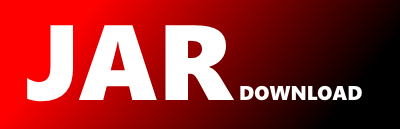
net.projectmonkey.object.mapper.util.CollectionUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of object-mapper Show documentation
Show all versions of object-mapper Show documentation
Object mapping implementation written as an alternative to modelmapper which is able to support inheritance, handles flattening / expanding in a precise way, and is extensible / configurable
The newest version!
package net.projectmonkey.object.mapper.util;
import java.util.*;
/*
*
* * Copyright 2012 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
/**
* @author Andy Moody
*/
public class CollectionUtil
{
public static boolean hasElements(final Collection> items)
{
return items != null && !items.isEmpty();
}
public static T[] addTo(T[] array, T...additionalValues)
{
List toReturn = createListWithAllElements(array, additionalValues);
return toReturn.toArray(array);
}
public static T[] addToWithoutDuplicates(T[] array, T...additionalValues)
{
List toReturn = createListWithAllElements(array, additionalValues);
Set linkedHashSet = new LinkedHashSet(toReturn);
return linkedHashSet.toArray(array);
}
private static List createListWithAllElements(final T[] array, final T[] additionalValues)
{
if(array == null)
{
throw new IllegalArgumentException("Cannot add to a null array");
}
List toReturn = new ArrayList(Arrays.asList(array));
if(additionalValues != null)
{
toReturn.addAll(Arrays.asList(additionalValues));
}
return toReturn;
}
public static void replace(List items, int position, T newObject)
{
items.add(position, newObject);
items.remove(position + 1);
}
public static int countInstancesOf(final T object, final List items, final int startingPosition)
{
int count = 0;
for(int i = startingPosition; i < items.size(); i++)
{
T item = items.get(i);
if(nullSafeEquals(object, item))
{
count ++;
}
}
return count;
}
public static int countInstancesOf(final T object, final List items)
{
return countInstancesOf(object, items, 0);
}
/**
* @param item
* @param items
* @param n
* @param
* @return the nth last index of the specified item, or -1 if there are not enough matching items.
*/
public static int nthLastIndexOf(final T item, final List items, int n)
{
int itemCount = 0;
for(int i = items.size() -1; i >= 0; i--)
{
if(nullSafeEquals(item, items.get(i)))
{
itemCount ++;
}
if(itemCount == n)
{
return i;
}
}
return -1;
}
/**
*
* @param collections
* @return a new list containing the constituent elements of all the provided collections.
*/
public static List combine(Collection... collections)
{
List toReturn = new ArrayList();
for (Collection collection : collections)
{
toReturn.addAll(collection);
}
return toReturn;
}
public static List combineLists(List> collections)
{
List toReturn = new ArrayList();
for (Collection collection : collections)
{
toReturn.addAll(collection);
}
return toReturn;
}
private static boolean nullSafeEquals(final T object, final T item)
{
boolean isItem = false;
if(object == null)
{
if(item == null)
{
isItem = true;
}
}
else if(object.equals(item))
{
isItem = true;
}
return isItem;
}
public static boolean startsWith(final List items, final List startingWith)
{
boolean toReturn = true;
if(hasElements(startingWith) && hasElements(items))
{
if(items.size() < startingWith.size())
{
toReturn = false;
}
else
{
for (int i = 0; i < startingWith.size(); i++)
{
if(!items.get(i).equals(startingWith.get(i)))
{
toReturn = false;
}
}
}
}
return toReturn;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy