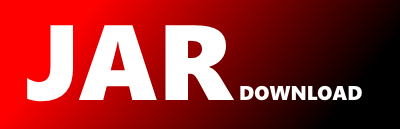
net.projectmonkey.object.mapper.construction.PropertyPopulationContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of object-mapper Show documentation
Show all versions of object-mapper Show documentation
Object mapping implementation written as an alternative to modelmapper which is able to support inheritance, handles flattening / expanding in a precise way, and is extensible / configurable
The newest version!
package net.projectmonkey.object.mapper.construction;
import net.projectmonkey.object.mapper.analysis.result.PropertyConfiguration;
import net.projectmonkey.object.mapper.analysis.result.PropertyPathElement;
import net.projectmonkey.object.mapper.construction.type.GenericTypeUtils;
import java.lang.reflect.Type;
import java.lang.reflect.TypeVariable;
import java.util.Map;
/*
*
* * Copyright 2012 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
/**
* @author Andy Moody
*/
public final class PropertyPopulationContext implements PopulationContext
{
private final PopulationContext parentContext;
private final PropertyPathElement destPath;
private final PropertyPathElement sourcePath;
private final Class destinationType;
private SourceType source;
private DestinationType destination;
private Map, Class>> destinationTypeArguments;
public PropertyPopulationContext(final PopulationContext parentContext, final PropertyPathElement destPath, final PropertyPathElement sourcePath, final SourceType source,
final Class destinationType)
{
this.parentContext = parentContext;
this.destPath = destPath;
this.sourcePath = sourcePath;
this.source = source;
this.destinationType = destinationType;
this.destinationTypeArguments = GenericTypeUtils.migrateDestinationTypeArguments(destPath, parentContext);
}
@Override
public SourceType getSource()
{
return source;
}
@Override
public Class getSourceType()
{
return (Class) sourcePath.getType();
}
@Override
public DestinationType getDestination()
{
return destination;
}
@Override
public Class getDestinationType()
{
return destinationType;
}
@Override
public Class> getParentSourceType()
{
return parentContext.getSourceType();
}
@Override
public Class> getParentDestinationType()
{
return parentContext.getDestinationType();
}
public Object getParentSource()
{
return parentContext.getSource();
}
public Object getParentDestination()
{
return parentContext.getDestination();
}
@Override
public void setDestination(final DestinationType destination)
{
this.destination = destination;
}
@Override
public Type getDestinationGenericType()
{
return destPath.getGenericType();
}
@Override
public PropertyConfiguration getSourceConfiguration(final Class> group)
{
return sourcePath.getConfigurationForGroup(group);
}
@Override
public PropertyConfiguration getDestinationConfiguration(final Class> group)
{
return destPath.getConfigurationForGroup(group);
}
@Override
public PropertyPathElement getDestinationProperty()
{
return destPath;
}
@Override
public void addDestinationTypeArguments(final Map, Class>> destinationTypesByVariable)
{
for (TypeVariable> typeVariable : destinationTypesByVariable.keySet())
{
if(!this.destinationTypeArguments.containsKey(typeVariable))
{
this.destinationTypeArguments.put(typeVariable, destinationTypesByVariable.get(typeVariable));
}
}
}
@Override
public Map, Class>> getDestinationTypeArguments()
{
return destinationTypeArguments;
}
@Override
public PropertyPathElement getSourceProperty()
{
return sourcePath;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy