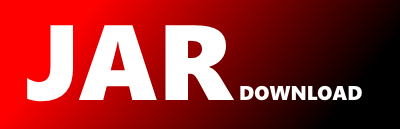
rapture.common.LogMessage Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This file is autogenerated and any changes will be overwritten.
*/
package rapture.common;
import rapture.common.*;
import rapture.object.Searchable;
import rapture.object.Storable;
import rapture.object.Debugable;
import rapture.common.version.ApiVersion;
import rapture.common.impl.jackson.JacksonUtil;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
*
**/
@SuppressWarnings("all")
public class LogMessage implements RaptureTransferObject, Debugable {
/*
* Start of field 'timestamp' (Long)
*/
private Long timestamp;
@JsonProperty("timestamp")
public Long getTimestamp() {
return timestamp;
}
@JsonProperty("timestamp")
public void setTimestamp(Long timestamp) {
this.timestamp = timestamp ;
}
/*
* end of field 'timestamp' (Long)
*/
/*
* Start of field 'host' (String)
*/
private String host;
@JsonProperty("host")
public String getHost() {
return host;
}
@JsonProperty("host")
public void setHost(String host) {
this.host = host ;
}
/*
* end of field 'host' (String)
*/
/*
* Start of field 'level' (String)
*/
private String level;
@JsonProperty("level")
public String getLevel() {
return level;
}
@JsonProperty("level")
public void setLevel(String level) {
this.level = level ;
}
/*
* end of field 'level' (String)
*/
/*
* Start of field 'appName' (String)
*/
private String appName;
@JsonProperty("appName")
public String getAppName() {
return appName;
}
@JsonProperty("appName")
public void setAppName(String appName) {
this.appName = appName ;
}
/*
* end of field 'appName' (String)
*/
/*
* Start of field 'threadName' (String)
*/
private String threadName;
@JsonProperty("threadName")
public String getThreadName() {
return threadName;
}
@JsonProperty("threadName")
public void setThreadName(String threadName) {
this.threadName = threadName ;
}
/*
* end of field 'threadName' (String)
*/
/*
* Start of field 'className' (String)
*/
private String className;
@JsonProperty("className")
public String getClassName() {
return className;
}
@JsonProperty("className")
public void setClassName(String className) {
this.className = className ;
}
/*
* end of field 'className' (String)
*/
/*
* Start of field 'lineNumber' (Integer)
*/
private Integer lineNumber;
@JsonProperty("lineNumber")
public Integer getLineNumber() {
return lineNumber;
}
@JsonProperty("lineNumber")
public void setLineNumber(Integer lineNumber) {
this.lineNumber = lineNumber ;
}
/*
* end of field 'lineNumber' (Integer)
*/
/*
* Start of field 'message' (String)
*/
private String message;
@JsonProperty("message")
public String getMessage() {
return message;
}
@JsonProperty("message")
public void setMessage(String message) {
this.message = message ;
}
/*
* end of field 'message' (String)
*/
/*
* Start of field 'workOrderURI' (String)
*/
private String workOrderURI;
@JsonProperty("workOrderURI")
public String getWorkOrderURI() {
return workOrderURI;
}
@JsonProperty("workOrderURI")
public void setWorkOrderURI(String workOrderURI) {
this.workOrderURI = workOrderURI ;
}
/*
* end of field 'workOrderURI' (String)
*/
/*
* Start of field 'workerId' (String)
*/
private String workerId;
@JsonProperty("workerId")
public String getWorkerId() {
return workerId;
}
@JsonProperty("workerId")
public void setWorkerId(String workerId) {
this.workerId = workerId ;
}
/*
* end of field 'workerId' (String)
*/
/*
* Start of field 'rfxScript' (String)
*/
private String rfxScript;
@JsonProperty("rfxScript")
public String getRfxScript() {
return rfxScript;
}
@JsonProperty("rfxScript")
public void setRfxScript(String rfxScript) {
this.rfxScript = rfxScript ;
}
/*
* end of field 'rfxScript' (String)
*/
/*
* Start of field 'stepName' (String)
*/
private String stepName;
@JsonProperty("stepName")
public String getStepName() {
return stepName;
}
@JsonProperty("stepName")
public void setStepName(String stepName) {
this.stepName = stepName ;
}
/*
* end of field 'stepName' (String)
*/
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((workerId == null) ? 0 : workerId.hashCode());
result = prime * result + ((level == null) ? 0 : level.hashCode());
result = prime * result + ((stepName == null) ? 0 : stepName.hashCode());
result = prime * result + ((appName == null) ? 0 : appName.hashCode());
result = prime * result + ((workOrderURI == null) ? 0 : workOrderURI.hashCode());
result = prime * result + ((host == null) ? 0 : host.hashCode());
result = prime * result + ((rfxScript == null) ? 0 : rfxScript.hashCode());
result = prime * result + ((className == null) ? 0 : className.hashCode());
result = prime * result + ((lineNumber == null) ? 0 : lineNumber.hashCode());
result = prime * result + ((message == null) ? 0 : message.hashCode());
result = prime * result + ((threadName == null) ? 0 : threadName.hashCode());
result = prime * result + ((timestamp == null) ? 0 : timestamp.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (getClass() != obj.getClass()) return false;
LogMessage other = (LogMessage) obj;
if (workerId == null) {
if (other.workerId != null) {
return false;
}
} else if (!workerId.equals(other.workerId)) {
return false;
}
if (level == null) {
if (other.level != null) {
return false;
}
} else if (!level.equals(other.level)) {
return false;
}
if (stepName == null) {
if (other.stepName != null) {
return false;
}
} else if (!stepName.equals(other.stepName)) {
return false;
}
if (appName == null) {
if (other.appName != null) {
return false;
}
} else if (!appName.equals(other.appName)) {
return false;
}
if (workOrderURI == null) {
if (other.workOrderURI != null) {
return false;
}
} else if (!workOrderURI.equals(other.workOrderURI)) {
return false;
}
if (host == null) {
if (other.host != null) {
return false;
}
} else if (!host.equals(other.host)) {
return false;
}
if (rfxScript == null) {
if (other.rfxScript != null) {
return false;
}
} else if (!rfxScript.equals(other.rfxScript)) {
return false;
}
if (className == null) {
if (other.className != null) {
return false;
}
} else if (!className.equals(other.className)) {
return false;
}
if (lineNumber == null) {
if (other.lineNumber != null) {
return false;
}
} else if (!lineNumber.equals(other.lineNumber)) {
return false;
}
if (message == null) {
if (other.message != null) {
return false;
}
} else if (!message.equals(other.message)) {
return false;
}
if (threadName == null) {
if (other.threadName != null) {
return false;
}
} else if (!threadName.equals(other.threadName)) {
return false;
}
if (timestamp == null) {
if (other.timestamp != null) {
return false;
}
} else if (!timestamp.equals(other.timestamp)) {
return false;
}
return true;
}
/**/
public String debug() {
StringBuilder sb = new StringBuilder();
{
sb.append(" workerId= ");
Object o = workerId;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" level= ");
Object o = level;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" stepName= ");
Object o = stepName;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" appName= ");
Object o = appName;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" workOrderURI= ");
Object o = workOrderURI;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" host= ");
Object o = host;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" rfxScript= ");
Object o = rfxScript;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" className= ");
Object o = className;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" lineNumber= ");
Object o = lineNumber;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" message= ");
Object o = message;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" threadName= ");
Object o = threadName;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" timestamp= ");
Object o = timestamp;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
return sb.append("\n").toString();
}
@Override
public String toString() {
return JacksonUtil.jsonFromObject(this);
}
private ApiVersion _raptureVersion;
@JsonProperty("_raptureVersion")
public ApiVersion get_raptureVersion() {
return _raptureVersion;
}
@JsonProperty("_raptureVersion")
public void set_raptureVersion(ApiVersion _raptureVersion) {
this._raptureVersion = _raptureVersion;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy