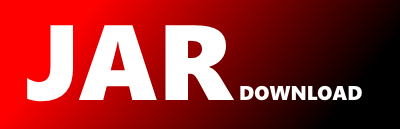
net.quasardb.qdb.ts.Result Maven / Gradle / Ivy
Go to download
API for the JNI components of the QuasarDB API for Java. Should not be included directly.
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy