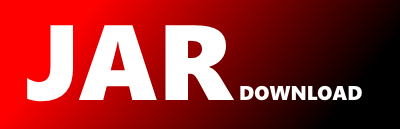
api.types.api Maven / Gradle / Ivy
[Contains information associated with an error captured by the Rapture system.]
@Bean
type ErrorWrapper(@package=rapture.common) {
String id;
Integer status;
String message;
String stackTrace;
}
[A return value from a native query.]
type RaptureQueryResult(@package=rapture.common) {
List rows;
}
[Defines a connection info]
type ConnectionInfo(@package=rapture.common) {
String host;
int port;
String username;
String password;
String dbName;
String instanceName;
Map(String, Object) options;
}
[Defines a BlobRepository.]
@Addressable(scheme = BLOB(true))
@Storable(storagePath : {authority}, prefix="blobConf")
type BlobRepoConfig(@package=rapture.common.model) {
String description;
String config;
String authority;
String metaConfig;
}
[ A RaptureField is the definition of a concept in Rapture, referenced within a type or a series of types.]
@Addressable(scheme = FIELD(false))
@Storable(storagePath : {authority, name}, prefix="field")
type RaptureField(@package=rapture.common) {
String authority;
String category;
String name;
String longName;
String description;
String units;
RaptureGroupingFn groupingFn = RaptureGroupingFn.SUM;
List bands = new ArrayList();
Set fieldPaths = new HashSet();
}
[Defines an audit result to be logged.]
type AuditLogEntry(@package=rapture.common.model) {
String category;
String entryId;
int level;
String logId;
String message;
String source;
String user;
Date when;
}
[]
@Storable(storagePath : { serverName }, prefix="audit/logj/config")
type Log4jAuditConfig(@package=rapture.common.model.audit) {
String serverName;
String logDirPath;
String xml;
}
[Stores the config information for an audit log.]
@Addressable(scheme = LOG(false))
@Storable(storagePath : {name}, prefix="audit/config")
type AuditLogConfig(@package=rapture.common) {
String name;
String config;
}
[This is a write handle returned after a write (update, delete, insert) operation to a document. It contains information about the document]
@Bean
type DocWriteHandle(@package=rapture.common.model) {
Boolean isSuccess;
RaptureURI documentURI;
RunEventHandle eventHandle; //if there was an event created associated with this
}
[Defines a script used to run a Rapture job.]
@Addressable(scheme = SCRIPT(false))
@Storable(storagePath : {authority, name}, prefix="script")
@Cacheable
type RaptureScript (@package=rapture.common) {
String name;
String script;
RaptureScriptLanguage language;
RaptureScriptPurpose purpose;
String authority;
List(RaptureParameter) parameters;
}
[Defines a portion of a Rapture script.]
@Addressable(scheme = SNIPPET(false))
@Storable(storagePath : {authority, name}, prefix="snippet")
@Cacheable
type RaptureSnippet (@package=rapture.common) {
String name;
String authority;
String snippet;
}
[Defines a variable used in a read-eval-print loop. ]
@Bean
type REPLVariable(@package=rapture.common) {
String name;
String serializedVar;
}
[Describes a read-eval-print loop in Reflex.]
@Storable(storagePath : { id }, prefix="reflex/repl", repoName=EPHEMERAL_REPO)
@Bean
type ReflexREPLSession(@package=rapture.common) {
String id;
List(REPLVariable) vars;
String partialLine = "";
List(String) functionDecls = null;
Date lastSeen;
}
[One of the scripting languages compatible with Rapture.]
type RaptureScriptLanguage (@package=rapture.common) {
String REFLEX;
}
[Describes the script's functionality.]
type RaptureScriptPurpose (@package=rapture.common) {
String INDEXGENERATOR;
String MAP;
String FILTER;
String OPERATION;
String PROGRAM;
String LINK;
}
[Metadata used by objects in the Lock API.]
@Addressable(scheme = LOCK(false))
@Storable(storagePath : {authority, name}, prefix="lock/config")
type RaptureLockConfig(@package=rapture.common) {
String name;
String config;
String authority;
String pathPosition;
}
[Handle to a Rapture lock.]
@Bean
type LockHandle(@package=rapture.common) {
String lockName;
String handle;
String lockHolder;
}
[Controls access to a resource using the standard semaphore model in programming.]
@Storable(storagePath: {lockKey}, ttlDays=7, repoName=EPHEMERAL_REPO)
type SemaphoreLock(@package=rapture.common) {
String lockKey;
Set stakeholderURIs = new HashSet();
}
[An object returned by public API calls that create things. If the object was created, the URI is
returned. Otherwise, the message contains the reason the object was not created.]
@Bean
type CreateResponse(@package=rapture.common) {
Boolean isCreated;
RaptureURI uri;
String message;
}
[A response returned when trying to acquire a semaphore lock. It indicates whether the lock
was acquired and identifies any existing stakeholders.]
@Bean
type SemaphoreAcquireResponse(@package=rapture.common) {
Boolean isAcquired;
RaptureURI acquiredURI;
Set existingStakeholderURIs = new HashSet();
}
[Holds the config info for a idgen as described in the idgen API.]
@Addressable(scheme = IDGEN(false))
@Storable(storagePath : {authority, name}, prefix="idgen")
type RaptureIdGenConfig(@package=rapture.common) {
String name;
String config;
String authority;
}
[Holds the config info for a Rapture table, as described in the table API.]
@Addressable(scheme = TABLE(false))
@Storable(storagePath : {authority, name}, prefix="indexOld")
type RaptureTableConfig(@package=rapture.common) {
String name;
String config;
String authority;
}
[Searches performed by any API calls are stored in this type.]
type RaptureSearchResult(@package=rapture.common) {
String displayName;
}
[Holds the config info for a repository.]
@Storable(storagePath : {"sys", name}, separator=".", prefix="repo/config", repoName=BOOTSTRAP_REPO)
type RepoConfig (@package=rapture.common.model) {
String name;
String config;
}
[Identifies the user making an API call. The metadata map allows API clients to associate values with a particular session (such as user preferences or client location).]
@Storable(storagePath : {context}, prefix="session", repoName=EPHEMERAL_REPO)
type CallingContext(@package=rapture.common) {
String user;
String context;
String salt;
Map metadata;
Boolean valid = false;
}
[Holds the details of a particular context.]
@Storable(storagePath : {sessionId}, prefix="context")
type RaptureContextInfo(@package=rapture.common) {
String sessionId;
String authority;
String perspective;
}
[Identifies the current authority.]
@Addressable(scheme = AUTHORITY(false))
type RaptureAuthority(@package=rapture.common) {
}
[Describes config info for a repository that stores Rapture documents.]
@Addressable(scheme = DOCUMENT(true))
@Storable(storagePath : {authority}, prefix="type")
type DocumentRepoConfig(@package=rapture.common.model) {
String description;
String config;
String authority;
IdGenURI idGenUri;
Boolean strictCheck = true;
Set indexes = new HashSet();
Set fullTextIndexes = new HashSet();
String updateQueue;
RaptureDocConfig documentRepo;
}
[Describes config info for a single Rapture document.]
@Storable(storagePath : {authority}, prefix="docConf")
type RaptureDocConfig(@package=rapture.common.model) {
String authority;
String config;
}
[Defines a user account for the Rapture system.]
@Storable(storagePath : {username}, prefix="sys.user", repoName=SETTINGS_REPO)
@Addressable(scheme = USER)
@Cacheable
type RaptureUser(@package=rapture.common.model) {
String username; //deprecated
String userId;
String emailAddress;
String salt = "";
String hashPassword;
String description = "";
Boolean inactive = false;
Boolean apiKey = false;
Boolean hasRoot = false;
String passwordResetToken;
Long tokenExpirationTime;
String registrationToken;
Boolean verified = true;
}
[The base object used by the entitlements API.]
@Addressable(scheme = ENTITLEMENT(true))
@Storable(storagePath : {name}, prefix="entitlement")
@Cacheable(shouldCacheNulls=true)
type RaptureEntitlement(@package=rapture.common.model) {
String name;
EntitlementType entType;
Set groups;
}
[A named collection of users who share any entitlements assigned to the group, as long as they remain members of the group.]
@Addressable(scheme = ENTITLEMENTGROUP(true))
@Storable(storagePath : {name}, prefix="entitlementgroup")
@Cacheable
type RaptureEntitlementGroup(@package=rapture.common.model) {
String name;
Set users;
String dynamicEntitlementClassName;
}
[Describes the main object used by the Event API.]
@Addressable(scheme = EVENT(false))
@Storable(storagePath : {uriFullPath}, prefix="event")
@Cacheable
type RaptureEvent(@package=rapture.common.model) {
String uriFullPath;
Set(RaptureEventScript) scripts;
Set(RaptureEventMessage) messages;
Set(RaptureEventNotification) notifications;
Set(RaptureEventWorkflow) workflows;
}
[This is a write handle returned after an event is executed.]
@Bean
type RunEventHandle(@package=rapture.common.model) {
RaptureURI eventUri;
String eventId;
Boolean didRun;
}
[Holds information about a server that is running Rapture.]
@Addressable(scheme = SERVER(false))
@Storable(storagePath : {serverId}, prefix="environment/server")
type RaptureServerInfo(@package=rapture.common.model) {
String serverId;
String name;
}
[Holds information about the status of a server that is running Rapture.]
@Addressable(scheme = SERVERSTATUS(false))
@Storable(storagePath : {serverId}, prefix="environment/status", repoName=EPHEMERAL_REPO)
type RaptureServerStatus(@package=rapture.common.model) {
String serverId;
Long status;
String statusMessage;
Date lastSeen = new Date();
}
[Holds config information for the main object used by the Plugin API.]
@Addressable(scheme = PLUGIN(false))
@Storable(storagePath : {plugin}, prefix="plugin/config")
type PluginConfig(@package=rapture.common) {
Map(String, PluginVersion) depends;
String description;
String plugin;
PluginVersion version;
}
[This is a more detailed variant of PluginConfig that details the complete contents of a version of a plugin instance.]
@Addressable(scheme = PLUGIN_MANIFEST(false))
@Storable(storagePath : {plugin}, prefix="plugin/manifest")
type PluginManifest(@package=rapture.common) {
List(PluginManifestItem) contents;
Map(String, PluginVersion) depends;
String description;
String plugin;
PluginVersion version;
}
[Contains a single entry in the contents of a Plugin manifest.]
@Addressable(scheme = PLUGIN_MANIFEST(false))
type PluginManifestItem(@package=rapture.common) {
String uri;
String hash;
}
[The PluginTransportItem is an internal class used by the PluginInstaller. It carries the encoded form of a Rapture object between the PluginInstaller and the Rapture server.]
@Bean
type PluginTransportItem(@package=rapture.common) {
String uri;
ByteArray content;
String hash;
}
[Holds version information for a Rapture plugin.]
type PluginVersion(@package=rapture.common) {
int major;
int minor;
int release;
long timestamp;
}
[Holds information about a stored JAR.]
@Addressable(scheme = JAR(false))
@Storable(storagePath : {authority}, prefix="jar", repoName=JAR_REPO)
type Jar(@package=rapture.common) {
String description;
String authority;
}
[Describes a repository for apps.]
@Storable(storagePath : {"settings"}, prefix="app/reposettings")
type AppRepoSettings(@package=rapture.common) {
String codeBase;
String raptureBase;
}
[Contains configuration details for hosted apps.]
@Storable(storagePath : {name}, prefix="app/config")
type AppConfig(@package=rapture.common) {
String name;
String title;
String vendor;
String homePage;
String longDescription;
String shortDescription;
String iconLocation;
String splashLocation;
String jarFile;
String mainClass;
}
[Contains information about an active instance of a hosted app.]
@Storable(storagePath : {appConfig, name}, prefix="app/instance")
type AppInstanceConfig(@package=rapture.common) {
String name;
String appConfig;
List(String) arguments;
Map(String, String) properties;
String apiKey;
}
[A RaptureExchange is the coordination point for a task based pipeline.
Clients put RapturePipelineTask instances onto an exchange, which then routes
that task to a set of queues that are then consumed.
This class defines the config of an exchange ]
@Storable(storagePath : {name}, prefix="exchange")
type RaptureExchange(@package=rapture.common.model) {
String domain;
String name;
RaptureExchangeType exchangeType;
List(RaptureExchangeQueue) queueBindings;
}
[Represents a task that has been submitted to the Rapture pipeline. Includes the task's status, type, and categories associated with it.]
type RapturePipelineTask(@package=rapture.common) {
PipelineTaskStatus status;
PipelineTaskType taskType;
int priority;
List categoryList;
String taskId;
String content;
String contentType;
Long epoch;
}
[Associates a server category with a comment.]
@Storable(storagePath : {name}, prefix="servercategory")
type ServerCategory(@package=rapture.common) {
String name;
String description;
}
[Describes bound queues in a task exchange pipeline.]
@Storable(storagePath : {name}, prefix="categorybinding")
type CategoryQueueBindings(@package=rapture.common) {
String name;
Map(String, Set(String)) bindings;
}
[Contains configuration details for a pipeline exchange.]
@Addressable(scheme = EXCHANGE_DOMAIN(false))
@Storable(storagePath: {name}, prefix="exchangeDomain")
type ExchangeDomain(@package=rapture.common) {
String name;
String config;
}
[Describes a table object.]
type TableRecord(@package=rapture.common) {
String keyName;
Map(String, Object) fields;
String content;
}
[Describes a query made against a table.]
type TableQuery(@package=rapture.common) {
List(TableSelect) fieldTests;
List(String) fieldReturns;
List(TableColumnSort) sortFields;
int skip;
int limit;
}
[Contains the metadata, if any, in a Rapture document.]
@Bean
type DocumentMetadata(@package=rapture.common.model) {
Integer version;
Date writeTime; //deprecated, replaced by modifiedTimestamp
Long createdTimestamp;
Long modifiedTimestamp;
String user;
String comment;
Boolean deleted;
}
[Contains a Rapture document and its metadata.]
@Bean
type DocumentWithMeta(@package=rapture.common.model) {
String displayName;
DocumentMetadata metaData;
String content;
}
[Holds a key/value pair for an attribute of a document object.]
type XferDocumentAttribute(@package=rapture.common) {
String attributeType;
String key;
String value;
}
[Contains details about the current tasks on a pipeline.]
type PipelineTaskStatus(@package=rapture.common) {
PipelineTaskState currentState;
String taskId;
String relatedTaskId;
Date creationTime;
Date startExecutionTime;
Date endExecutionTime;
int suspensionCount;
List(String) output;
}
[Contains info for a script-driven job in Rapture.]
@Addressable(scheme = JOB(false))
@Storable(storagePath : {jobURI}, prefix="versioned/schedule/job/v2")
type RaptureJob(@package=rapture.common) {
JobURI jobURI;
String description;
ScriptURI scriptURI;
String cronSpec;
String timeZone = "America/New_York";
Map params;
Boolean autoActivate = true;
Boolean activated = true;
JobType jobType;
Integer maxRuntimeMinutes = -1;
String appStatusNamePattern; //appstatus name pattern, will be interpreted and passed in to workflow
}
[Contains info about an active or queued job.]
@Storable(storagePath : {jobURI, execTime}, ttlDays=180, prefix="versioned/schedule/exec/default/v2")
type RaptureJobExec(@package=rapture.common) {
JobURI jobURI;
JobType jobType;
JobExecStatus status = JobExecStatus.WAITING;
Long execTime;
Map passedParams = new HashMap();
String execDetails = ""; //stores additional details, to be interpreted based on jobType
}
[Represents the workorderId, which is stored in a RaptureJobExec for a workflow-based job.]
@Bean
type WorkflowJobDetails(@package=rapture.common) {
String workOrderURI;
}
[Contains a copy of the upcoming JobExec for a RaptureJob.
It is used by the ScheduleManager to determine whether something needs to run.
This way the ScheduleManager doesn't have to inefficiently sift through all jobs to make this decision.]
@Storable(storagePath : {jobURI}, prefix="versioned/schedule/exec/upcoming/v2")
@Extends(rapture.common RaptureJobExec)
type UpcomingJobExec(@package=rapture.common) {
}
[Contains a copy of the previous JobExec for a RaptureJob.
It is used to retrieve statuses of jobs that were previously executed, so that
the ScheduleManager doesn't have to inefficiently sift through all jobs to determine statuses.]
@Storable(storagePath : {jobURI}, ttlDays=180, prefix="versioned/schedule/exec/last/v2")
@Extends(rapture.common RaptureJobExec)
type LastJobExec(@package=rapture.common) {
}
[The status for an individual workflow-based job execution -- either upcoming or in the past.]
@Bean
type WorkflowJobExecDetails(@package=rapture.common) {
JobURI jobURI;
Map parameters;
Map passedParams = new HashMap(); //optional override
WorkflowURI workflowURI;
WorkOrderURI workOrderURI;
String workOrderID; //a shorter version of workOrderURI -- just the id at the end, useful for display
Long startDate;
Long lastUpdated;
WorkOrderExecutionState workOrderStatus;
JobExecStatus jobStatus;
String prettyStatus; //a human-readable status, aggregating jobStatus and workOrderStatus
Long overrunMillis = 0L; //how much we have overrun, or 0 if not overrun yet
Integer maxRuntimeMinutes = -1;
String notes; // optional notes about this workflow's status (e.g. "Still running")
JobErrorAck errorAck; // set if this is in error state and someone acknowledged the error
}
[Acknowledgement of a job failure or delay.]
@Storable(storagePath : {jobURI, execTime}, repoName=EPHEMERAL_REPO)
type JobErrorAck(@package=rapture.common) {
JobURI jobURI;
Long execTime;
JobErrorType errorType; // the type of error that was acknowledged
Long timestamp; // when this was acknowledged
String user; // who acknowledged this
}
[The status for a workflow-based job that's already completed.]
@Bean
type WorkflowExecsStatus(@package=rapture.common) {
List failed = new ArrayList();
List ok = new ArrayList();
List overrun = new ArrayList();
List success = new ArrayList();
}
[An customizable description of a workflow layout. The detailed positions are filled in by the client based on local font sizes and the like]
@Storable(storagePath: {workflowURI})
type WorkflowGridLayout(@package=rapture.common.dp) {
String workflowURI;
List(WorkflowColumnLayout) columns;
List(WorkflowArrowLayout) arrows;
}
[Simple holder for box in a grid by column to make layout easier]
@Bean
type WorkflowColumnLayout(@package=rapture.common.dp) {
Integer columnNumber;
List(WorkflowBoxLayout) boxes;
}
[Grid details for a box in a WorkflowGridLayout]
@Bean
type WorkflowBoxLayout(@package=rapture.common.dp) {
Integer gx;
Integer gy;
String kind;
String name; // name for display, not guaranteed to be unique
String id; // rigid reference, not for display
Integer offsetX; //replace absolute offsets with fractions?
Integer offsetY;
}
[Grid details for a box in a WorkflowArrowLayout]
@Bean
type WorkflowArrowLayout(@package=rapture.common.dp) {
String name;
String fromBoxName;
String toBoxName;
Integer offsetX;
Integer offsetY;
}
[Metadata for a Rapture server group.]
@Storable(storagePath : {name}, prefix="runner/servergroup")
type RaptureServerGroup(@package=rapture.common) {
String name;
String description;
Integer jmxPort;
Set(String) inclusions;
Set(String) exclusions;
Set(String) libraries;
}
[Describes an application that this Rapture instance recognizes.]
@Storable(storagePath : {name}, prefix="runner/definition")
type RaptureApplicationDefinition(@package=rapture.common) {
String name;
String description;
String version;
}
[Describes a third-party library being used in this Rapture instance.]
@Storable(storagePath : {name}, prefix="runner/library")
type RaptureLibraryDefinition(@package=rapture.common) {
String name;
String description;
String version;
}
[Describes an active application.]
@Storable(storagePath : {serverGroup, name}, prefix="runner/instance")
type RaptureApplicationInstance(@package=rapture.common) {
String name;
String appName;
String description;
String serverGroup;
String timeRangeSpecification;
Integer retryCount = 0;
String parameters;
String apiUser;
String lockedBy;
Boolean oneShot = false;
Boolean finished = false;
String status;
Date lastStateChange;
}
[Describes the state of a currently running instance of RaptureRunner.]
@Bean
type RaptureRunnerInstanceStatus(@package=rapture.common) {
String serverGroup;
String appInstance;
String appName;
String status;
Date lastSeen;
Boolean needsRestart = false;
}
[Contains info about the capabilities that exist in the current Rapture system.]
@Storable(storagePath : {server, instanceName}, prefix="runner/capabilities", repoName=EPHEMERAL_REPO)
type RaptureInstanceCapabilities(@package=rapture.common) {
String server;
String instanceName;
Map capabilities = new HashMap();
}
[Contains status info for all instances of RaptureRunner.]
@Storable(storagePath : {serverName}, prefix="runner/status", repoName=EPHEMERAL_REPO)
type RaptureRunnerStatus(@package=rapture.common) {
String serverName;
Map statusByInstanceName;
}
[Config info for the current RaptureRunner implementation.]
@Storable(storagePath : {""}, prefix="runner/config")
type RaptureRunnerConfig(@package=rapture.common) {
Map(String, String) config;
}
[Config info for the main object in the Notification API.]
@Addressable(scheme = NOTIFICATION(false))
@Storable(storagePath : {name}, prefix="notification")
type RaptureNotificationConfig(@package=rapture.common.model) {
String name;
String config;
String purpose;
}
[The object returned by a notification]
type NotificationResult(@package=rapture.common) {
Long currentEpoch;
List(String) references;
}
[Contains all relevant data for a notification.]
type NotificationInfo(@package=rapture.common) {
String id;
String content;
String reference;
Long epoch;
Date when;
String contentType;
String who;
}
[Information about a folder or a file in a repository.]
type RaptureFolderInfo(@package=rapture.common) {
String name;
boolean folder;
}
[Parameters that should be passed to a script. Deprecated]
type RaptureParameter(@package=rapture.common) {
String name;
RaptureParameterType parameterType;
}
[Script input and output metadata.]
type ScriptInterface(@package=rapture.common) {
Map inputs;
Map outputs;
}
[Metadata for an script parameter.]
type ScriptParameter(@package=rapture.common) {
RaptureParameterType parameterType;
String description;
Object defaultValue;
}
[The status of the link between two jobs.]
@Storable(storagePath : {to, from}, prefix="joblinkstatus", repoName=EPHEMERAL_REPO)
type JobLinkStatus(@package=rapture.common) {
String from;
String to;
Integer level;
Date lastChange;
}
[The link between two jobs.]
@Storable(storagePath : {from, to}, prefix="joblink")
type JobLink(@package=rapture.common) {
String from;
String to;
}
[A response to a query on Activities]
@Bean
type ActivityQueryResponse(@package=rapture.common) {
Boolean isLast; // whether this is the last response. if false, the response is split in batches: use nextBatchId to get the rest
String nextBatchId; // a unique id that can be used to read the next batch of responses if this was not marked as isLast
List activities = new ArrayList();
}
[An activity.]
@Storable(storagePath : {id}, ttlDays=7, prefix="versioned/activity/activity/v2")
@Indexable(name: "default", fields: {id, lastSeen})
type Activity(@package=rapture.common) {
String id; // a unique id for this activity
String description; // a human-friendly description of the activity
String message; // a human-friendly text message of the status
Long progress; // how many units are complete
Long max; // the maximum units when this will be considered finished
Long lastSeen; // when the last update was done
ActivityStatus status;
}
[Holds the config info for a Rapture table, as described in the table API.]
@Addressable(scheme = TABLE(false))
@Storable(storagePath : {authority, name}, prefix="table")
type TableConfig(@package=rapture.common) {
String name;
String config;
String authority;
}
[Describes a table object.]
type TableRecord(@package=rapture.common) {
String keyName;
Map(String, Object) fields;
String content;
}
[Describes a query made against a table.]
type TableQuery(@package=rapture.common) {
List(TableSelect) fieldTests;
List(String) fieldReturns;
List(TableColumnSort) sortFields;
int skip;
int limit;
}
[An activity.]
@Deprecated("use Activity")
@Storable(storagePath : {id}, prefix="activity", repoName=EPHEMERAL_REPO)
type RaptureActivity(@package=rapture.common) {
String id;
String otherId;
String message;
Long progress;
Long maxProgress;
Boolean requestFinish = false;
Date lastSeen;
Long expiresAt;
Boolean finished = false;
}
[A structured repository definition]
@Addressable(scheme = STRUCTURED(true))
@Storable(storagePath : {authority})
type StructuredRepoConfig(@package=rapture.common) {
String description;
String config;
String authority;
}
[metadata for a column within a structured store table]
@Bean
type StructuredColumn(@package=rapture.common) {
String name;
String nativeName;
String raptureType; //TODO replace this with an enumeration
String nativeType;
}
[metadata for a table in a structured repository]
@Storable(storagePath : {authority, name})
type StructuredTable(@package=rapture.column) {
String authority;
String name;
String nativeName;
List(StructuredColumn) columns;
}
[metadata that describes the rows of a SQL table (name->datatype)]
@Bean
type TableMeta(@package=rapture.common) {
Map rows;
}
[A table index]
@Bean
type TableIndex(@package=rapture.common) {
String name;
List columns;
}
[A foreign key]
@Bean
type ForeignKey(@package=rapture.common) {
String column;
String foreignTable;
String foreignColumn;
}
[Configuration info for a repository that stores series data.]
@Addressable(scheme = SERIES(true))
@Storable(storagePath : {authority}, prefix="series")
type SeriesRepoConfig(@package=rapture.common) {
String description;
String config;
String authority;
String seriesName;
String sampleColumn;
}
[This object holds the data for a series value that was requested, without specifying what type to present data as. ]
type SeriesPoint(@package=rapture.common) {
String column;
String value;
}
[Convenience structure for casting SeriesValues to Doubles.]
type SeriesDouble(@package=rapture.common) {
String columns;
Double values;
}
[convenience structure for casting SeriesValues to Strings.]
type SeriesString(@package=rapture.common) {
String columns;
String values;
}
[The config used when archiving the data in a type.]
@Storable(storagePath : {authority, typeName}, prefix="archiveSettings", repoName=SETTINGS_REPO)
@Addressable(scheme = DOCUMENT(true))
@Cacheable
type TypeArchiveConfig(@package=rapture.common) {
String authority;
String typeName;
Boolean useScript = false;
String scriptName = "";
Long versionsToKeep = new Long(-1);
Long timeRangeToKeepInDays = new Long(-1);
}
//-----------------------
//Decision process objects
//-----------------------
[Defines a workflow. Each workflow is a flowchart of WorkflowSteps and Transitions.]
@Storable(storagePath : {workflowURI}, prefix="dp/workflow")
@Addressable(scheme = WORKFLOW(false))
@Cacheable
type Workflow(@package=rapture.common.dp) {
WorkflowURI workflowURI;
//the semaphore type and config below is used when creating Work Orders
SemaphoreType semaphoreType = SemaphoreType.UNLIMITED;
String semaphoreConfig;
List steps = new ArrayList();
String startStep; // name of the step to start on by default
String category = "alpha"; // name of the category associated with this workflow. defaults
// to "alpha", which is the category associated with RaptureAPIServer and
// RaptureComputeServer
Map view = new HashMap();
List expectedArguments = new ArrayList();
String defaultAppStatusNamePattern; //appstatus name pattern, to be used if nothing passed in to workorder
}
[An argument that's expected to be passed to a workflow]
@Bean
type ExpectedArgument(@package=rapture.common.dp) {
String name;
Boolean isRequired;
String defaultValue;
}
[An argument passed in to a workorder. Not to be confused with ExpectedArgument, which is a list of possible arguments]
@Bean
type PassedArgument(@package=rapture.common.dp) {
String name;
String value;
}
[The argument values that were passed in to a workorder]
@Storable(storagePath: {workOrderURI}, prefix="dp/woArguments", repoName=EPHEMERAL_REPO)
type WorkOrderArguments(@package=rapture.common.dp) {
WorkOrderURI workOrderURI; // the uri of the associated workorder
List arguments = new ArrayList();
}
[Points to the next step in a given sequence.]
@Bean
type Transition(@package=rapture.common.dp) {
String name;
String targetStep; // set to $RETURN:returnCode for a terminal step. the return code is the name of the
// transition to take in the step in the calling context (if any)
// targetStep is assumed to be the stepName, not a full URI
//
// We'll need to document special target steps. Currently, look at
// StepHelper to figure out what's available
}
[An executable step with config data.]
@Bean
type Step(@package=rapture.common.dp) {
String name; //this is not a URI, just a String with this step's name
String description; //this is a description of the step
String executable; // workflow:// script:// qtemplate:// $RETURN:value $SLEEP:275
// $varName or #literal -- always switch on and discard first character
Map view = new HashMap();
List transitions = new ArrayList();
String categoryOverride; //category associated with this step, if it overrides the workflow category
Integer softTimeout = -1;
}
[Each time a Workflow is executed, the execution is identified by a matching WorkOrder. A WorkOrderURI may be qualified with an id (index) of a particular Worker, e.g. workorder://myProj/myFlow#0]
@Storable(storagePath: {workOrderURI}, prefix="dp/workorder")
@Addressable(scheme = WORKORDER(false))
@Indexable(name: "default", fields: {workOrderURI, priority, startTime, endTime})
type WorkOrder(@package=rapture.common.dp) {
WorkOrderURI workOrderURI;
WorkflowURI workflowURI; //uri of Workflow used when creating this WorkOrder
List workerIds; // all ids since the start of time
List pendingIds; // top-level ids that have not terminated
Integer priority;
Long startTime; //epoch in milliseconds
Long endTime = new Long(-1); //epoch in milliseconds
SemaphoreType semaphoreType = SemaphoreType.UNLIMITED;
String semaphoreConfig;
WorkOrderExecutionState status;
}
[Holds the hash value of the initial argument values for this workorder. Useful info since two workorders with the same hash
have the same initial arguments, which means that they could be compared like for like (e.g. in terms of how long they take to run)]
@Storable(storagePath: {workOrderURI}, prefix="dp/workorder/argsHash", repoName=EPHEMERAL_REPO)
type WorkOrderInitialArgsHash(@package=rapture.common.dp) {
WorkOrderURI workOrderURI;
String hashValue;
}
[Workflow historical metrics]
@Bean
type WorkflowHistoricalMetrics(@package=rapture.common.dp) {
Double workflowAverage;
Double jobAverage;
Double workflowWithArgsAverage;
String workflowMetricName;
String jobMetricName;
String argsHashMetricName;
}
[The execution context for a WorkOrder]
@Bean
type ExecutionContext(@package=rapture.common.dp) {
WorkOrderURI workOrderURI; //the uri of the associated workorder
Map data;
}
[An individual field from the execution context for a WorkOrder]
@Storable(storagePath: {workOrderURI, varName}, prefix="dp/execontextfield", repoName=EPHEMERAL_REPO)
type ExecutionContextField(@package=rapture.common.dp) {
WorkOrderURI workOrderURI; // the uri of the associated workorder
String varName;
String value;
}
[The status of a work order.]
@Bean
type WorkOrderStatus(@package=rapture.common.dp) {
List workerIds;
WorkOrderExecutionState status; // ACTIVE, CANCELLING, CANCELLED, FINISHED, ERROR
}
[Used for multiple work orders that share the same app status name.]
@Storable(storagePath: {name}, encoding="RaptureLightweightCoder", prefix="dp/statusgroup")
type AppStatusGroup(@package=rapture.common) {
String name;
Map idToStatus = new HashMap();
}
[The status of a Rapture app.]
@Storable(storagePath: {name}, encoding="RaptureLightweightCoder", prefix="dp/appstatus")
type AppStatus(@package=rapture.common) {
String name;
WorkOrderURI workOrderURI;
WorkOrderExecutionState overallStatus;
Long lastUpdated;
}
[Deprecated! However it cannot be easily deleted because Worker contains List stepExecutionRecords
and that's going to give JSON a hard time. We need to have a strategy to handle things like this. See RAP-2596]
@Deprecated("use StepRecord")
@Bean
type StepExecutionRecord(@package=rapture.common.dp) {
WorkflowURI stepURI; //fully qualified workflowURI with stepName (e.g. //myProj/myWorkflow#myStep)
Long time; //in milliseconds
String hostname;
StepExecutionRecordType recordType;
}
[A detailed step execution record, containing start and finish times and short step name.]
@Bean
type StepRecord(@package=rapture.common.dp) {
WorkflowURI stepURI; //fully qualified workflowURI with stepName (e.g. //myProj/myWorkflow#myStep)
String name; //short step name, e.g. myStep
Long startTime;
Long endTime;
String retVal;
String hostname;
WorkOrderExecutionState status; //status of this step
ErrorWrapper exceptionInfo; //@Nullable: the rapture exception id associated with this, in case this failed and there was an exception.
String activityId; //@Nullable: the activity id associated with this step record, in case we are reporting progress on it
}
[A list of step executions for a particular Worker]
@Storable(storagePath: {workOrderURI, workerId}, prefix="dp/stepRecords", repoName=EPHEMERAL_REPO)
type StepRecordsWrapper(@package=rapture.common.dp) {
WorkOrderURI workOrderURI;
String workerId;
List stepRecords = new ArrayList();
}
[Contains additional data about the app status.]
@Bean
type AppStatusDetails(@package=rapture.common.dp) {
AppStatus appStatus;
Map> workerIdToSteps = new HashMap>();
String logURI;
Map extraContextValues = new HashMap();
}
[A request to cancel a work order.]
@Storable(storagePath: {workOrderURI})
type WorkOrderCancellation(@package=rapture.common.dp) {
WorkOrderURI workOrderURI;
Long time;
}
[Workers keep track of progress when executing a work order. Each worker handles one thread of execution.]
@Storable(storagePath: {workOrderURI, id}, prefix="dp/worker")
@Indexable(name: "default", fields: {workOrderURI, status})
type Worker(@package=rapture.common.dp) {
WorkOrderURI workOrderURI; //the uri of the associated workorder
String id;
// the view of the current step, overlayed by things such as $varName or #literal -- always switch
// on and discard first character
Map viewOverlay = new HashMap();
// fully qualified workflowURI with stepName (e.g. //myProj/myWorkflow#myStep)
List stack = new ArrayList();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy