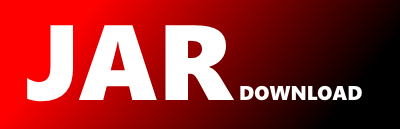
com.incapture.rapgen.TTreeDoc Maven / Gradle / Ivy
// $ANTLR 3.5.2 /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g 2016-03-01 15:22:59
package com.incapture.rapgen;
import java.util.Map;
import java.util.HashMap;
import com.incapture.rapgen.annotations.*;
import org.antlr.runtime.*;
import org.antlr.runtime.tree.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
import org.antlr.stringtemplate.*;
import org.antlr.stringtemplate.language.*;
import java.util.HashMap;
@SuppressWarnings("all")
public class TTreeDoc extends AbstractTTreeDoc {
public static final String[] tokenNames = new String[] {
"", "", "", "", "ADDRESSABLE", "API", "AT", "BANG",
"BEAN", "BOOLTYPE", "BYTEARRAYTYPE", "CACHEABLE", "CLOSESQUARE", "COLUMN",
"COMMA", "COMMENT", "CRUDTYPED", "DATA", "DATETYPE", "DEPRECATED", "DOC",
"DOT", "DOUBLETYPE", "DYNENT", "ENTITLE", "EQUAL", "EXTENDS", "FALSE",
"G_THAN", "ID", "INCLUDE", "INDEXED", "INT", "INTTYPE", "LBRAC", "LISTTYPE",
"LONGCTYPE", "LONGTYPE", "LPAREN", "L_THAN", "MAPTYPE", "MINUS", "NEW",
"OBJECTTYPE", "OPENSQUARE", "PACKAGE", "PACKAGENAME", "PRIVATE", "PUBLIC",
"RBRAC", "REGULARENTITLE", "RPAREN", "SCHEME", "SDKNAME", "SEMI", "SETTYPE",
"SQUOTE", "STORABLE", "STORAGE_PATH", "STREAMING", "STRING", "STRINGTYPE",
"TRUE", "TYPED", "VOIDTYPE", "WS", "APIENTRY", "APISEC", "CLASSTYPE",
"COMPLEX", "CRUDINFO", "CRUDINFOENTRY", "CRUDPACKAGEANNOTATION", "CRUDTYPEDEF",
"ENTITLEASPECT", "ENUM", "EXPR", "FIELD_CONSTRUCTOR", "FNNAME", "INDEX_COMPONENT",
"INDEX_NAME", "INNERA", "INNERC", "INNERT", "MAIN", "MEMBER", "MEMBERS",
"MINVERSIONDEF", "NAME", "PARAM", "PARAMS", "PRIVATEVIS", "PUBLICVIS",
"RAW", "RETTYPE", "SCRIPT", "SDKDEF", "STATEMENT", "STORABLE_ENCODING",
"STORABLE_PREFIX", "STORABLE_REPO_NAME", "STORABLE_SEPARATOR", "STORABLE_TTL_DAYS",
"STORAGE_PATH_ADDER", "STREAMINGASPECT", "TYPE", "TYPEASPECT", "TYPEDEF",
"TYPEFIELDS", "TYPEMEMBER", "VERSIONDEF", "VISIBILITY", "112", "113",
"114", "115", "116", "117", "118", "119", "120", "121", "122", "123",
"124", "125", "126", "127", "128", "129", "130", "131", "132", "133",
"134", "135", "136", "137", "138", "139"
};
public static final int EOF=-1;
public static final int ADDRESSABLE=4;
public static final int API=5;
public static final int AT=6;
public static final int BANG=7;
public static final int BEAN=8;
public static final int BOOLTYPE=9;
public static final int BYTEARRAYTYPE=10;
public static final int CACHEABLE=11;
public static final int CLOSESQUARE=12;
public static final int COLUMN=13;
public static final int COMMA=14;
public static final int COMMENT=15;
public static final int CRUDTYPED=16;
public static final int DATA=17;
public static final int DATETYPE=18;
public static final int DEPRECATED=19;
public static final int DOC=20;
public static final int DOT=21;
public static final int DOUBLETYPE=22;
public static final int DYNENT=23;
public static final int ENTITLE=24;
public static final int EQUAL=25;
public static final int EXTENDS=26;
public static final int FALSE=27;
public static final int G_THAN=28;
public static final int ID=29;
public static final int INCLUDE=30;
public static final int INDEXED=31;
public static final int INT=32;
public static final int INTTYPE=33;
public static final int LBRAC=34;
public static final int LISTTYPE=35;
public static final int LONGCTYPE=36;
public static final int LONGTYPE=37;
public static final int LPAREN=38;
public static final int L_THAN=39;
public static final int MAPTYPE=40;
public static final int MINUS=41;
public static final int NEW=42;
public static final int OBJECTTYPE=43;
public static final int OPENSQUARE=44;
public static final int PACKAGE=45;
public static final int PACKAGENAME=46;
public static final int PRIVATE=47;
public static final int PUBLIC=48;
public static final int RBRAC=49;
public static final int REGULARENTITLE=50;
public static final int RPAREN=51;
public static final int SCHEME=52;
public static final int SDKNAME=53;
public static final int SEMI=54;
public static final int SETTYPE=55;
public static final int SQUOTE=56;
public static final int STORABLE=57;
public static final int STORAGE_PATH=58;
public static final int STREAMING=59;
public static final int STRING=60;
public static final int STRINGTYPE=61;
public static final int TRUE=62;
public static final int TYPED=63;
public static final int VOIDTYPE=64;
public static final int WS=65;
public static final int APIENTRY=66;
public static final int APISEC=67;
public static final int CLASSTYPE=68;
public static final int COMPLEX=69;
public static final int CRUDINFO=70;
public static final int CRUDINFOENTRY=71;
public static final int CRUDPACKAGEANNOTATION=72;
public static final int CRUDTYPEDEF=73;
public static final int ENTITLEASPECT=74;
public static final int ENUM=75;
public static final int EXPR=76;
public static final int FIELD_CONSTRUCTOR=77;
public static final int FNNAME=78;
public static final int INDEX_COMPONENT=79;
public static final int INDEX_NAME=80;
public static final int INNERA=81;
public static final int INNERC=82;
public static final int INNERT=83;
public static final int MAIN=84;
public static final int MEMBER=85;
public static final int MEMBERS=86;
public static final int MINVERSIONDEF=87;
public static final int NAME=88;
public static final int PARAM=89;
public static final int PARAMS=90;
public static final int PRIVATEVIS=91;
public static final int PUBLICVIS=92;
public static final int RAW=93;
public static final int RETTYPE=94;
public static final int SCRIPT=95;
public static final int SDKDEF=96;
public static final int STATEMENT=97;
public static final int STORABLE_ENCODING=98;
public static final int STORABLE_PREFIX=99;
public static final int STORABLE_REPO_NAME=100;
public static final int STORABLE_SEPARATOR=101;
public static final int STORABLE_TTL_DAYS=102;
public static final int STORAGE_PATH_ADDER=103;
public static final int STREAMINGASPECT=104;
public static final int TYPE=105;
public static final int TYPEASPECT=106;
public static final int TYPEDEF=107;
public static final int TYPEFIELDS=108;
public static final int TYPEMEMBER=109;
public static final int VERSIONDEF=110;
public static final int VISIBILITY=111;
// delegates
public TTreeDoc_TTreeShared gTTreeShared;
public AbstractTTreeDoc[] getDelegates() {
return new AbstractTTreeDoc[] {gTTreeShared};
}
// delegators
public TTreeDoc(TreeNodeStream input) {
this(input, new RecognizerSharedState());
}
public TTreeDoc(TreeNodeStream input, RecognizerSharedState state) {
super(input, state);
gTTreeShared = new TTreeDoc_TTreeShared(input, state, this);
}
protected StringTemplateGroup templateLib =
new StringTemplateGroup("TTreeDocTemplates", AngleBracketTemplateLexer.class);
public void setTemplateLib(StringTemplateGroup templateLib) {
this.templateLib = templateLib;
}
public StringTemplateGroup getTemplateLib() {
return templateLib;
}
/** allows convenient multi-value initialization:
* "new STAttrMap().put(...).put(...)"
*/
@SuppressWarnings("serial")
public static class STAttrMap extends HashMap {
public STAttrMap put(String attrName, Object value) {
super.put(attrName, value);
return this;
}
}
@Override public String[] getTokenNames() { return TTreeDoc.tokenNames; }
@Override public String getGrammarFileName() { return "/Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g"; }
public static class hmxdef_return extends TreeRuleReturnScope {
public List types;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "hmxdef"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:23:1: hmxdef returns [List types] : ^( MAIN versionExpr minVerExpr ( ^( INNERT typeExpr ) | ^( INNERA a= apiExpr ) )* ) ;
public final TTreeDoc.hmxdef_return hmxdef() throws RecognitionException {
TTreeDoc.hmxdef_return retval = new TTreeDoc.hmxdef_return();
retval.start = input.LT(1);
TreeRuleReturnScope a =null;
TreeRuleReturnScope typeExpr1 =null;
retval.types = new ArrayList();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:26:4: ( ^( MAIN versionExpr minVerExpr ( ^( INNERT typeExpr ) | ^( INNERA a= apiExpr ) )* ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:26:6: ^( MAIN versionExpr minVerExpr ( ^( INNERT typeExpr ) | ^( INNERA a= apiExpr ) )* )
{
match(input,MAIN,FOLLOW_MAIN_in_hmxdef101);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_versionExpr_in_hmxdef103);
versionExpr();
state._fsp--;
pushFollow(FOLLOW_minVerExpr_in_hmxdef105);
minVerExpr();
state._fsp--;
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:27:11: ( ^( INNERT typeExpr ) | ^( INNERA a= apiExpr ) )*
loop1:
while (true) {
int alt1=3;
int LA1_0 = input.LA(1);
if ( (LA1_0==INNERT) ) {
alt1=1;
}
else if ( (LA1_0==INNERA) ) {
alt1=2;
}
switch (alt1) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:27:12: ^( INNERT typeExpr )
{
match(input,INNERT,FOLLOW_INNERT_in_hmxdef119);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_typeExpr_in_hmxdef121);
typeExpr1=typeExpr();
state._fsp--;
retval.types.add((typeExpr1!=null?((StringTemplate)typeExpr1.getTemplate()):null));
match(input, Token.UP, null);
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:28:14: ^( INNERA a= apiExpr )
{
match(input,INNERA,FOLLOW_INNERA_in_hmxdef140);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_apiExpr_in_hmxdef144);
a=apiExpr();
state._fsp--;
match(input, Token.UP, null);
}
break;
default :
break loop1;
}
}
match(input, Token.UP, null);
StringTemplate s = templateLib.getInstanceOf("docTypes",new STAttrMap().put("types", retval.types));
addApiTemplate("types.tex", "1", s);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "hmxdef"
public static class typeExpr_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "typeExpr"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:37:1: typeExpr : ^( TYPEDEF doc= DOC ( beanAnnotation | cacheableAnnotation | extendsAnnotation | deprecatedAnnotation | addressableAnnotation | storableAnnotation | indexedAnnotation )* name= ID typeAspect typeFields ) ;
public final TTreeDoc.typeExpr_return typeExpr() throws RecognitionException {
TTreeDoc.typeExpr_return retval = new TTreeDoc.typeExpr_return();
retval.start = input.LT(1);
CommonTree doc=null;
CommonTree name=null;
TreeRuleReturnScope typeFields2 =null;
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:37:10: ( ^( TYPEDEF doc= DOC ( beanAnnotation | cacheableAnnotation | extendsAnnotation | deprecatedAnnotation | addressableAnnotation | storableAnnotation | indexedAnnotation )* name= ID typeAspect typeFields ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:38:2: ^( TYPEDEF doc= DOC ( beanAnnotation | cacheableAnnotation | extendsAnnotation | deprecatedAnnotation | addressableAnnotation | storableAnnotation | indexedAnnotation )* name= ID typeAspect typeFields )
{
match(input,TYPEDEF,FOLLOW_TYPEDEF_in_typeExpr178);
match(input, Token.DOWN, null);
doc=(CommonTree)match(input,DOC,FOLLOW_DOC_in_typeExpr182);
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:38:20: ( beanAnnotation | cacheableAnnotation | extendsAnnotation | deprecatedAnnotation | addressableAnnotation | storableAnnotation | indexedAnnotation )*
loop2:
while (true) {
int alt2=8;
switch ( input.LA(1) ) {
case BEAN:
{
alt2=1;
}
break;
case CACHEABLE:
{
alt2=2;
}
break;
case EXTENDS:
{
alt2=3;
}
break;
case DEPRECATED:
{
alt2=4;
}
break;
case ADDRESSABLE:
{
alt2=5;
}
break;
case STORABLE:
{
alt2=6;
}
break;
case INDEXED:
{
alt2=7;
}
break;
}
switch (alt2) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:38:21: beanAnnotation
{
pushFollow(FOLLOW_beanAnnotation_in_typeExpr185);
beanAnnotation();
state._fsp--;
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:38:36: cacheableAnnotation
{
pushFollow(FOLLOW_cacheableAnnotation_in_typeExpr187);
cacheableAnnotation();
state._fsp--;
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:38:56: extendsAnnotation
{
pushFollow(FOLLOW_extendsAnnotation_in_typeExpr189);
extendsAnnotation();
state._fsp--;
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:38:74: deprecatedAnnotation
{
pushFollow(FOLLOW_deprecatedAnnotation_in_typeExpr191);
deprecatedAnnotation();
state._fsp--;
}
break;
case 5 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:38:95: addressableAnnotation
{
pushFollow(FOLLOW_addressableAnnotation_in_typeExpr193);
addressableAnnotation();
state._fsp--;
}
break;
case 6 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:38:117: storableAnnotation
{
pushFollow(FOLLOW_storableAnnotation_in_typeExpr195);
storableAnnotation();
state._fsp--;
}
break;
case 7 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:38:136: indexedAnnotation
{
pushFollow(FOLLOW_indexedAnnotation_in_typeExpr197);
indexedAnnotation();
state._fsp--;
}
break;
default :
break loop2;
}
}
name=(CommonTree)match(input,ID,FOLLOW_ID_in_typeExpr203);
pushFollow(FOLLOW_typeAspect_in_typeExpr205);
typeAspect();
state._fsp--;
pushFollow(FOLLOW_typeFields_in_typeExpr207);
typeFields2=typeFields();
state._fsp--;
match(input, Token.UP, null);
String d = doc.getText();
d = d.substring(1,d.length()-1);
retval.st = templateLib.getInstanceOf("docTypeDef",new STAttrMap().put("name", name).put("fields", (typeFields2!=null?((TTreeDoc.typeFields_return)typeFields2).fields:null)).put("doc", d));
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "typeExpr"
public static class typeAspect_return extends TreeRuleReturnScope {
public String name;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "typeAspect"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:44:1: typeAspect returns [String name] : ^( TYPEASPECT p= PACKAGENAME ) ;
public final TTreeDoc.typeAspect_return typeAspect() throws RecognitionException {
TTreeDoc.typeAspect_return retval = new TTreeDoc.typeAspect_return();
retval.start = input.LT(1);
CommonTree p=null;
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:44:33: ( ^( TYPEASPECT p= PACKAGENAME ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:45:5: ^( TYPEASPECT p= PACKAGENAME )
{
match(input,TYPEASPECT,FOLLOW_TYPEASPECT_in_typeAspect226);
match(input, Token.DOWN, null);
p=(CommonTree)match(input,PACKAGENAME,FOLLOW_PACKAGENAME_in_typeAspect230);
match(input, Token.UP, null);
retval.name = (p!=null?p.getText():null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "typeAspect"
public static class typeFields_return extends TreeRuleReturnScope {
public List fields;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "typeFields"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:47:1: typeFields returns [List fields] : ^( TYPEFIELDS (t= typeFieldDef )* ) ;
public final TTreeDoc.typeFields_return typeFields() throws RecognitionException {
TTreeDoc.typeFields_return retval = new TTreeDoc.typeFields_return();
retval.start = input.LT(1);
TreeRuleReturnScope t =null;
retval.fields = new ArrayList();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:50:3: ( ^( TYPEFIELDS (t= typeFieldDef )* ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:51:5: ^( TYPEFIELDS (t= typeFieldDef )* )
{
match(input,TYPEFIELDS,FOLLOW_TYPEFIELDS_in_typeFields255);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:51:18: (t= typeFieldDef )*
loop3:
while (true) {
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0==TYPEMEMBER) ) {
alt3=1;
}
switch (alt3) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:51:19: t= typeFieldDef
{
pushFollow(FOLLOW_typeFieldDef_in_typeFields260);
t=typeFieldDef();
state._fsp--;
retval.fields.add((t!=null?((StringTemplate)t.getTemplate()):null));
}
break;
default :
break loop3;
}
}
match(input, Token.UP, null);
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "typeFields"
public static class typeFieldDef_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "typeFieldDef"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:53:1: typeFieldDef : ^( TYPEMEMBER ^( TYPE vartype ) ^( NAME ID ) ^( FIELD_CONSTRUCTOR ( fieldConstructor )? ) ) ;
public final TTreeDoc.typeFieldDef_return typeFieldDef() throws RecognitionException {
TTreeDoc.typeFieldDef_return retval = new TTreeDoc.typeFieldDef_return();
retval.start = input.LT(1);
CommonTree ID3=null;
TreeRuleReturnScope vartype4 =null;
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:53:14: ( ^( TYPEMEMBER ^( TYPE vartype ) ^( NAME ID ) ^( FIELD_CONSTRUCTOR ( fieldConstructor )? ) ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:54:5: ^( TYPEMEMBER ^( TYPE vartype ) ^( NAME ID ) ^( FIELD_CONSTRUCTOR ( fieldConstructor )? ) )
{
match(input,TYPEMEMBER,FOLLOW_TYPEMEMBER_in_typeFieldDef278);
match(input, Token.DOWN, null);
match(input,TYPE,FOLLOW_TYPE_in_typeFieldDef281);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_vartype_in_typeFieldDef283);
vartype4=vartype();
state._fsp--;
match(input, Token.UP, null);
match(input,NAME,FOLLOW_NAME_in_typeFieldDef287);
match(input, Token.DOWN, null);
ID3=(CommonTree)match(input,ID,FOLLOW_ID_in_typeFieldDef289);
match(input, Token.UP, null);
match(input,FIELD_CONSTRUCTOR,FOLLOW_FIELD_CONSTRUCTOR_in_typeFieldDef293);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:54:65: ( fieldConstructor )?
int alt4=2;
int LA4_0 = input.LA(1);
if ( ((LA4_0 >= ADDRESSABLE && LA4_0 <= 139)) ) {
alt4=1;
}
switch (alt4) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:54:65: fieldConstructor
{
pushFollow(FOLLOW_fieldConstructor_in_typeFieldDef295);
fieldConstructor();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
}
match(input, Token.UP, null);
retval.st = templateLib.getInstanceOf("docTypeField",new STAttrMap().put("name", ID3 ).put("type", (vartype4!=null?(input.getTokenStream().toString(input.getTreeAdaptor().getTokenStartIndex(vartype4.start),input.getTreeAdaptor().getTokenStopIndex(vartype4.start))):null)));
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "typeFieldDef"
public static class vartype_return extends TreeRuleReturnScope {
public String mainType;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "vartype"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:62:1: vartype returns [String mainType] : ( ^( RAW INTTYPE ) | ^( RAW LONGTYPE ) | ^( RAW DATETYPE ) | ^( RAW OBJECTTYPE ) | ^( RAW LONGCTYPE ) | ^( RAW BOOLTYPE ) | ^( RAW DOUBLETYPE ) | ^( RAW STRINGTYPE ) | ^( RAW BYTEARRAYTYPE ) | ^( RAW VOIDTYPE ) | ^( LISTTYPE v= vartype ) | ^( SETTYPE v= vartype ) | ^( MAPTYPE k= vartype v= vartype ) | ^( COMPLEX typeid= ID ) );
public final TTreeDoc.vartype_return vartype() throws RecognitionException {
TTreeDoc.vartype_return retval = new TTreeDoc.vartype_return();
retval.start = input.LT(1);
CommonTree typeid=null;
TreeRuleReturnScope v =null;
TreeRuleReturnScope k =null;
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:62:34: ( ^( RAW INTTYPE ) | ^( RAW LONGTYPE ) | ^( RAW DATETYPE ) | ^( RAW OBJECTTYPE ) | ^( RAW LONGCTYPE ) | ^( RAW BOOLTYPE ) | ^( RAW DOUBLETYPE ) | ^( RAW STRINGTYPE ) | ^( RAW BYTEARRAYTYPE ) | ^( RAW VOIDTYPE ) | ^( LISTTYPE v= vartype ) | ^( SETTYPE v= vartype ) | ^( MAPTYPE k= vartype v= vartype ) | ^( COMPLEX typeid= ID ) )
int alt5=14;
switch ( input.LA(1) ) {
case RAW:
{
int LA5_1 = input.LA(2);
if ( (LA5_1==DOWN) ) {
switch ( input.LA(3) ) {
case INTTYPE:
{
alt5=1;
}
break;
case LONGTYPE:
{
alt5=2;
}
break;
case DATETYPE:
{
alt5=3;
}
break;
case OBJECTTYPE:
{
alt5=4;
}
break;
case LONGCTYPE:
{
alt5=5;
}
break;
case BOOLTYPE:
{
alt5=6;
}
break;
case DOUBLETYPE:
{
alt5=7;
}
break;
case STRINGTYPE:
{
alt5=8;
}
break;
case BYTEARRAYTYPE:
{
alt5=9;
}
break;
case VOIDTYPE:
{
alt5=10;
}
break;
default:
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 5, 6, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 5, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case LISTTYPE:
{
alt5=11;
}
break;
case SETTYPE:
{
alt5=12;
}
break;
case MAPTYPE:
{
alt5=13;
}
break;
case COMPLEX:
{
alt5=14;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 5, 0, input);
throw nvae;
}
switch (alt5) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:63:5: ^( RAW INTTYPE )
{
match(input,RAW,FOLLOW_RAW_in_vartype319);
match(input, Token.DOWN, null);
match(input,INTTYPE,FOLLOW_INTTYPE_in_vartype321);
match(input, Token.UP, null);
retval.mainType = "int";
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:64:7: ^( RAW LONGTYPE )
{
match(input,RAW,FOLLOW_RAW_in_vartype333);
match(input, Token.DOWN, null);
match(input,LONGTYPE,FOLLOW_LONGTYPE_in_vartype335);
match(input, Token.UP, null);
retval.mainType = "long";
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:65:7: ^( RAW DATETYPE )
{
match(input,RAW,FOLLOW_RAW_in_vartype347);
match(input, Token.DOWN, null);
match(input,DATETYPE,FOLLOW_DATETYPE_in_vartype349);
match(input, Token.UP, null);
retval.mainType = "Date";
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:66:7: ^( RAW OBJECTTYPE )
{
match(input,RAW,FOLLOW_RAW_in_vartype361);
match(input, Token.DOWN, null);
match(input,OBJECTTYPE,FOLLOW_OBJECTTYPE_in_vartype363);
match(input, Token.UP, null);
retval.mainType = "Object";
}
break;
case 5 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:67:7: ^( RAW LONGCTYPE )
{
match(input,RAW,FOLLOW_RAW_in_vartype375);
match(input, Token.DOWN, null);
match(input,LONGCTYPE,FOLLOW_LONGCTYPE_in_vartype377);
match(input, Token.UP, null);
retval.mainType = "Long";
}
break;
case 6 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:68:7: ^( RAW BOOLTYPE )
{
match(input,RAW,FOLLOW_RAW_in_vartype389);
match(input, Token.DOWN, null);
match(input,BOOLTYPE,FOLLOW_BOOLTYPE_in_vartype391);
match(input, Token.UP, null);
retval.mainType = "boolean";
}
break;
case 7 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:69:7: ^( RAW DOUBLETYPE )
{
match(input,RAW,FOLLOW_RAW_in_vartype403);
match(input, Token.DOWN, null);
match(input,DOUBLETYPE,FOLLOW_DOUBLETYPE_in_vartype405);
match(input, Token.UP, null);
retval.mainType = "double";
}
break;
case 8 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:70:7: ^( RAW STRINGTYPE )
{
match(input,RAW,FOLLOW_RAW_in_vartype417);
match(input, Token.DOWN, null);
match(input,STRINGTYPE,FOLLOW_STRINGTYPE_in_vartype419);
match(input, Token.UP, null);
retval.mainType = "String";
}
break;
case 9 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:71:7: ^( RAW BYTEARRAYTYPE )
{
match(input,RAW,FOLLOW_RAW_in_vartype431);
match(input, Token.DOWN, null);
match(input,BYTEARRAYTYPE,FOLLOW_BYTEARRAYTYPE_in_vartype433);
match(input, Token.UP, null);
retval.mainType = "byte[]";
}
break;
case 10 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:72:7: ^( RAW VOIDTYPE )
{
match(input,RAW,FOLLOW_RAW_in_vartype445);
match(input, Token.DOWN, null);
match(input,VOIDTYPE,FOLLOW_VOIDTYPE_in_vartype447);
match(input, Token.UP, null);
retval.mainType = "void";
}
break;
case 11 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:73:7: ^( LISTTYPE v= vartype )
{
match(input,LISTTYPE,FOLLOW_LISTTYPE_in_vartype459);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_vartype_in_vartype463);
v=vartype();
state._fsp--;
match(input, Token.UP, null);
retval.mainType = "List<" + (v!=null?((TTreeDoc.vartype_return)v).mainType:null) + ">" ;
}
break;
case 12 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:74:7: ^( SETTYPE v= vartype )
{
match(input,SETTYPE,FOLLOW_SETTYPE_in_vartype475);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_vartype_in_vartype479);
v=vartype();
state._fsp--;
match(input, Token.UP, null);
retval.mainType = "Set<" + (v!=null?((TTreeDoc.vartype_return)v).mainType:null) + ">" ;
}
break;
case 13 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:75:7: ^( MAPTYPE k= vartype v= vartype )
{
match(input,MAPTYPE,FOLLOW_MAPTYPE_in_vartype491);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_vartype_in_vartype495);
k=vartype();
state._fsp--;
pushFollow(FOLLOW_vartype_in_vartype499);
v=vartype();
state._fsp--;
match(input, Token.UP, null);
retval.mainType = "Map<" + (k!=null?((TTreeDoc.vartype_return)k).mainType:null) + "," + (v!=null?((TTreeDoc.vartype_return)v).mainType:null) + ">" ;
}
break;
case 14 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:76:7: ^( COMPLEX typeid= ID )
{
match(input,COMPLEX,FOLLOW_COMPLEX_in_vartype511);
match(input, Token.DOWN, null);
typeid=(CommonTree)match(input,ID,FOLLOW_ID_in_vartype515);
match(input, Token.UP, null);
retval.mainType = (typeid!=null?typeid.getText():null);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "vartype"
protected static class apiExpr_scope {
String apiName;
}
protected Stack apiExpr_stack = new Stack();
public static class apiExpr_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "apiExpr"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:78:1: apiExpr : ^( APISEC doc= DOC (deprecated= deprecatedAspect )? apiType= ID (a= apilist ) ) ;
public final TTreeDoc.apiExpr_return apiExpr() throws RecognitionException {
apiExpr_stack.push(new apiExpr_scope());
TTreeDoc.apiExpr_return retval = new TTreeDoc.apiExpr_return();
retval.start = input.LT(1);
CommonTree doc=null;
CommonTree apiType=null;
TreeRuleReturnScope deprecated =null;
TreeRuleReturnScope a =null;
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:80:4: ( ^( APISEC doc= DOC (deprecated= deprecatedAspect )? apiType= ID (a= apilist ) ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:81:4: ^( APISEC doc= DOC (deprecated= deprecatedAspect )? apiType= ID (a= apilist ) )
{
match(input,APISEC,FOLLOW_APISEC_in_apiExpr534);
match(input, Token.DOWN, null);
doc=(CommonTree)match(input,DOC,FOLLOW_DOC_in_apiExpr538);
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:81:31: (deprecated= deprecatedAspect )?
int alt6=2;
int LA6_0 = input.LA(1);
if ( (LA6_0==DEPRECATED) ) {
alt6=1;
}
switch (alt6) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:81:31: deprecated= deprecatedAspect
{
pushFollow(FOLLOW_deprecatedAspect_in_apiExpr542);
deprecated=deprecatedAspect();
state._fsp--;
}
break;
}
apiType=(CommonTree)match(input,ID,FOLLOW_ID_in_apiExpr547);
apiExpr_stack.peek().apiName = (apiType!=null?apiType.getText():null);
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:81:100: (a= apilist )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:81:101: a= apilist
{
pushFollow(FOLLOW_apilist_in_apiExpr554);
a=apilist();
state._fsp--;
}
match(input, Token.UP, null);
// This is the definition of a set of apis in the same "class", so we
// need to emit at this level the summary navigation page that has
// links to the names of the other pages
// Imports for all types referenced
String d = doc.getText();
d = d.substring(1,d.length()-1);
// Construct the template for the API - not the passed apis etc are collected at the lower level
StringTemplate s = templateLib.getInstanceOf("docApiList",new STAttrMap().put("apitype", (apiType!=null?apiType.getText():null)).put("apis", (a!=null?((TTreeDoc.apilist_return)a).fns:null)).put("doc", d));
addApiTemplate((apiType!=null?apiType.getText():null) + ".tex", "1", s);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
apiExpr_stack.pop();
}
return retval;
}
// $ANTLR end "apiExpr"
public static class apilist_return extends TreeRuleReturnScope {
public List fns;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "apilist"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:99:1: apilist returns [List fns] : (a= apistmt )+ ;
public final TTreeDoc.apilist_return apilist() throws RecognitionException {
TTreeDoc.apilist_return retval = new TTreeDoc.apilist_return();
retval.start = input.LT(1);
TreeRuleReturnScope a =null;
retval.fns = new ArrayList();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:103:3: ( (a= apistmt )+ )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:103:3: (a= apistmt )+
{
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:103:3: (a= apistmt )+
int cnt7=0;
loop7:
while (true) {
int alt7=2;
int LA7_0 = input.LA(1);
if ( (LA7_0==APIENTRY) ) {
alt7=1;
}
switch (alt7) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:103:4: a= apistmt
{
pushFollow(FOLLOW_apistmt_in_apilist580);
a=apistmt();
state._fsp--;
retval.fns.add((a!=null?((StringTemplate)a.getTemplate()):null));
}
break;
default :
if ( cnt7 >= 1 ) break loop7;
EarlyExitException eee = new EarlyExitException(7, input);
throw eee;
}
cnt7++;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "apilist"
public static class deprecatedAspect_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "deprecatedAspect"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:107:1: deprecatedAspect : ( ( DEPRECATED ID ) | ( DEPRECATED STRING ) );
public final TTreeDoc.deprecatedAspect_return deprecatedAspect() throws RecognitionException {
TTreeDoc.deprecatedAspect_return retval = new TTreeDoc.deprecatedAspect_return();
retval.start = input.LT(1);
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:108:5: ( ( DEPRECATED ID ) | ( DEPRECATED STRING ) )
int alt8=2;
int LA8_0 = input.LA(1);
if ( (LA8_0==DEPRECATED) ) {
int LA8_1 = input.LA(2);
if ( (LA8_1==ID) ) {
alt8=1;
}
else if ( (LA8_1==STRING) ) {
alt8=2;
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 8, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 8, 0, input);
throw nvae;
}
switch (alt8) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:108:7: ( DEPRECATED ID )
{
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:108:7: ( DEPRECATED ID )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:108:9: DEPRECATED ID
{
match(input,DEPRECATED,FOLLOW_DEPRECATED_in_deprecatedAspect598);
match(input,ID,FOLLOW_ID_in_deprecatedAspect600);
}
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:109:7: ( DEPRECATED STRING )
{
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:109:7: ( DEPRECATED STRING )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:109:9: DEPRECATED STRING
{
match(input,DEPRECATED,FOLLOW_DEPRECATED_in_deprecatedAspect612);
match(input,STRING,FOLLOW_STRING_in_deprecatedAspect614);
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "deprecatedAspect"
public static class visibility_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "visibility"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:113:1: visibility : ( PRIVATEVIS | PUBLICVIS );
public final TTreeDoc.visibility_return visibility() throws RecognitionException {
TTreeDoc.visibility_return retval = new TTreeDoc.visibility_return();
retval.start = input.LT(1);
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:113:12: ( PRIVATEVIS | PUBLICVIS )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:
{
if ( (input.LA(1) >= PRIVATEVIS && input.LA(1) <= PUBLICVIS) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "visibility"
public static class apistmt_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "apistmt"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:115:1: apistmt : ^( APIENTRY doc= DOC ^( ENTITLEASPECT (rent= REGULARENTITLE )+ (k= ID v= ID )* ) ( deprecatedAspect )? ^( VISIBILITY visibility ) ^( RETTYPE vartype ) ^( FNNAME name= ID ) p= paramList ) ;
public final TTreeDoc.apistmt_return apistmt() throws RecognitionException {
TTreeDoc.apistmt_return retval = new TTreeDoc.apistmt_return();
retval.start = input.LT(1);
CommonTree doc=null;
CommonTree rent=null;
CommonTree k=null;
CommonTree v=null;
CommonTree name=null;
TreeRuleReturnScope p =null;
TreeRuleReturnScope vartype5 =null;
List regularEntitlements = new ArrayList();
Map dynamicEntMap = new HashMap();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:120:3: ( ^( APIENTRY doc= DOC ^( ENTITLEASPECT (rent= REGULARENTITLE )+ (k= ID v= ID )* ) ( deprecatedAspect )? ^( VISIBILITY visibility ) ^( RETTYPE vartype ) ^( FNNAME name= ID ) p= paramList ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:120:3: ^( APIENTRY doc= DOC ^( ENTITLEASPECT (rent= REGULARENTITLE )+ (k= ID v= ID )* ) ( deprecatedAspect )? ^( VISIBILITY visibility ) ^( RETTYPE vartype ) ^( FNNAME name= ID ) p= paramList )
{
match(input,APIENTRY,FOLLOW_APIENTRY_in_apistmt648);
match(input, Token.DOWN, null);
doc=(CommonTree)match(input,DOC,FOLLOW_DOC_in_apistmt652);
match(input,ENTITLEASPECT,FOLLOW_ENTITLEASPECT_in_apistmt655);
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:120:38: (rent= REGULARENTITLE )+
int cnt9=0;
loop9:
while (true) {
int alt9=2;
int LA9_0 = input.LA(1);
if ( (LA9_0==REGULARENTITLE) ) {
alt9=1;
}
switch (alt9) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:120:39: rent= REGULARENTITLE
{
rent=(CommonTree)match(input,REGULARENTITLE,FOLLOW_REGULARENTITLE_in_apistmt660);
regularEntitlements.add((rent!=null?rent.getText():null));
}
break;
default :
if ( cnt9 >= 1 ) break loop9;
EarlyExitException eee = new EarlyExitException(9, input);
throw eee;
}
cnt9++;
}
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:120:100: (k= ID v= ID )*
loop10:
while (true) {
int alt10=2;
int LA10_0 = input.LA(1);
if ( (LA10_0==ID) ) {
alt10=1;
}
switch (alt10) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:120:101: k= ID v= ID
{
k=(CommonTree)match(input,ID,FOLLOW_ID_in_apistmt669);
v=(CommonTree)match(input,ID,FOLLOW_ID_in_apistmt673);
dynamicEntMap.put((k!=null?k.getText():null), (v!=null?v.getText():null));
}
break;
default :
break loop10;
}
}
match(input, Token.UP, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:120:153: ( deprecatedAspect )?
int alt11=2;
int LA11_0 = input.LA(1);
if ( (LA11_0==DEPRECATED) ) {
alt11=1;
}
switch (alt11) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:120:153: deprecatedAspect
{
pushFollow(FOLLOW_deprecatedAspect_in_apistmt680);
deprecatedAspect();
state._fsp--;
}
break;
}
match(input,VISIBILITY,FOLLOW_VISIBILITY_in_apistmt684);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_visibility_in_apistmt686);
visibility();
state._fsp--;
match(input, Token.UP, null);
match(input,RETTYPE,FOLLOW_RETTYPE_in_apistmt690);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_vartype_in_apistmt692);
vartype5=vartype();
state._fsp--;
match(input, Token.UP, null);
match(input,FNNAME,FOLLOW_FNNAME_in_apistmt696);
match(input, Token.DOWN, null);
name=(CommonTree)match(input,ID,FOLLOW_ID_in_apistmt700);
match(input, Token.UP, null);
pushFollow(FOLLOW_paramList_in_apistmt705);
p=paramList();
state._fsp--;
match(input, Token.UP, null);
// An apistmt needs to generate in two files - the api file (which is done at a higher level) and in
// a whole new set of files which are dependent on the fnName
// This is a single api call
String d = doc.getText();
d = d.substring(1,d.length()-1);
String ent = reassembleFullEntitlmentPath(regularEntitlements, dynamicEntMap);
retval.st = templateLib.getInstanceOf("docApiEntry",new STAttrMap().put("apitype", apiExpr_stack.peek().apiName).put("ret", (vartype5!=null?((TTreeDoc.vartype_return)vartype5).mainType:null)).put("name", (name!=null?name.getText():null)).put("apiparams", (p!=null?((TTreeDoc.paramList_return)p).paramList:null)).put("paramNames", (p!=null?((TTreeDoc.paramList_return)p).paramNames:null)).put("doc", d).put("ent", ent));
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "apistmt"
public static class paramList_return extends TreeRuleReturnScope {
public List paramList;
public List paramNames;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "paramList"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:131:1: paramList returns [List paramList, List paramNames] : ^( PARAMS (a= param )* ) ;
public final TTreeDoc.paramList_return paramList() throws RecognitionException {
TTreeDoc.paramList_return retval = new TTreeDoc.paramList_return();
retval.start = input.LT(1);
TreeRuleReturnScope a =null;
retval.paramList = new ArrayList();
retval.paramNames = new ArrayList();
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:136:2: ( ^( PARAMS (a= param )* ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:137:2: ^( PARAMS (a= param )* )
{
match(input,PARAMS,FOLLOW_PARAMS_in_paramList727);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:137:11: (a= param )*
loop12:
while (true) {
int alt12=2;
int LA12_0 = input.LA(1);
if ( (LA12_0==PARAM) ) {
alt12=1;
}
switch (alt12) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:137:12: a= param
{
pushFollow(FOLLOW_param_in_paramList732);
a=param();
state._fsp--;
retval.paramList.add((a!=null?((StringTemplate)a.getTemplate()):null));
retval.paramNames.add((a!=null?((TTreeDoc.param_return)a).name:null));
}
break;
default :
break loop12;
}
}
match(input, Token.UP, null);
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "paramList"
public static class param_return extends TreeRuleReturnScope {
public String name;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "param"
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:142:1: param returns [String name] : ^( PARAM ^( TYPE t= vartype ) ^( NAME n= ID ) ( BANG )? ) ;
public final TTreeDoc.param_return param() throws RecognitionException {
TTreeDoc.param_return retval = new TTreeDoc.param_return();
retval.start = input.LT(1);
CommonTree n=null;
TreeRuleReturnScope t =null;
try {
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:142:27: ( ^( PARAM ^( TYPE t= vartype ) ^( NAME n= ID ) ( BANG )? ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:142:30: ^( PARAM ^( TYPE t= vartype ) ^( NAME n= ID ) ( BANG )? )
{
match(input,PARAM,FOLLOW_PARAM_in_param749);
match(input, Token.DOWN, null);
match(input,TYPE,FOLLOW_TYPE_in_param752);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_vartype_in_param756);
t=vartype();
state._fsp--;
match(input, Token.UP, null);
match(input,NAME,FOLLOW_NAME_in_param760);
match(input, Token.DOWN, null);
n=(CommonTree)match(input,ID,FOLLOW_ID_in_param764);
match(input, Token.UP, null);
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:142:69: ( BANG )?
int alt13=2;
int LA13_0 = input.LA(1);
if ( (LA13_0==BANG) ) {
alt13=1;
}
switch (alt13) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/CodeGen/CodeGenLib/src/main/antlr3/com/incapture/rapgen/TTreeDoc.g:142:69: BANG
{
match(input,BANG,FOLLOW_BANG_in_param767);
}
break;
}
match(input, Token.UP, null);
retval.name = (n!=null?n.getText():null);
retval.st = templateLib.getInstanceOf("param",new STAttrMap().put("type", (t!=null?((TTreeDoc.vartype_return)t).mainType:null)).put("name", (n!=null?n.getText():null)));
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "param"
// Delegated rules
public TTreeDoc_TTreeShared.fieldConstructor_return fieldConstructor() throws RecognitionException { return gTTreeShared.fieldConstructor(); }
public TTreeDoc_TTreeShared.addressableAnnotation_return addressableAnnotation() throws RecognitionException { return gTTreeShared.addressableAnnotation(); }
public TTreeDoc_TTreeShared.cacheableAnnotation_return cacheableAnnotation() throws RecognitionException { return gTTreeShared.cacheableAnnotation(); }
public TTreeDoc_TTreeShared.storableAnnotation_return storableAnnotation() throws RecognitionException { return gTTreeShared.storableAnnotation(); }
public TTreeDoc_TTreeShared.sdkExpr_return sdkExpr() throws RecognitionException { return gTTreeShared.sdkExpr(); }
public TTreeDoc_TTreeShared.beanAnnotation_return beanAnnotation() throws RecognitionException { return gTTreeShared.beanAnnotation(); }
public TTreeDoc_TTreeShared.minVerExpr_return minVerExpr() throws RecognitionException { return gTTreeShared.minVerExpr(); }
public TTreeDoc_TTreeShared.indexedAnnotation_return indexedAnnotation() throws RecognitionException { return gTTreeShared.indexedAnnotation(); }
public TTreeDoc_TTreeShared.deprecatedAnnotation_return deprecatedAnnotation() throws RecognitionException { return gTTreeShared.deprecatedAnnotation(); }
public TTreeDoc_TTreeShared.versionExpr_return versionExpr() throws RecognitionException { return gTTreeShared.versionExpr(); }
public TTreeDoc_TTreeShared.extendsAnnotation_return extendsAnnotation() throws RecognitionException { return gTTreeShared.extendsAnnotation(); }
public static final BitSet FOLLOW_MAIN_in_hmxdef101 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_versionExpr_in_hmxdef103 = new BitSet(new long[]{0x0000000000000000L,0x0000000000800000L});
public static final BitSet FOLLOW_minVerExpr_in_hmxdef105 = new BitSet(new long[]{0x0000000000000008L,0x00000000000A0000L});
public static final BitSet FOLLOW_INNERT_in_hmxdef119 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_typeExpr_in_hmxdef121 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_INNERA_in_hmxdef140 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_apiExpr_in_hmxdef144 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_TYPEDEF_in_typeExpr178 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_DOC_in_typeExpr182 = new BitSet(new long[]{0x02000000A4080910L});
public static final BitSet FOLLOW_beanAnnotation_in_typeExpr185 = new BitSet(new long[]{0x02000000A4080910L});
public static final BitSet FOLLOW_cacheableAnnotation_in_typeExpr187 = new BitSet(new long[]{0x02000000A4080910L});
public static final BitSet FOLLOW_extendsAnnotation_in_typeExpr189 = new BitSet(new long[]{0x02000000A4080910L});
public static final BitSet FOLLOW_deprecatedAnnotation_in_typeExpr191 = new BitSet(new long[]{0x02000000A4080910L});
public static final BitSet FOLLOW_addressableAnnotation_in_typeExpr193 = new BitSet(new long[]{0x02000000A4080910L});
public static final BitSet FOLLOW_storableAnnotation_in_typeExpr195 = new BitSet(new long[]{0x02000000A4080910L});
public static final BitSet FOLLOW_indexedAnnotation_in_typeExpr197 = new BitSet(new long[]{0x02000000A4080910L});
public static final BitSet FOLLOW_ID_in_typeExpr203 = new BitSet(new long[]{0x0000000000000000L,0x0000040000000000L});
public static final BitSet FOLLOW_typeAspect_in_typeExpr205 = new BitSet(new long[]{0x0000000000000000L,0x0000100000000000L});
public static final BitSet FOLLOW_typeFields_in_typeExpr207 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_TYPEASPECT_in_typeAspect226 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_PACKAGENAME_in_typeAspect230 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_TYPEFIELDS_in_typeFields255 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_typeFieldDef_in_typeFields260 = new BitSet(new long[]{0x0000000000000008L,0x0000200000000000L});
public static final BitSet FOLLOW_TYPEMEMBER_in_typeFieldDef278 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_TYPE_in_typeFieldDef281 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_vartype_in_typeFieldDef283 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_NAME_in_typeFieldDef287 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_typeFieldDef289 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_FIELD_CONSTRUCTOR_in_typeFieldDef293 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_fieldConstructor_in_typeFieldDef295 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_RAW_in_vartype319 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_INTTYPE_in_vartype321 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_RAW_in_vartype333 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_LONGTYPE_in_vartype335 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_RAW_in_vartype347 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_DATETYPE_in_vartype349 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_RAW_in_vartype361 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_OBJECTTYPE_in_vartype363 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_RAW_in_vartype375 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_LONGCTYPE_in_vartype377 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_RAW_in_vartype389 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_BOOLTYPE_in_vartype391 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_RAW_in_vartype403 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_DOUBLETYPE_in_vartype405 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_RAW_in_vartype417 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_STRINGTYPE_in_vartype419 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_RAW_in_vartype431 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_BYTEARRAYTYPE_in_vartype433 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_RAW_in_vartype445 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_VOIDTYPE_in_vartype447 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_LISTTYPE_in_vartype459 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_vartype_in_vartype463 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_SETTYPE_in_vartype475 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_vartype_in_vartype479 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_MAPTYPE_in_vartype491 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_vartype_in_vartype495 = new BitSet(new long[]{0x0080010800000000L,0x0000000020000020L});
public static final BitSet FOLLOW_vartype_in_vartype499 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_COMPLEX_in_vartype511 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_vartype515 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_APISEC_in_apiExpr534 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_DOC_in_apiExpr538 = new BitSet(new long[]{0x0000000020080000L});
public static final BitSet FOLLOW_deprecatedAspect_in_apiExpr542 = new BitSet(new long[]{0x0000000020000000L});
public static final BitSet FOLLOW_ID_in_apiExpr547 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000004L});
public static final BitSet FOLLOW_apilist_in_apiExpr554 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_apistmt_in_apilist580 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000004L});
public static final BitSet FOLLOW_DEPRECATED_in_deprecatedAspect598 = new BitSet(new long[]{0x0000000020000000L});
public static final BitSet FOLLOW_ID_in_deprecatedAspect600 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DEPRECATED_in_deprecatedAspect612 = new BitSet(new long[]{0x1000000000000000L});
public static final BitSet FOLLOW_STRING_in_deprecatedAspect614 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_APIENTRY_in_apistmt648 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_DOC_in_apistmt652 = new BitSet(new long[]{0x0000000000000000L,0x0000000000000400L});
public static final BitSet FOLLOW_ENTITLEASPECT_in_apistmt655 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_REGULARENTITLE_in_apistmt660 = new BitSet(new long[]{0x0004000020000008L});
public static final BitSet FOLLOW_ID_in_apistmt669 = new BitSet(new long[]{0x0000000020000000L});
public static final BitSet FOLLOW_ID_in_apistmt673 = new BitSet(new long[]{0x0000000020000008L});
public static final BitSet FOLLOW_deprecatedAspect_in_apistmt680 = new BitSet(new long[]{0x0000000000000000L,0x0000800000000000L});
public static final BitSet FOLLOW_VISIBILITY_in_apistmt684 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_visibility_in_apistmt686 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_RETTYPE_in_apistmt690 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_vartype_in_apistmt692 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_FNNAME_in_apistmt696 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_apistmt700 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_paramList_in_apistmt705 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_PARAMS_in_paramList727 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_param_in_paramList732 = new BitSet(new long[]{0x0000000000000008L,0x0000000002000000L});
public static final BitSet FOLLOW_PARAM_in_param749 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_TYPE_in_param752 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_vartype_in_param756 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_NAME_in_param760 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_param764 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_BANG_in_param767 = new BitSet(new long[]{0x0000000000000008L});
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy