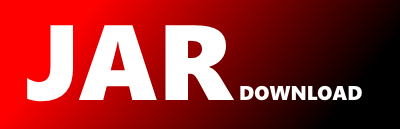
rapture.common.SeriesRepoConfig Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This file is autogenerated and any changes will be overwritten.
*/
package rapture.common;
import rapture.common.*;
import rapture.object.Searchable;
import rapture.object.Storable;
import rapture.object.Debugable;
import rapture.common.version.ApiVersion;
import rapture.common.impl.jackson.JacksonUtil;
import rapture.common.Scheme;
import rapture.object.Addressable;
import rapture.common.model.IndexConfig;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
*
**/
@SuppressWarnings("all")
public class SeriesRepoConfig implements RaptureTransferObject, Debugable ,Storable , Addressable, Searchable {
/*
* Start of field 'description' (String)
*/
private String description;
@JsonProperty("description")
public String getDescription() {
return description;
}
@JsonProperty("description")
public void setDescription(String description) {
this.description = description ;
}
/*
* end of field 'description' (String)
*/
/*
* Start of field 'config' (String)
*/
private String config;
@JsonProperty("config")
public String getConfig() {
return config;
}
@JsonProperty("config")
public void setConfig(String config) {
this.config = config ;
}
/*
* end of field 'config' (String)
*/
/*
* Start of field 'authority' (String)
*/
private String authority;
@JsonProperty("authority")
public String getAuthority() {
return authority;
}
@JsonProperty("authority")
public void setAuthority(String authority) {
this.authority = authority ;
}
/*
* end of field 'authority' (String)
*/
/*
* Start of field 'seriesName' (String)
*/
private String seriesName;
@JsonProperty("seriesName")
public String getSeriesName() {
return seriesName;
}
@JsonProperty("seriesName")
public void setSeriesName(String seriesName) {
this.seriesName = seriesName ;
}
/*
* end of field 'seriesName' (String)
*/
/*
* Start of field 'sampleColumn' (String)
*/
private String sampleColumn;
@JsonProperty("sampleColumn")
public String getSampleColumn() {
return sampleColumn;
}
@JsonProperty("sampleColumn")
public void setSampleColumn(String sampleColumn) {
this.sampleColumn = sampleColumn ;
}
/*
* end of field 'sampleColumn' (String)
*/
//@Override
public RaptureURI getAddressURI() {
return new RaptureURI(getStoragePath(), Scheme.SERIES);
// return new SERIESURI(getStoragePath());
}
public static final Scheme scheme = Scheme.SERIES;
//@Override
public Scheme getScheme() {
return scheme;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((sampleColumn == null) ? 0 : sampleColumn.hashCode());
result = prime * result + ((seriesName == null) ? 0 : seriesName.hashCode());
result = prime * result + ((authority == null) ? 0 : authority.hashCode());
result = prime * result + ((description == null) ? 0 : description.hashCode());
result = prime * result + ((config == null) ? 0 : config.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (getClass() != obj.getClass()) return false;
SeriesRepoConfig other = (SeriesRepoConfig) obj;
if (sampleColumn == null) {
if (other.sampleColumn != null) {
return false;
}
} else if (!sampleColumn.equals(other.sampleColumn)) {
return false;
}
if (seriesName == null) {
if (other.seriesName != null) {
return false;
}
} else if (!seriesName.equals(other.seriesName)) {
return false;
}
if (authority == null) {
if (other.authority != null) {
return false;
}
} else if (!authority.equals(other.authority)) {
return false;
}
if (description == null) {
if (other.description != null) {
return false;
}
} else if (!description.equals(other.description)) {
return false;
}
if (config == null) {
if (other.config != null) {
return false;
}
} else if (!config.equals(other.config)) {
return false;
}
return true;
}
/**/
public String debug() {
StringBuilder sb = new StringBuilder();
{
sb.append(" sampleColumn= ");
Object o = sampleColumn;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" seriesName= ");
Object o = seriesName;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" authority= ");
Object o = authority;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" description= ");
Object o = description;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
{
sb.append(" config= ");
Object o = config;
if (o != null) {
if (o instanceof java.util.Collection) {
sb.append("{ ");
for (Object oo : (java.util.Collection>) o) {
if (oo == null) sb.append("null");
else if (oo instanceof Debugable) sb.append(((Debugable) oo).debug());
else sb.append(oo.toString()).append(", ");
}
sb.append(" } ");
} else {
if (o instanceof Debugable) sb.append(((Debugable) o).debug());
else sb.append(o.toString());
}
}
}
return sb.append("\n").toString();
}
@Override
public String getStoragePath() {
// Add key fields
return new SeriesRepoConfigPathBuilder()
.authority(getAuthority())
.buildStoragePath();
}
@Override
public RaptureURI getStorageLocation() {
return new SeriesRepoConfigPathBuilder()
.authority(getAuthority())
.buildStorageLocation();
}
private Boolean ftsIndex = true;
private String ftsIndexRepo;
@Override
public Boolean getFtsIndex() {
return ftsIndex;
}
@Override
public void setFtsIndex(boolean ftsIndex) {
this.ftsIndex = ftsIndex;
}
@Override
public String getFtsIndexRepo() {
return ftsIndexRepo;
}
@Override
public void setFtsIndexRepo(String ftsIndexRepo) {
this.ftsIndexRepo = ftsIndexRepo;
}
@Override
public String toString() {
return JacksonUtil.jsonFromObject(this);
}
private ApiVersion _raptureVersion;
@JsonProperty("_raptureVersion")
public ApiVersion get_raptureVersion() {
return _raptureVersion;
}
@JsonProperty("_raptureVersion")
public void set_raptureVersion(ApiVersion _raptureVersion) {
this._raptureVersion = _raptureVersion;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy