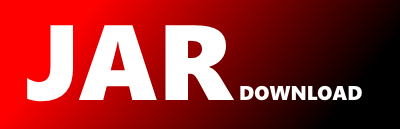
com.incapture.rapgen.TTreeRuby_TTreeShared Maven / Gradle / Ivy
// $ANTLR 3.5.2 TTreeShared.g 2016-04-14 13:13:57
package com.incapture.rapgen;
import com.incapture.rapgen.annotations.*;
import org.antlr.runtime.*;
import org.antlr.runtime.tree.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
import org.antlr.stringtemplate.*;
import org.antlr.stringtemplate.language.*;
import java.util.HashMap;
@SuppressWarnings("all")
public class TTreeRuby_TTreeShared extends AbstractTTree {
public static final int EOF=-1;
public static final int ADDRESSABLE=4;
public static final int API=5;
public static final int AT=6;
public static final int BANG=7;
public static final int BEAN=8;
public static final int BOOLTYPE=9;
public static final int BYTEARRAYTYPE=10;
public static final int CACHEABLE=11;
public static final int CLOSESQUARE=12;
public static final int COLUMN=13;
public static final int COMMA=14;
public static final int COMMENT=15;
public static final int CRUDTYPED=16;
public static final int DATA=17;
public static final int DATETYPE=18;
public static final int DEPRECATED=19;
public static final int DOC=20;
public static final int DOT=21;
public static final int DOUBLETYPE=22;
public static final int DYNENT=23;
public static final int ENTITLE=24;
public static final int EQUAL=25;
public static final int EXTENDS=26;
public static final int FALSE=27;
public static final int G_THAN=28;
public static final int ID=29;
public static final int INCLUDE=30;
public static final int INDEXED=31;
public static final int INT=32;
public static final int INTTYPE=33;
public static final int LBRAC=34;
public static final int LISTTYPE=35;
public static final int LONGCTYPE=36;
public static final int LONGTYPE=37;
public static final int LPAREN=38;
public static final int L_THAN=39;
public static final int MAPTYPE=40;
public static final int MINUS=41;
public static final int NEW=42;
public static final int OBJECTTYPE=43;
public static final int OPENSQUARE=44;
public static final int PACKAGE=45;
public static final int PACKAGENAME=46;
public static final int PRIVATE=47;
public static final int PUBLIC=48;
public static final int RBRAC=49;
public static final int REGULARENTITLE=50;
public static final int RPAREN=51;
public static final int SCHEME=52;
public static final int SDKNAME=53;
public static final int SEMI=54;
public static final int SETTYPE=55;
public static final int SQUOTE=56;
public static final int STORABLE=57;
public static final int STORAGE_PATH=58;
public static final int STREAMING=59;
public static final int STRING=60;
public static final int STRINGTYPE=61;
public static final int TRUE=62;
public static final int TYPED=63;
public static final int VOIDTYPE=64;
public static final int WS=65;
public static final int APIENTRY=66;
public static final int APISEC=67;
public static final int CLASSTYPE=68;
public static final int COMPLEX=69;
public static final int CRUDINFO=70;
public static final int CRUDINFOENTRY=71;
public static final int CRUDPACKAGEANNOTATION=72;
public static final int CRUDTYPEDEF=73;
public static final int ENTITLEASPECT=74;
public static final int ENUM=75;
public static final int EXPR=76;
public static final int FIELD_CONSTRUCTOR=77;
public static final int FNNAME=78;
public static final int INDEX_COMPONENT=79;
public static final int INDEX_NAME=80;
public static final int INNERA=81;
public static final int INNERC=82;
public static final int INNERT=83;
public static final int MAIN=84;
public static final int MEMBER=85;
public static final int MEMBERS=86;
public static final int MINVERSIONDEF=87;
public static final int NAME=88;
public static final int PARAM=89;
public static final int PARAMS=90;
public static final int PRIVATEVIS=91;
public static final int PUBLICVIS=92;
public static final int RAW=93;
public static final int RETTYPE=94;
public static final int SCRIPT=95;
public static final int SDKDEF=96;
public static final int STATEMENT=97;
public static final int STORABLE_ENCODING=98;
public static final int STORABLE_PREFIX=99;
public static final int STORABLE_REPO_NAME=100;
public static final int STORABLE_SEPARATOR=101;
public static final int STORABLE_TTL_DAYS=102;
public static final int STORAGE_PATH_ADDER=103;
public static final int STREAMINGASPECT=104;
public static final int TYPE=105;
public static final int TYPEASPECT=106;
public static final int TYPEDEF=107;
public static final int TYPEFIELDS=108;
public static final int TYPEMEMBER=109;
public static final int VERSIONDEF=110;
public static final int VISIBILITY=111;
// delegates
public AbstractTTree[] getDelegates() {
return new AbstractTTree[] {};
}
// delegators
public TTreeRuby gTTreeRuby;
public TTreeRuby gParent;
public TTreeRuby_TTreeShared(TreeNodeStream input, TTreeRuby gTTreeRuby) {
this(input, new RecognizerSharedState(), gTTreeRuby);
}
public TTreeRuby_TTreeShared(TreeNodeStream input, RecognizerSharedState state, TTreeRuby gTTreeRuby) {
super(input, state);
this.gTTreeRuby = gTTreeRuby;
gParent = gTTreeRuby;
}
protected StringTemplateGroup templateLib =
new StringTemplateGroup("TTreeRuby_TTreeSharedTemplates", AngleBracketTemplateLexer.class);
public void setTemplateLib(StringTemplateGroup templateLib) {
this.templateLib = templateLib;
}
public StringTemplateGroup getTemplateLib() {
return templateLib;
}
/** allows convenient multi-value initialization:
* "new STAttrMap().put(...).put(...)"
*/
@SuppressWarnings("serial")
public static class STAttrMap extends HashMap {
public STAttrMap put(String attrName, Object value) {
super.put(attrName, value);
return this;
}
}
@Override public String[] getTokenNames() { return TTreeRuby.tokenNames; }
@Override public String getGrammarFileName() { return "TTreeShared.g"; }
public static class sdkExpr_return extends TreeRuleReturnScope {
public String name;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "sdkExpr"
// TTreeShared.g:11:1: sdkExpr returns [String name] : ^( SDKDEF ID ) ;
public final TTreeRuby_TTreeShared.sdkExpr_return sdkExpr() throws RecognitionException {
TTreeRuby_TTreeShared.sdkExpr_return retval = new TTreeRuby_TTreeShared.sdkExpr_return();
retval.start = input.LT(1);
CommonTree ID1=null;
try {
// TTreeShared.g:11:30: ( ^( SDKDEF ID ) )
// TTreeShared.g:11:32: ^( SDKDEF ID )
{
match(input,SDKDEF,FOLLOW_SDKDEF_in_sdkExpr32);
match(input, Token.DOWN, null);
ID1=(CommonTree)match(input,ID,FOLLOW_ID_in_sdkExpr34);
match(input, Token.UP, null);
retval.name = (ID1!=null?ID1.getText():null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "sdkExpr"
public static class versionExpr_return extends TreeRuleReturnScope {
public int major;
public int minor;
public int micro;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "versionExpr"
// TTreeShared.g:13:1: versionExpr returns [int major, int minor, int micro] : ^(def= VERSIONDEF majorLocal= INT minorLocal= INT (microLocal= INT )? ) ;
public final TTreeRuby_TTreeShared.versionExpr_return versionExpr() throws RecognitionException {
TTreeRuby_TTreeShared.versionExpr_return retval = new TTreeRuby_TTreeShared.versionExpr_return();
retval.start = input.LT(1);
CommonTree def=null;
CommonTree majorLocal=null;
CommonTree minorLocal=null;
CommonTree microLocal=null;
try {
// TTreeShared.g:13:54: ( ^(def= VERSIONDEF majorLocal= INT minorLocal= INT (microLocal= INT )? ) )
// TTreeShared.g:13:56: ^(def= VERSIONDEF majorLocal= INT minorLocal= INT (microLocal= INT )? )
{
def=(CommonTree)match(input,VERSIONDEF,FOLLOW_VERSIONDEF_in_versionExpr51);
match(input, Token.DOWN, null);
majorLocal=(CommonTree)match(input,INT,FOLLOW_INT_in_versionExpr55);
minorLocal=(CommonTree)match(input,INT,FOLLOW_INT_in_versionExpr59);
// TTreeShared.g:13:102: (microLocal= INT )?
int alt1=2;
int LA1_0 = input.LA(1);
if ( (LA1_0==INT) ) {
alt1=1;
}
switch (alt1) {
case 1 :
// TTreeShared.g:13:104: microLocal= INT
{
microLocal=(CommonTree)match(input,INT,FOLLOW_INT_in_versionExpr64);
}
break;
}
match(input, Token.UP, null);
retval.major = Integer.parseInt((majorLocal!=null?majorLocal.getText():null));
retval.minor = Integer.parseInt((minorLocal!=null?minorLocal.getText():null));
if (microLocal != null && (microLocal!=null?microLocal.getText():null).length() > 0) {
retval.micro = Integer.parseInt((microLocal!=null?microLocal.getText():null));
}
else {
retval.micro = 0; //assume 0 if no macro specified
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "versionExpr"
public static class minVerExpr_return extends TreeRuleReturnScope {
public int major;
public int minor;
public int micro;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "minVerExpr"
// TTreeShared.g:24:1: minVerExpr returns [int major, int minor, int micro] : ^(def= MINVERSIONDEF majorLocal= INT minorLocal= INT (microLocal= INT )? ) ;
public final TTreeRuby_TTreeShared.minVerExpr_return minVerExpr() throws RecognitionException {
TTreeRuby_TTreeShared.minVerExpr_return retval = new TTreeRuby_TTreeShared.minVerExpr_return();
retval.start = input.LT(1);
CommonTree def=null;
CommonTree majorLocal=null;
CommonTree minorLocal=null;
CommonTree microLocal=null;
try {
// TTreeShared.g:24:53: ( ^(def= MINVERSIONDEF majorLocal= INT minorLocal= INT (microLocal= INT )? ) )
// TTreeShared.g:24:55: ^(def= MINVERSIONDEF majorLocal= INT minorLocal= INT (microLocal= INT )? )
{
def=(CommonTree)match(input,MINVERSIONDEF,FOLLOW_MINVERSIONDEF_in_minVerExpr83);
match(input, Token.DOWN, null);
majorLocal=(CommonTree)match(input,INT,FOLLOW_INT_in_minVerExpr87);
minorLocal=(CommonTree)match(input,INT,FOLLOW_INT_in_minVerExpr91);
// TTreeShared.g:24:104: (microLocal= INT )?
int alt2=2;
int LA2_0 = input.LA(1);
if ( (LA2_0==INT) ) {
alt2=1;
}
switch (alt2) {
case 1 :
// TTreeShared.g:24:106: microLocal= INT
{
microLocal=(CommonTree)match(input,INT,FOLLOW_INT_in_minVerExpr96);
}
break;
}
match(input, Token.UP, null);
retval.major = Integer.parseInt((majorLocal!=null?majorLocal.getText():null));
retval.minor = Integer.parseInt((minorLocal!=null?minorLocal.getText():null));
if (microLocal != null && (microLocal!=null?microLocal.getText():null).length() > 0) {
retval.micro = Integer.parseInt((microLocal!=null?microLocal.getText():null));
}
else {
retval.micro = 0; //assume 0 if no macro specified
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "minVerExpr"
public static class beanAnnotation_return extends TreeRuleReturnScope {
public BeanAnnotation result;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "beanAnnotation"
// TTreeShared.g:36:1: beanAnnotation returns [BeanAnnotation result] : BEAN ;
public final TTreeRuby_TTreeShared.beanAnnotation_return beanAnnotation() throws RecognitionException {
TTreeRuby_TTreeShared.beanAnnotation_return retval = new TTreeRuby_TTreeShared.beanAnnotation_return();
retval.start = input.LT(1);
try {
// TTreeShared.g:38:5: ( BEAN )
// TTreeShared.g:38:7: BEAN
{
match(input,BEAN,FOLLOW_BEAN_in_beanAnnotation119);
retval.result = new BeanAnnotation();
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "beanAnnotation"
public static class cacheableAnnotation_return extends TreeRuleReturnScope {
public CacheableAnnotation result;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "cacheableAnnotation"
// TTreeShared.g:41:1: cacheableAnnotation returns [CacheableAnnotation result] : CACHEABLE ( LBRAC ID EQUAL ( TRUE | FALSE ) RBRAC )? ;
public final TTreeRuby_TTreeShared.cacheableAnnotation_return cacheableAnnotation() throws RecognitionException {
TTreeRuby_TTreeShared.cacheableAnnotation_return retval = new TTreeRuby_TTreeShared.cacheableAnnotation_return();
retval.start = input.LT(1);
try {
// TTreeShared.g:42:5: ( CACHEABLE ( LBRAC ID EQUAL ( TRUE | FALSE ) RBRAC )? )
// TTreeShared.g:42:7: CACHEABLE ( LBRAC ID EQUAL ( TRUE | FALSE ) RBRAC )?
{
match(input,CACHEABLE,FOLLOW_CACHEABLE_in_cacheableAnnotation142);
retval.result = new CacheableAnnotation();
// TTreeShared.g:42:58: ( LBRAC ID EQUAL ( TRUE | FALSE ) RBRAC )?
int alt4=2;
int LA4_0 = input.LA(1);
if ( (LA4_0==LBRAC) ) {
alt4=1;
}
switch (alt4) {
case 1 :
// TTreeShared.g:42:59: LBRAC ID EQUAL ( TRUE | FALSE ) RBRAC
{
match(input,LBRAC,FOLLOW_LBRAC_in_cacheableAnnotation147);
match(input,ID,FOLLOW_ID_in_cacheableAnnotation149);
match(input,EQUAL,FOLLOW_EQUAL_in_cacheableAnnotation151);
// TTreeShared.g:42:74: ( TRUE | FALSE )
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0==TRUE) ) {
alt3=1;
}
else if ( (LA3_0==FALSE) ) {
alt3=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 3, 0, input);
throw nvae;
}
switch (alt3) {
case 1 :
// TTreeShared.g:42:75: TRUE
{
match(input,TRUE,FOLLOW_TRUE_in_cacheableAnnotation154);
retval.result.setShouldCacheNulls(true);
}
break;
case 2 :
// TTreeShared.g:42:121: FALSE
{
match(input,FALSE,FOLLOW_FALSE_in_cacheableAnnotation160);
}
break;
}
match(input,RBRAC,FOLLOW_RBRAC_in_cacheableAnnotation163);
}
break;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "cacheableAnnotation"
protected static class addressableAnnotation_scope {
boolean isPrimitive;
}
protected Stack addressableAnnotation_stack = new Stack();
public static class addressableAnnotation_return extends TreeRuleReturnScope {
public AddressableAnnotation result;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "addressableAnnotation"
// TTreeShared.g:45:1: addressableAnnotation returns [AddressableAnnotation result] : ADDRESSABLE ID ( TRUE | FALSE )? ;
public final TTreeRuby_TTreeShared.addressableAnnotation_return addressableAnnotation() throws RecognitionException {
addressableAnnotation_stack.push(new addressableAnnotation_scope());
TTreeRuby_TTreeShared.addressableAnnotation_return retval = new TTreeRuby_TTreeShared.addressableAnnotation_return();
retval.start = input.LT(1);
CommonTree ID2=null;
addressableAnnotation_stack.peek().isPrimitive = false;
try {
// TTreeShared.g:53:5: ( ADDRESSABLE ID ( TRUE | FALSE )? )
// TTreeShared.g:53:7: ADDRESSABLE ID ( TRUE | FALSE )?
{
match(input,ADDRESSABLE,FOLLOW_ADDRESSABLE_in_addressableAnnotation200);
ID2=(CommonTree)match(input,ID,FOLLOW_ID_in_addressableAnnotation202);
// TTreeShared.g:54:9: ( TRUE | FALSE )?
int alt5=3;
int LA5_0 = input.LA(1);
if ( (LA5_0==TRUE) ) {
alt5=1;
}
else if ( (LA5_0==FALSE) ) {
alt5=2;
}
switch (alt5) {
case 1 :
// TTreeShared.g:54:10: TRUE
{
match(input,TRUE,FOLLOW_TRUE_in_addressableAnnotation214);
addressableAnnotation_stack.peek().isPrimitive = true;
}
break;
case 2 :
// TTreeShared.g:55:11: FALSE
{
match(input,FALSE,FOLLOW_FALSE_in_addressableAnnotation229);
addressableAnnotation_stack.peek().isPrimitive = false;
}
break;
}
retval.result = new AddressableAnnotation((ID2!=null?ID2.getText():null), addressableAnnotation_stack.peek().isPrimitive);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
addressableAnnotation_stack.pop();
}
return retval;
}
// $ANTLR end "addressableAnnotation"
protected static class storableAnnotation_scope {
StorableAnnotation annotation;
}
protected Stack storableAnnotation_stack = new Stack();
public static class storableAnnotation_return extends TreeRuleReturnScope {
public StorableAnnotation result;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "storableAnnotation"
// TTreeShared.g:59:1: storableAnnotation returns [StorableAnnotation result] : STORABLE ( ^( STORAGE_PATH_ADDER (s1= STRING |field= ID ) ) )+ ( ^( STORABLE_SEPARATOR separator= STRING ) )? ( ^( STORABLE_ENCODING encoding= STRING ) )? ( ^( STORABLE_TTL_DAYS ttlValue= INT ) )? ( ^( STORABLE_PREFIX prefix= STRING ) )? ( ^( STORABLE_REPO_NAME repoConstant= ID ) )? ( ^( STORABLE_REPO_NAME repoName= STRING ) )? ;
public final TTreeRuby_TTreeShared.storableAnnotation_return storableAnnotation() throws RecognitionException {
storableAnnotation_stack.push(new storableAnnotation_scope());
TTreeRuby_TTreeShared.storableAnnotation_return retval = new TTreeRuby_TTreeShared.storableAnnotation_return();
retval.start = input.LT(1);
CommonTree s1=null;
CommonTree field=null;
CommonTree separator=null;
CommonTree encoding=null;
CommonTree ttlValue=null;
CommonTree prefix=null;
CommonTree repoConstant=null;
CommonTree repoName=null;
storableAnnotation_stack.peek().annotation = new StorableAnnotation();
try {
// TTreeShared.g:67:5: ( STORABLE ( ^( STORAGE_PATH_ADDER (s1= STRING |field= ID ) ) )+ ( ^( STORABLE_SEPARATOR separator= STRING ) )? ( ^( STORABLE_ENCODING encoding= STRING ) )? ( ^( STORABLE_TTL_DAYS ttlValue= INT ) )? ( ^( STORABLE_PREFIX prefix= STRING ) )? ( ^( STORABLE_REPO_NAME repoConstant= ID ) )? ( ^( STORABLE_REPO_NAME repoName= STRING ) )? )
// TTreeShared.g:67:7: STORABLE ( ^( STORAGE_PATH_ADDER (s1= STRING |field= ID ) ) )+ ( ^( STORABLE_SEPARATOR separator= STRING ) )? ( ^( STORABLE_ENCODING encoding= STRING ) )? ( ^( STORABLE_TTL_DAYS ttlValue= INT ) )? ( ^( STORABLE_PREFIX prefix= STRING ) )? ( ^( STORABLE_REPO_NAME repoConstant= ID ) )? ( ^( STORABLE_REPO_NAME repoName= STRING ) )?
{
match(input,STORABLE,FOLLOW_STORABLE_in_storableAnnotation282);
// TTreeShared.g:68:7: ( ^( STORAGE_PATH_ADDER (s1= STRING |field= ID ) ) )+
int cnt7=0;
loop7:
while (true) {
int alt7=2;
int LA7_0 = input.LA(1);
if ( (LA7_0==STORAGE_PATH_ADDER) ) {
alt7=1;
}
switch (alt7) {
case 1 :
// TTreeShared.g:68:8: ^( STORAGE_PATH_ADDER (s1= STRING |field= ID ) )
{
match(input,STORAGE_PATH_ADDER,FOLLOW_STORAGE_PATH_ADDER_in_storableAnnotation293);
match(input, Token.DOWN, null);
// TTreeShared.g:69:8: (s1= STRING |field= ID )
int alt6=2;
int LA6_0 = input.LA(1);
if ( (LA6_0==STRING) ) {
alt6=1;
}
else if ( (LA6_0==ID) ) {
alt6=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 6, 0, input);
throw nvae;
}
switch (alt6) {
case 1 :
// TTreeShared.g:69:9: s1= STRING
{
s1=(CommonTree)match(input,STRING,FOLLOW_STRING_in_storableAnnotation306);
storableAnnotation_stack.peek().annotation.addField((s1!=null?s1.getText():null), com.incapture.rapgen.annotations.storable.StorableFieldType.STRING);
}
break;
case 2 :
// TTreeShared.g:70:10: field= ID
{
field=(CommonTree)match(input,ID,FOLLOW_ID_in_storableAnnotation321);
storableAnnotation_stack.peek().annotation.addField((field!=null?field.getText():null), com.incapture.rapgen.annotations.storable.StorableFieldType.ID);
}
break;
}
match(input, Token.UP, null);
}
break;
default :
if ( cnt7 >= 1 ) break loop7;
EarlyExitException eee = new EarlyExitException(7, input);
throw eee;
}
cnt7++;
}
// TTreeShared.g:72:7: ( ^( STORABLE_SEPARATOR separator= STRING ) )?
int alt8=2;
int LA8_0 = input.LA(1);
if ( (LA8_0==STORABLE_SEPARATOR) ) {
alt8=1;
}
switch (alt8) {
case 1 :
// TTreeShared.g:72:8: ^( STORABLE_SEPARATOR separator= STRING )
{
match(input,STORABLE_SEPARATOR,FOLLOW_STORABLE_SEPARATOR_in_storableAnnotation344);
match(input, Token.DOWN, null);
separator=(CommonTree)match(input,STRING,FOLLOW_STRING_in_storableAnnotation348);
storableAnnotation_stack.peek().annotation.setSeparator((separator!=null?separator.getText():null));
match(input, Token.UP, null);
}
break;
}
// TTreeShared.g:73:7: ( ^( STORABLE_ENCODING encoding= STRING ) )?
int alt9=2;
int LA9_0 = input.LA(1);
if ( (LA9_0==STORABLE_ENCODING) ) {
alt9=1;
}
switch (alt9) {
case 1 :
// TTreeShared.g:73:8: ^( STORABLE_ENCODING encoding= STRING )
{
match(input,STORABLE_ENCODING,FOLLOW_STORABLE_ENCODING_in_storableAnnotation364);
match(input, Token.DOWN, null);
encoding=(CommonTree)match(input,STRING,FOLLOW_STRING_in_storableAnnotation368);
storableAnnotation_stack.peek().annotation.setEncodingType((encoding!=null?encoding.getText():null));
match(input, Token.UP, null);
}
break;
}
// TTreeShared.g:74:7: ( ^( STORABLE_TTL_DAYS ttlValue= INT ) )?
int alt10=2;
int LA10_0 = input.LA(1);
if ( (LA10_0==STORABLE_TTL_DAYS) ) {
alt10=1;
}
switch (alt10) {
case 1 :
// TTreeShared.g:74:8: ^( STORABLE_TTL_DAYS ttlValue= INT )
{
match(input,STORABLE_TTL_DAYS,FOLLOW_STORABLE_TTL_DAYS_in_storableAnnotation384);
match(input, Token.DOWN, null);
ttlValue=(CommonTree)match(input,INT,FOLLOW_INT_in_storableAnnotation388);
storableAnnotation_stack.peek().annotation.setTtlDays(ttlValue);
match(input, Token.UP, null);
}
break;
}
// TTreeShared.g:75:7: ( ^( STORABLE_PREFIX prefix= STRING ) )?
int alt11=2;
int LA11_0 = input.LA(1);
if ( (LA11_0==STORABLE_PREFIX) ) {
alt11=1;
}
switch (alt11) {
case 1 :
// TTreeShared.g:75:8: ^( STORABLE_PREFIX prefix= STRING )
{
match(input,STORABLE_PREFIX,FOLLOW_STORABLE_PREFIX_in_storableAnnotation404);
match(input, Token.DOWN, null);
prefix=(CommonTree)match(input,STRING,FOLLOW_STRING_in_storableAnnotation408);
storableAnnotation_stack.peek().annotation.setPrefix((prefix!=null?prefix.getText():null));
match(input, Token.UP, null);
}
break;
}
// TTreeShared.g:76:7: ( ^( STORABLE_REPO_NAME repoConstant= ID ) )?
int alt12=2;
int LA12_0 = input.LA(1);
if ( (LA12_0==STORABLE_REPO_NAME) ) {
int LA12_1 = input.LA(2);
if ( (LA12_1==DOWN) ) {
int LA12_3 = input.LA(3);
if ( (LA12_3==ID) ) {
alt12=1;
}
}
}
switch (alt12) {
case 1 :
// TTreeShared.g:76:8: ^( STORABLE_REPO_NAME repoConstant= ID )
{
match(input,STORABLE_REPO_NAME,FOLLOW_STORABLE_REPO_NAME_in_storableAnnotation424);
match(input, Token.DOWN, null);
repoConstant=(CommonTree)match(input,ID,FOLLOW_ID_in_storableAnnotation428);
storableAnnotation_stack.peek().annotation.setRepoConstant((repoConstant!=null?repoConstant.getText():null));
match(input, Token.UP, null);
}
break;
}
// TTreeShared.g:77:7: ( ^( STORABLE_REPO_NAME repoName= STRING ) )?
int alt13=2;
int LA13_0 = input.LA(1);
if ( (LA13_0==STORABLE_REPO_NAME) ) {
alt13=1;
}
switch (alt13) {
case 1 :
// TTreeShared.g:77:8: ^( STORABLE_REPO_NAME repoName= STRING )
{
match(input,STORABLE_REPO_NAME,FOLLOW_STORABLE_REPO_NAME_in_storableAnnotation444);
match(input, Token.DOWN, null);
repoName=(CommonTree)match(input,STRING,FOLLOW_STRING_in_storableAnnotation448);
storableAnnotation_stack.peek().annotation.setRepoName((repoName!=null?repoName.getText():null));
match(input, Token.UP, null);
}
break;
}
retval.result = storableAnnotation_stack.peek().annotation;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
storableAnnotation_stack.pop();
}
return retval;
}
// $ANTLR end "storableAnnotation"
public static class deprecatedAnnotation_return extends TreeRuleReturnScope {
public DeprecatedAnnotation result;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "deprecatedAnnotation"
// TTreeShared.g:81:1: deprecatedAnnotation returns [DeprecatedAnnotation result] : ( ( DEPRECATED ID ) | ( DEPRECATED STRING ) );
public final TTreeRuby_TTreeShared.deprecatedAnnotation_return deprecatedAnnotation() throws RecognitionException {
TTreeRuby_TTreeShared.deprecatedAnnotation_return retval = new TTreeRuby_TTreeShared.deprecatedAnnotation_return();
retval.start = input.LT(1);
CommonTree ID3=null;
CommonTree STRING4=null;
try {
// TTreeShared.g:83:5: ( ( DEPRECATED ID ) | ( DEPRECATED STRING ) )
int alt14=2;
int LA14_0 = input.LA(1);
if ( (LA14_0==DEPRECATED) ) {
int LA14_1 = input.LA(2);
if ( (LA14_1==ID) ) {
alt14=1;
}
else if ( (LA14_1==STRING) ) {
alt14=2;
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 14, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 14, 0, input);
throw nvae;
}
switch (alt14) {
case 1 :
// TTreeShared.g:83:7: ( DEPRECATED ID )
{
// TTreeShared.g:83:7: ( DEPRECATED ID )
// TTreeShared.g:83:9: DEPRECATED ID
{
match(input,DEPRECATED,FOLLOW_DEPRECATED_in_deprecatedAnnotation487);
ID3=(CommonTree)match(input,ID,FOLLOW_ID_in_deprecatedAnnotation489);
retval.result = new DeprecatedAnnotation((ID3!=null?ID3.getText():null));
}
}
break;
case 2 :
// TTreeShared.g:84:7: ( DEPRECATED STRING )
{
// TTreeShared.g:84:7: ( DEPRECATED STRING )
// TTreeShared.g:84:9: DEPRECATED STRING
{
match(input,DEPRECATED,FOLLOW_DEPRECATED_in_deprecatedAnnotation504);
STRING4=(CommonTree)match(input,STRING,FOLLOW_STRING_in_deprecatedAnnotation506);
retval.result = new DeprecatedAnnotation((STRING4!=null?STRING4.getText():null).substring(1, (STRING4!=null?STRING4.getText():null).length() -1));
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "deprecatedAnnotation"
protected static class indexedAnnotation_scope {
IndexedAnnotation annotation;
}
protected Stack indexedAnnotation_stack = new Stack();
public static class indexedAnnotation_return extends TreeRuleReturnScope {
public IndexedAnnotation result;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "indexedAnnotation"
// TTreeShared.g:87:1: indexedAnnotation returns [IndexedAnnotation result] : INDEXED ^( INDEX_NAME ( STRING ) ) ( ^( INDEX_COMPONENT ( ID ) ) )+ ;
public final TTreeRuby_TTreeShared.indexedAnnotation_return indexedAnnotation() throws RecognitionException {
indexedAnnotation_stack.push(new indexedAnnotation_scope());
TTreeRuby_TTreeShared.indexedAnnotation_return retval = new TTreeRuby_TTreeShared.indexedAnnotation_return();
retval.start = input.LT(1);
CommonTree STRING5=null;
CommonTree ID6=null;
indexedAnnotation_stack.peek().annotation = new IndexedAnnotation();
try {
// TTreeShared.g:95:5: ( INDEXED ^( INDEX_NAME ( STRING ) ) ( ^( INDEX_COMPONENT ( ID ) ) )+ )
// TTreeShared.g:95:7: INDEXED ^( INDEX_NAME ( STRING ) ) ( ^( INDEX_COMPONENT ( ID ) ) )+
{
match(input,INDEXED,FOLLOW_INDEXED_in_indexedAnnotation550);
match(input,INDEX_NAME,FOLLOW_INDEX_NAME_in_indexedAnnotation553);
match(input, Token.DOWN, null);
// TTreeShared.g:95:27: ( STRING )
// TTreeShared.g:95:28: STRING
{
STRING5=(CommonTree)match(input,STRING,FOLLOW_STRING_in_indexedAnnotation555);
indexedAnnotation_stack.peek().annotation.setName((STRING5!=null?STRING5.getText():null).substring(1, (STRING5!=null?STRING5.getText():null).length() - 1));
}
match(input, Token.UP, null);
// TTreeShared.g:96:7: ( ^( INDEX_COMPONENT ( ID ) ) )+
int cnt15=0;
loop15:
while (true) {
int alt15=2;
int LA15_0 = input.LA(1);
if ( (LA15_0==INDEX_COMPONENT) ) {
alt15=1;
}
switch (alt15) {
case 1 :
// TTreeShared.g:96:8: ^( INDEX_COMPONENT ( ID ) )
{
match(input,INDEX_COMPONENT,FOLLOW_INDEX_COMPONENT_in_indexedAnnotation570);
match(input, Token.DOWN, null);
// TTreeShared.g:96:25: ( ID )
// TTreeShared.g:96:26: ID
{
ID6=(CommonTree)match(input,ID,FOLLOW_ID_in_indexedAnnotation572);
indexedAnnotation_stack.peek().annotation.addField((ID6!=null?ID6.getText():null));
}
match(input, Token.UP, null);
}
break;
default :
if ( cnt15 >= 1 ) break loop15;
EarlyExitException eee = new EarlyExitException(15, input);
throw eee;
}
cnt15++;
}
retval.result = indexedAnnotation_stack.peek().annotation;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
indexedAnnotation_stack.pop();
}
return retval;
}
// $ANTLR end "indexedAnnotation"
protected static class extendsAnnotation_scope {
StringBuilder sb;
}
protected Stack extendsAnnotation_stack = new Stack();
public static class extendsAnnotation_return extends TreeRuleReturnScope {
public ExtendsAnnotation result;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "extendsAnnotation"
// TTreeShared.g:100:1: extendsAnnotation returns [ExtendsAnnotation result] : EXTENDS PACKAGENAME ID ;
public final TTreeRuby_TTreeShared.extendsAnnotation_return extendsAnnotation() throws RecognitionException {
extendsAnnotation_stack.push(new extendsAnnotation_scope());
TTreeRuby_TTreeShared.extendsAnnotation_return retval = new TTreeRuby_TTreeShared.extendsAnnotation_return();
retval.start = input.LT(1);
CommonTree PACKAGENAME7=null;
CommonTree ID8=null;
extendsAnnotation_stack.peek().sb = new StringBuilder();
try {
// TTreeShared.g:108:5: ( EXTENDS PACKAGENAME ID )
// TTreeShared.g:108:7: EXTENDS PACKAGENAME ID
{
match(input,EXTENDS,FOLLOW_EXTENDS_in_extendsAnnotation618);
PACKAGENAME7=(CommonTree)match(input,PACKAGENAME,FOLLOW_PACKAGENAME_in_extendsAnnotation620);
ID8=(CommonTree)match(input,ID,FOLLOW_ID_in_extendsAnnotation622);
retval.result = new ExtendsAnnotation((PACKAGENAME7!=null?PACKAGENAME7.getText():null) + "." + (ID8!=null?ID8.getText():null));
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
extendsAnnotation_stack.pop();
}
return retval;
}
// $ANTLR end "extendsAnnotation"
protected static class fieldConstructor_scope {
StringBuilder sb;
}
protected Stack fieldConstructor_stack = new Stack();
public static class fieldConstructor_return extends TreeRuleReturnScope {
public String constructor;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "fieldConstructor"
// TTreeShared.g:112:1: fieldConstructor returns [String constructor] : (val= NEW )? (val= (~ NEW ) )+ ;
public final TTreeRuby_TTreeShared.fieldConstructor_return fieldConstructor() throws RecognitionException {
fieldConstructor_stack.push(new fieldConstructor_scope());
TTreeRuby_TTreeShared.fieldConstructor_return retval = new TTreeRuby_TTreeShared.fieldConstructor_return();
retval.start = input.LT(1);
CommonTree val=null;
fieldConstructor_stack.peek().sb = new StringBuilder();
try {
// TTreeShared.g:119:5: ( (val= NEW )? (val= (~ NEW ) )+ )
// TTreeShared.g:120:7: (val= NEW )? (val= (~ NEW ) )+
{
// TTreeShared.g:120:7: (val= NEW )?
int alt16=2;
int LA16_0 = input.LA(1);
if ( (LA16_0==NEW) ) {
alt16=1;
}
switch (alt16) {
case 1 :
// TTreeShared.g:120:8: val= NEW
{
val=(CommonTree)match(input,NEW,FOLLOW_NEW_in_fieldConstructor668);
fieldConstructor_stack.peek().sb.append(val).append(" ");
}
break;
}
// TTreeShared.g:121:7: (val= (~ NEW ) )+
int cnt17=0;
loop17:
while (true) {
int alt17=2;
int LA17_0 = input.LA(1);
if ( ((LA17_0 >= ADDRESSABLE && LA17_0 <= MINUS)||(LA17_0 >= OBJECTTYPE && LA17_0 <= 139)) ) {
alt17=1;
}
switch (alt17) {
case 1 :
// TTreeShared.g:121:8: val= (~ NEW )
{
val=(CommonTree)input.LT(1);
if ( (input.LA(1) >= ADDRESSABLE && input.LA(1) <= MINUS)||(input.LA(1) >= OBJECTTYPE && input.LA(1) <= 139) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
fieldConstructor_stack.peek().sb.append(val);
}
break;
default :
if ( cnt17 >= 1 ) break loop17;
EarlyExitException eee = new EarlyExitException(17, input);
throw eee;
}
cnt17++;
}
retval.constructor = fieldConstructor_stack.peek().sb.toString();
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
fieldConstructor_stack.pop();
}
return retval;
}
// $ANTLR end "fieldConstructor"
// Delegated rules
public static final BitSet FOLLOW_SDKDEF_in_sdkExpr32 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_sdkExpr34 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_VERSIONDEF_in_versionExpr51 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_INT_in_versionExpr55 = new BitSet(new long[]{0x0000000100000000L});
public static final BitSet FOLLOW_INT_in_versionExpr59 = new BitSet(new long[]{0x0000000100000008L});
public static final BitSet FOLLOW_INT_in_versionExpr64 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_MINVERSIONDEF_in_minVerExpr83 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_INT_in_minVerExpr87 = new BitSet(new long[]{0x0000000100000000L});
public static final BitSet FOLLOW_INT_in_minVerExpr91 = new BitSet(new long[]{0x0000000100000008L});
public static final BitSet FOLLOW_INT_in_minVerExpr96 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_BEAN_in_beanAnnotation119 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CACHEABLE_in_cacheableAnnotation142 = new BitSet(new long[]{0x0000000400000002L});
public static final BitSet FOLLOW_LBRAC_in_cacheableAnnotation147 = new BitSet(new long[]{0x0000000020000000L});
public static final BitSet FOLLOW_ID_in_cacheableAnnotation149 = new BitSet(new long[]{0x0000000002000000L});
public static final BitSet FOLLOW_EQUAL_in_cacheableAnnotation151 = new BitSet(new long[]{0x4000000008000000L});
public static final BitSet FOLLOW_TRUE_in_cacheableAnnotation154 = new BitSet(new long[]{0x0002000000000000L});
public static final BitSet FOLLOW_FALSE_in_cacheableAnnotation160 = new BitSet(new long[]{0x0002000000000000L});
public static final BitSet FOLLOW_RBRAC_in_cacheableAnnotation163 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ADDRESSABLE_in_addressableAnnotation200 = new BitSet(new long[]{0x0000000020000000L});
public static final BitSet FOLLOW_ID_in_addressableAnnotation202 = new BitSet(new long[]{0x4000000008000002L});
public static final BitSet FOLLOW_TRUE_in_addressableAnnotation214 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FALSE_in_addressableAnnotation229 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_STORABLE_in_storableAnnotation282 = new BitSet(new long[]{0x0000000000000000L,0x0000008000000000L});
public static final BitSet FOLLOW_STORAGE_PATH_ADDER_in_storableAnnotation293 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_STRING_in_storableAnnotation306 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_ID_in_storableAnnotation321 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_STORABLE_SEPARATOR_in_storableAnnotation344 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_STRING_in_storableAnnotation348 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_STORABLE_ENCODING_in_storableAnnotation364 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_STRING_in_storableAnnotation368 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_STORABLE_TTL_DAYS_in_storableAnnotation384 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_INT_in_storableAnnotation388 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_STORABLE_PREFIX_in_storableAnnotation404 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_STRING_in_storableAnnotation408 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_STORABLE_REPO_NAME_in_storableAnnotation424 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_storableAnnotation428 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_STORABLE_REPO_NAME_in_storableAnnotation444 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_STRING_in_storableAnnotation448 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_DEPRECATED_in_deprecatedAnnotation487 = new BitSet(new long[]{0x0000000020000000L});
public static final BitSet FOLLOW_ID_in_deprecatedAnnotation489 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DEPRECATED_in_deprecatedAnnotation504 = new BitSet(new long[]{0x1000000000000000L});
public static final BitSet FOLLOW_STRING_in_deprecatedAnnotation506 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INDEXED_in_indexedAnnotation550 = new BitSet(new long[]{0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_INDEX_NAME_in_indexedAnnotation553 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_STRING_in_indexedAnnotation555 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_INDEX_COMPONENT_in_indexedAnnotation570 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_indexedAnnotation572 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_EXTENDS_in_extendsAnnotation618 = new BitSet(new long[]{0x0000400000000000L});
public static final BitSet FOLLOW_PACKAGENAME_in_extendsAnnotation620 = new BitSet(new long[]{0x0000000020000000L});
public static final BitSet FOLLOW_ID_in_extendsAnnotation622 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_NEW_in_fieldConstructor668 = new BitSet(new long[]{0xFFFFFBFFFFFFFFF0L,0xFFFFFFFFFFFFFFFFL,0x0000000000000FFFL});
public static final BitSet FOLLOW_set_in_fieldConstructor684 = new BitSet(new long[]{0xFFFFFBFFFFFFFFF2L,0xFFFFFFFFFFFFFFFFL,0x0000000000000FFFL});
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy