && configuredClass != topLevelType && currentClass != configuredClass)
{
throw new IllegalStateException(currentClass+" and "+configuredClass+" are both configured for group " +group+" in context "+context);
}
currentClass = configuredClass;
}
}
return currentClass;
}
public static interface ConfiguredTypeRetriever
{
Class extends T> retrieveType(PropertyConfiguration configuration);
Class getTopLevelType();
List extends T> getConfigured(final ConversionConfiguration configuration, final PopulationContext, ?> context);
boolean isApplicable(T item, PopulationContext, ?> context);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy