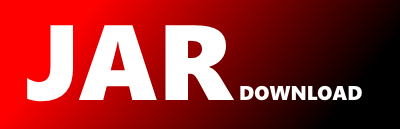
net.quasardb.qdb.ts.Writer Maven / Gradle / Ivy
Go to download
API for the JNI components of the QuasarDB API for Java. Should not be included directly.
The newest version!
Please wait ...