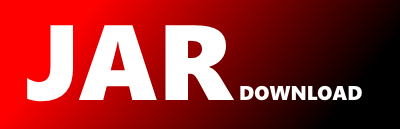
rapture.common.api.AdminApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.common.api;
import rapture.common.exception.RaptureException;
import rapture.common.CallingContext;
import java.util.List;
import java.util.Map;
import rapture.common.TypeArchiveConfig;
import rapture.common.model.RepoConfig;
import rapture.common.CallingContext;
import rapture.common.model.RaptureUser;
@SuppressWarnings("all")
public interface AdminApi {
/**
* This function retrieves the system properties in use for this instance of Rapture.
* As system properties are often used to control external connectivity, a client
* can determine the inferred connectivity endpoints by using this api call. It returns
* a map from system property name (the key) to value. You cannot modify the system
* properties of Rapture through the api, they are set by the administrator as part
* of the general setup of a Rapture system. Note that this call returns the properties
* for the actual end point that the client is connected to, it is not necessarily
* true that the same properties will be set for every API endpoint.
*
*/
Map getSystemProperties(CallingContext context, List keys);
/**
* Rapture is a hierarchical set of repositories, and this method returns the config
* of the top most level - that used for general config and temporary (transient)
* values such as sessions. In clustered mode these configs would be referencing shared
* storage, and in test mode they would normally refer to in-memory versions of the
* config. The manipulation of the top level config can be performed through the
* Bootstrap API.
*
*/
List getRepoConfig(CallingContext context);
/**
* When a user logs into Rapture they create a transient session and this method is
* a way of retrieving all of the sessions for a given user. The CallingContext is
* a common object passed around Rapture api calls.
*
*/
List getSessionsForUser(CallingContext context, String user);
/**
* This method removes a user from this Rapture system. The user is removed from all
* entitlement groups also. The actual user definition is retained and marked as inactive
* (so the user cannot login). This is because the user may still be referenced in
* audit trails and the change history in type repositories.
*
*/
void deleteUser(CallingContext context, String userName);
/**
* This method destroys a user record. The user must have been previously disabled using
* 'deleteUser' before this method may be called. This is a severe method that should
* only be used in non-production machines or to correct an administrative error in
* creating an account with the wrong name before that account has been used. Reference
* to the missing user may still exist, and may not display properly in some UIs
*
*/
void destroyUser(CallingContext context, String userName);
/**
* This method restores a user that has been deleted
*
*/
void restoreUser(CallingContext context, String userName);
/**
* This method adds a user to the Rapture environment. The user will be in no entitlement
* groups by default. The password field passed is actually the MD5 hash of the password
* - or at least the same hash function that will be applied when logging in to the
* system (the password is hashed, and then hashed again with the salt returned during
* the login protocol).
*
*/
void addUser(CallingContext context, String userName, String description, String hashPassword, String email);
/**
* Verify user by providing a secret token
*
*/
Boolean verifyUser(CallingContext context, String userName, String token);
/**
* This api call can be used to determine whether a given user exists in the Rapture
* system. Only system administrators can use this api call.
*
*/
Boolean doesUserExist(CallingContext context, String userName);
/**
* Retrieve a single user given their name.
*
*/
RaptureUser getUser(CallingContext context, String userName);
/**
* Generates an api user, for use in connecting to Rapture in a relatively opaque way
* using a shared secret. An api user can log in with their access key without a password.
*
*/
RaptureUser generateApiUser(CallingContext context, String prefix, String description);
/**
* This method gives an administrator the ability to reset the password of a user. The
* user will have the new password passed. The newHashPassword parameter should be
* an MD5 of the new password - internally this will be hashed further against a
* salt for this user.
*
*/
void resetUserPassword(CallingContext context, String userName, String newHashPassword);
/**
* Creates password reset token and emails it to the user
*
*/
String createPasswordResetToken(CallingContext context, String username);
/**
* Creates registration token and emails it to the user
*
*/
String createRegistrationToken(CallingContext context, String username);
/**
* Cancels password reset token
*
*/
void cancelPasswordResetToken(CallingContext context, String username);
/**
* Emails user
*
*/
void emailUser(CallingContext context, String userName, String emailTemplate, Map templateValues);
/**
* This method updates user email.
*
*/
void updateUserEmail(CallingContext context, String userName, String newEmail);
/**
* This function adds a template to the Rapture system. A template is a simple way of
* registering predefined configs that can be used to automatically generate configs
* for repositories, queues, and the like. Templates use the popular StringTemplate
* library for merging values into a text template.
*
*/
void addTemplate(CallingContext context, String name, String template, Boolean overwrite);
/**
* This method executes a template, replacing parts of the template with the passed
* parameters to create a new string.
*
*/
String runTemplate(CallingContext context, String name, String parameters);
/**
* This method returns the definition of a template.
*
*/
String getTemplate(CallingContext context, String name);
/**
* Copies the data from one DocumentRepo to another. The target repository is wiped
* out before hand if 'wipe' is set to true. The target must already exist when this
* method is called.
*
*/
void copyDocumentRepo(CallingContext context, String srcAuthority, String targAuthority, Boolean wipe);
/**
* Use this method to add an IP address to a white list of allowed IP addresses that
* can log in to this Rapture environment. Once set only IP addresses in this ipAddress
* list can access Rapture. By default there are no whitelist IP addresses defined
* which actually means that all IP addresses are allowed.
*
*/
void addIPToWhiteList(CallingContext context, String ipAddress);
/**
* Use this method to remove an IP address from a white list
*
*/
void removeIPFromWhiteList(CallingContext context, String ipAddress);
/**
* Use this method to return the IP white list
*
*/
List getIPWhiteList(CallingContext context);
/**
* This method retrieves all of the registered users in the system
*
*/
List getAllUsers(CallingContext context);
/**
* This method kicks off a process that will migrate a DocumentRepo to an alternate
* config. A temporary type will be created with the new config, the old type will
* be locked for modifications and then all of the documents in the existing type will
* be copied to the new type, with the metadata intact. Optionally a number of historical
* versions will be kept if the source repository (and target) support it. Once all
* of the data has been copied the config attached to each type will be swapped and
* the type released for access. The temporary type will then be dropped.
*
*/
void initiateTypeConversion(CallingContext context, String raptureURI, String newConfig, int versionsToKeep);
/**
* Set the archive config for a type
*
*/
void putArchiveConfig(CallingContext context, String raptureURI, TypeArchiveConfig config);
/**
* Retrieve the archive config for a authority
*
*/
TypeArchiveConfig getArchiveConfig(CallingContext context, String raptureURI);
/**
* Delete the archive config for a authority
*
*/
void deleteArchiveConfig(CallingContext context, String raptureURI);
/**
* A general purpose function that tests (or refreshes) the api connection to Rapture
* with no side effects.
*
*/
Boolean ping(CallingContext context);
/**
* This function adds values to the metadata field of the CallingContext. It's used
* to hold values specific to this connection. Since it's set by the caller the values
* cannot be considered entirely trusted, and private or secure data such as passwords
* shouldn't be stored in here. If overwrite is false and an entry already exists then
* an exception should be thrown
*
*/
void addMetadata(CallingContext context, Map values, Boolean overwrite);
/**
* Set the MOTD (message of the day) for this environment. Setting to a zero length
* string implies that there is no message of the day
*
*/
void setMOTD(CallingContext context, String message);
/**
* Retrieve the MOTD
*
*/
String getMOTD(CallingContext context);
/**
* Set the name of this environment
*
*/
void setEnvironmentName(CallingContext context, String name);
/**
* Set the properties of this environment. Usually for displaying then name (e.g. BANNER_COLOR)
*
*/
void setEnvironmentProperties(CallingContext context, Map properties);
/**
* Get the name of this environment
*
*/
String getEnvironmentName(CallingContext context);
/**
* Get the properties of this environment
*
*/
Map getEnvironmentProperties(CallingContext context);
/**
* Encode a String using the default encoding mechanism
*
*/
String encode(CallingContext context, String toEncode);
/**
* Create a URI with proper encoding given a path and a leaf. Normal URI characters
* such as : or / in the path will not be encoded
*
*/
String createURI(CallingContext context, String path, String leaf);
/**
* Create a URI with proper encoding given a list of elements. The return value will
* begin with // Each element will be encoded (including all punctuation characters)
* and the elements joined together separated by /
*
*/
String createMultipartURI(CallingContext context, List elements);
/**
* Decode the supplied String according to the URI encoding/decoding rules
*
*/
String decode(CallingContext context, String encoded);
/**
* Find the groups for a given user and return just the names
*
*/
List findGroupNamesByUser(CallingContext context, String username);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy