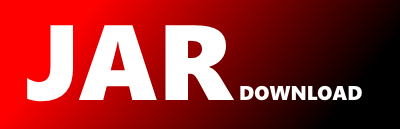
net.projectmonkey.object.mapper.construction.TestPopulationContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of object-mapper Show documentation
Show all versions of object-mapper Show documentation
Object mapping implementation written as an alternative to modelmapper which is able to support inheritance, handles flattening / expanding in a precise way, and is extensible / configurable
The newest version!
package net.projectmonkey.object.mapper.construction;
import net.projectmonkey.object.mapper.analysis.result.PropertyPathElement;
import net.projectmonkey.object.mapper.analysis.result.PropertyConfiguration;
import java.lang.reflect.Type;
import java.lang.reflect.TypeVariable;
import java.util.HashMap;
import java.util.Map;
/*
*
* * Copyright 2012 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
/**
* @author Andy Moody
*/
public class TestPopulationContext implements PopulationContext
{
private Type destinationGenericType;
private SourceType source;
private Class extends SourceType> sourceType;
private DestinationType destination;
private Class extends DestinationType> destinationType;
private Class> parentSourceType;
private Class> parentDestinationType;
private Map, PropertyConfiguration> sourceConfigurations = new HashMap, PropertyConfiguration>();
private Map, PropertyConfiguration> destinationConfigurations = new HashMap, PropertyConfiguration>();
private PropertyPathElement sourceProperty;
private PropertyPathElement destinationProperty;
private Object parentSource;
private Object parentDestination;
private Map, Class>> destinationTypeArguments = new HashMap, Class>>();
@Override
public SourceType getSource()
{
return source;
}
@Override
public Class extends SourceType> getSourceType()
{
return sourceType;
}
@Override
public DestinationType getDestination()
{
return destination;
}
@Override
public Class extends DestinationType> getDestinationType()
{
return destinationType;
}
@Override
public Class> getParentSourceType()
{
return parentSourceType;
}
@Override
public Class> getParentDestinationType()
{
return parentDestinationType;
}
@Override
public Object getParentDestination()
{
return parentDestination;
}
@Override
public Object getParentSource()
{
return parentSource;
}
@Override
public void setDestination(final DestinationType destination)
{
this.destination = destination;
}
@Override
public Type getDestinationGenericType()
{
return destinationGenericType;
}
@Override
public PropertyConfiguration getSourceConfiguration(final Class> group)
{
return sourceConfigurations.get(group);
}
@Override
public PropertyConfiguration getDestinationConfiguration(final Class> group)
{
return destinationConfigurations.get(group);
}
@Override
public PropertyPathElement getSourceProperty()
{
return sourceProperty;
}
@Override
public PropertyPathElement getDestinationProperty()
{
return destinationProperty;
}
@Override
public void addDestinationTypeArguments(final Map, Class>> destinationTypesByVariable)
{
this.destinationTypeArguments.putAll(destinationTypesByVariable);
}
@Override
public Map, Class>> getDestinationTypeArguments()
{
return destinationTypeArguments;
}
public TestPopulationContext setDestinationGenericType(Type destinationGenericType)
{
this.destinationGenericType = destinationGenericType;
return this;
}
public TestPopulationContext setSource(final SourceType source)
{
this.source = source;
return this;
}
public TestPopulationContext setSourceType(final Class extends SourceType> sourceType)
{
this.sourceType = sourceType;
return this;
}
public TestPopulationContext setDestinationType(final Class extends DestinationType> destinationType)
{
this.destinationType = destinationType;
return this;
}
public TestPopulationContext setParentSourceType(final Class> parentSourceType)
{
this.parentSourceType = parentSourceType;
return this;
}
public TestPopulationContext setParentDestinationType(final Class> parentDestinationType)
{
this.parentDestinationType = parentDestinationType;
return this;
}
public TestPopulationContext setDestinationObject(DestinationType destination)
{
this.destination = destination;
return this;
}
public TestPopulationContext addSourceConfiguration(Class> group, PropertyConfiguration configuration)
{
sourceConfigurations.put(group, configuration);
return this;
}
public TestPopulationContext addDestinationConfiguration(Class> group, PropertyConfiguration configuration)
{
destinationConfigurations.put(group, configuration);
return this;
}
public TestPopulationContext setSourceProperty(PropertyPathElement sourceProperty)
{
this.sourceProperty = sourceProperty;
return this;
}
public TestPopulationContext setDestinationProperty(PropertyPathElement destinationProperty)
{
this.destinationProperty = destinationProperty;
return this;
}
public TestPopulationContext setParentDestination(Object parentDestination)
{
this.parentDestination = parentDestination;
return this;
}
public TestPopulationContext setParentSource(Object parentSource)
{
this.parentSource = parentSource;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy