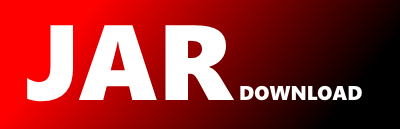
rapture.common.api.AuditApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.common.api;
import rapture.common.exception.RaptureException;
import rapture.common.CallingContext;
import java.util.List;
import java.util.Map;
import rapture.common.model.AuditLogEntry;
import rapture.common.RaptureFolderInfo;
import rapture.common.AuditLogConfig;
@SuppressWarnings("all")
public interface AuditApi {
/**
* Sets up anything needed for audit to run properly. This should be called from the
* _startup.rfx script. This call is used internally by Rapture on startup, and is
* normally called only for debugging purposes.
*
*/
void setup(CallingContext context, Boolean force);
/**
* This method creates a new audit log, given a name and a config string. The config
* string defines the implementation to be used to store the audit entries.
*
*/
void createAuditLog(CallingContext context, String name, String config);
/**
* This method checks whether an audit log exists at the specified URI. The log must
* have been created using createAuditLog.
*
*/
Boolean doesAuditLogExist(CallingContext context, String logURI);
/**
* This method searches for audit logs whose name follows the pattern prefix/anything_else/under/here,
* where prefix is the argument that is passed in.
*
*/
List getChildren(CallingContext context, String prefix);
/**
* This method removes a previously created audit log.
*
*/
void deleteAuditLog(CallingContext context, String logURI);
/**
* This method retrieves the config information for a previously created audit log.
*
*/
AuditLogConfig getAuditLog(CallingContext context, String logURI);
/**
* This method writes an audit entry to the audit log specified by the URI parameter.
*
*/
void writeAuditEntry(CallingContext context, String logURI, String category, int level, String message);
/**
* This method writes an audit entry to an audit log.
*
*/
void writeAuditEntryData(CallingContext context, String logURI, String category, int level, String message, Map data);
/**
* This method retrieves previously registered log entries, given a maximum number of
* entries to return.
*
*/
List getRecentLogEntries(CallingContext context, String logURI, int count);
/**
* This method retrieves any entries since a given entry was retrieved. The date of
* this audit entry is used to determine the start point of the query.
*
*/
List getEntriesSince(CallingContext context, String logURI, AuditLogEntry when);
/**
* This method retrieves all of the recent API call activity (including login) performed
* by a user, given a maximum number of entries to return. A 'count' argument of less
* than 0 will return everything available.
*
*/
List getRecentUserActivity(CallingContext context, String user, int count);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy