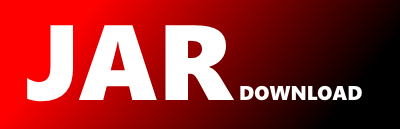
net.projectmonkey.object.mapper.construction.rule.resolver.StandardRuleResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of object-mapper Show documentation
Show all versions of object-mapper Show documentation
Object mapping implementation written as an alternative to modelmapper which is able to support inheritance, handles flattening / expanding in a precise way, and is extensible / configurable
The newest version!
package net.projectmonkey.object.mapper.construction.rule.resolver;
/*
*
* * Copyright 2012 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
import net.projectmonkey.object.mapper.construction.PopulationContext;
import net.projectmonkey.object.mapper.context.ExecutionContext;
import net.projectmonkey.object.mapper.analysis.result.PropertyConfiguration;
import net.projectmonkey.object.mapper.construction.rule.MappingRule;
import net.projectmonkey.object.mapper.construction.rule.PropertyGroupRule;
import net.projectmonkey.object.mapper.context.All;
import net.projectmonkey.object.mapper.util.ReflectionUtil;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
* The base MappingRuleResolver.
* Resolves rules by:
*
* Returning the rules configured for the group by the {@link net.projectmonkey.object.mapper.annotations.group.Group}
* annotations if there is precisely one. Returning all configured rules for the current
* group.
*
* If no rules are configured for the current group then any rules configured for the {@link net.projectmonkey.object.mapper.context.All} group will be returned.
*
* If there are no configured rules for the specific group or the {@link net.projectmonkey.object.mapper.context.All} group then
* the {@link #defaultRules} and {@link net.projectmonkey.object.mapper.context.ConversionConfiguration#customRules} lists
* to find all appropriate rules.
*
* @author Andy Moody
*/
public class StandardRuleResolver implements MappingRuleResolver
{
public static final StandardRuleResolver INSTANCE = new StandardRuleResolver();
private static final List> defaultRules;
static
{
defaultRules = Arrays.>asList(
PropertyGroupRule.INSTANCE
);
}
private StandardRuleResolver(){}
@Override
public List> getAllApplicableRules(final PopulationContext context)
{
List> toReturn = new ArrayList>();
Class> group = ExecutionContext.getConfiguration().getGroup();
List> rulesForGroup = resolveRuleClasses(context, group);
if(rulesForGroup.isEmpty())
{
rulesForGroup.addAll(resolveRuleClasses(context, All.class));
}
if(rulesForGroup.isEmpty())
{
List> customRules = ExecutionContext.getConfiguration().getCustomRules();
toReturn.addAll(filterApplicableRules(customRules, context));
toReturn.addAll(filterApplicableRules(defaultRules, context));
}
else
{
for (Class extends MappingRule> ruleClass : rulesForGroup)
{
MappingRule rule = ReflectionUtil.createOrRetrieve(ruleClass);
if(!rule.isApplicableFor(context))
{
throw new IllegalStateException("Configured rule "+ruleClass+" is not applicable for context "+context);
}
toReturn.add(rule);
}
}
return toReturn;
}
private List> filterApplicableRules(List> rules, final PopulationContext context)
{
List> toReturn = new ArrayList>();
for(MappingRule,?> rule : rules)
{
if(rule.isApplicableFor(context))
{
toReturn.add((MappingRule) rule);
}
}
return toReturn;
}
private List> resolveRuleClasses(final PopulationContext, ?> context, final Class> group)
{
List groupConfigurations = Arrays.asList(context.getSourceConfiguration(group),
context.getDestinationConfiguration(group));
List> toReturn = new ArrayList>();
for (PropertyConfiguration groupConfiguration : groupConfigurations)
{
if(groupConfiguration != null)
{
Class extends MappingRule>[] configuredRules = groupConfiguration.getRules();
if(configuredRules != null)
{
for (Class extends MappingRule> configuredRule : configuredRules)
{
if(configuredRule != null && configuredRule != MappingRule.class)
{
toReturn.add(configuredRule);
}
}
}
}
}
return toReturn;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy