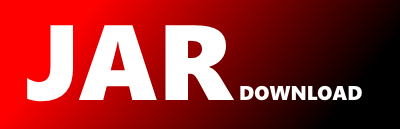
net.projectmonkey.object.mapper.construction.converter.IterableConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of object-mapper Show documentation
Show all versions of object-mapper Show documentation
Object mapping implementation written as an alternative to modelmapper which is able to support inheritance, handles flattening / expanding in a precise way, and is extensible / configurable
The newest version!
package net.projectmonkey.object.mapper.construction.converter;
import net.projectmonkey.object.mapper.ObjectMappingService;
import net.projectmonkey.object.mapper.construction.ElementPopulationContext;
import net.projectmonkey.object.mapper.construction.PopulationContext;
import net.projectmonkey.object.mapper.context.ExecutionContext;
import java.lang.reflect.Array;
import java.util.Collection;
import java.util.Iterator;
import static net.projectmonkey.object.mapper.construction.type.GenericTypeUtils.TypeAndClass;
/*
*
* * Copyright 2012 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
/**
* Support class for converting Iterable and psuedo-Iterable (array) instances. Implementors must
* support source and destination types that are Iterable or array instances.
*
* Adapted from ModelMapper (modelmapper.org)
*
* @author Jonathan Halterman
* @author Andy Moody
*/
abstract class IterableConverter implements Converter
{
@Override
public DestinationType convert(final PopulationContext context)
{
SourceType source = context.getSource();
Class destinationType = (Class) context.getDestinationType();
int sourceLength = getSourceLength(source);
TypeAndClass elementType = getElementType(destinationType, context);
DestinationType destination = createDestination(destinationType, sourceLength, elementType);
int index = 0;
for (Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy