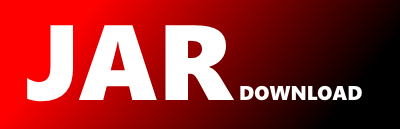
rapture.common.api.DecisionApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.common.api;
import rapture.common.exception.RaptureException;
import rapture.common.CallingContext;
import java.util.List;
import java.util.Map;
import rapture.common.AppStatus;
import rapture.common.CreateResponse;
import rapture.common.RaptureFolderInfo;
import rapture.common.dp.WorkOrderStatus;
import rapture.common.dp.Workflow;
import rapture.common.dp.Step;
import rapture.common.dp.WorkOrderCancellation;
import rapture.common.LogQueryResponse;
import rapture.common.dp.WorkOrderDebug;
import rapture.common.dp.WorkOrder;
import rapture.common.dp.AppStatusDetails;
import rapture.common.dp.Transition;
import rapture.common.dp.Worker;
import rapture.common.dp.WorkflowHistoricalMetrics;
import rapture.common.ErrorWrapper;
@SuppressWarnings("all")
public interface DecisionApi {
/**
* Returns all workflow definitions
*
*/
List getAllWorkflows(CallingContext context);
/**
* Returns a list of full display names of the paths below this one. Ideally optimized
* depending on the repo.
*
*/
List getWorkflowChildren(CallingContext context, String workflowURI);
/**
* Return a list of full display names of the paths below this one. Ideally optimized
* depending on the repo.
*
*/
List getWorkOrderChildren(CallingContext context, String parentPath);
/**
* Create or update a workflow to contain only the specified nodes and transitions.
*
*/
void putWorkflow(CallingContext context, Workflow workflow);
/**
* Returns a workflow definition, or null if not found.
*
*/
Workflow getWorkflow(CallingContext context, String workflowURI);
/**
* Returns a step definition, or null if not found
*
*/
Step getWorkflowStep(CallingContext context, String stepURI);
/**
* Gets the category associated with a step. This is the step's own categoryOverride,
* if present, or otherwise the category associated with the entire workflow.
*
*/
String getStepCategory(CallingContext context, String stepURI);
/**
* Adds a new step to an existing workflow initially containing the specified transitions
*
*/
void addStep(CallingContext context, String workflowURI, Step step);
/**
* Removes a step from a workflow.
*
*/
void removeStep(CallingContext context, String workflowURI, String stepName);
/**
* Adds a new Transition to a workflow.
*
*/
void addTransition(CallingContext context, String workflowURI, String stepName, Transition transition);
/**
* Removes a transition from a workflow.
*
*/
void removeTransition(CallingContext context, String workflowURI, String stepName, String transitionName);
/**
* Deletes a workflow.
*
*/
void deleteWorkflow(CallingContext context, String workflowURI);
/**
* Creates and executes a workflow. If there is a defaultAppStatusUriPattern set for
* this Workflow then it will be used for the appstatus URI. Otherwise, no appstatus
* will be created. TODO make workOrderURI format align with permission checks.
*
*/
String createWorkOrder(CallingContext context, String workflowURI, Map argsMap);
/**
* Creates and executes a workflow. Same as createWorkOrder, but the appStatusUriPattern
* is passed as an explicit argument instead of using the default appStatusUriPattern
* (if one has been set). Note that the app status allows the Workflow and its output
* to be accessed via the web interface; workflows without an app status are not accessible
* in this way.
*
*/
CreateResponse createWorkOrderP(CallingContext context, String workflowURI, Map argsMap, String appStatusUriPattern);
/**
* Releases the lock associated with this WorkOrder. This method should only be used
* by admins, in case there was an unexpected problem that caused a WorkOrder to finish
* or die without releasing the lock.
*
*/
void releaseWorkOrderLock(CallingContext context, String workOrderURI);
/**
* Gets the status of a workOrder
*
*/
WorkOrderStatus getWorkOrderStatus(CallingContext context, String workOrderURI);
/**
* Writes an audit entry related to a workOrder. Messages may be INFO or ERROR based
* on the boolean fourth parameter
*
*/
void writeWorkflowAuditEntry(CallingContext context, String workOrderURI, String message, Boolean error);
/**
* Gets the WorkOrder objects starting on a given day. Orders that carried over from
* the previous day are not included.
*
*/
List getWorkOrdersByDay(CallingContext context, Long startTimeInstant);
/**
* Gets all the workorder URIs associated with the given workflow uri, from a starting
* point given in Unix epoch time. Passing in 0 or null as the start time will get
* all workorders from the beginning of time.
*
*/
List getWorkOrdersByWorkflow(CallingContext context, Long startTimeInstant, String workflowUri);
/**
* Gets the top-level status object associated with the work order
*
*/
WorkOrder getWorkOrder(CallingContext context, String workOrderURI);
/**
* Get the worker
*
*/
Worker getWorker(CallingContext context, String workOrderURI, String workerId);
/**
* Requests cancellation of a work order. This method returns immediately once the cancellation
* is recorded, but the individual workers may continue for some time before stopping,
* depending on the type of step being executed.
*
*/
void cancelWorkOrder(CallingContext context, String workOrderURI);
/**
* Resume work order
*
*/
CreateResponse resumeWorkOrder(CallingContext context, String workOrderURI, String resumeStepURI);
/**
* Returns true if CancelWorkOrder was called.
*
*/
Boolean wasCancelCalled(CallingContext context, String workOrderURI);
/**
* Gets details for the cancellation for a workOrder -- or null if not cancelled.
*
*/
WorkOrderCancellation getCancellationDetails(CallingContext context, String workOrderURI);
/**
* Gets the detailed context information for a work order in progress
*
*/
WorkOrderDebug getWorkOrderDebug(CallingContext context, String workOrderURI);
/**
* Defines the IdGen config for work order items.
*
*/
void setWorkOrderIdGenConfig(CallingContext context, String config, Boolean force);
/**
* Sets a literal in the context. The literal value that is stored will be returned
* after a read. The workerURI is a workOrderURI with the element set to the worker
* ID.
*
*/
void setContextLiteral(CallingContext context, String workerURI, String varAlias, String literalValue);
/**
* Set a literal in the context. This means that whatever is stored will be evaluated
* before being returned, so it must be a valid expression. The workerURI is a workOrderURI
* with the element set to the worker id
*
*/
void setContextLink(CallingContext context, String workerURI, String varAlias, String expressionValue);
/**
* Gets a value in the context, as json. The workerURI is a workOrderURI with the element
* set to the worker id.
*
*/
String getContextValue(CallingContext context, String workerURI, String varAlias);
/**
* Adds an error to the context of a particular worker. The workerURI is a workOrderURI
* with the element set to the worker id
*
*/
void addErrorToContext(CallingContext context, String workerURI, ErrorWrapper errorWrapper);
/**
* Gets the errors from the context for a given worker. The workerURI is a workOrderURI
* with the element set to the worker id.
*
*/
List getErrorsFromContext(CallingContext context, String workerURI);
/**
* Get info about any exception(s) thrown during execution of this workorder
*
*/
List getExceptionInfo(CallingContext context, String workOrderURI);
/**
* Report status of the step - workerURI: the uri of this WorkOrder with the element
* set to the worker ID - stepStartTime: the time when the step being reported started
* - message: a human-friendly message to display - progress: how many units are
* currently complete - max: how many units in total would mark this as done
*
*/
void reportStepProgress(CallingContext context, String workerURI, Long stepStartTime, String message, Long progress, Long max);
/**
* Gets app statuses by prefix.
*
*/
List getAppStatuses(CallingContext context, String prefix);
/**
* Gets detailed app status info by prefix. Also returns any context values requested
* in the second argument.
*
*/
List getAppStatusDetails(CallingContext context, String prefix, List extraContextValues);
/**
* Get any defined average runtimes for the past month for a workflow
*
*/
WorkflowHistoricalMetrics getMonthlyMetrics(CallingContext context, String workflowURI, String jobURI, String argsHashValue, String state);
/**
* Get log messages for a workflow. Note: logs get deleted after a certain number of
* days, so this only retrieves any log messages that are within the configured log
* retention period. If the retention period is before the startTime, an empty response
* is returned. workOrderURI: required startTime: required endTime: required keepAlive:
* required, milliseconds to keep alive the batch, max 30000 bufferSize: required,
* max 100 nextBatchId: optional, if null start from beginning stepName: optional
* stepStartTime: optional, this is a timestamp in a string because of a limitation
* in Rapture where int or long arguments cannot be null
*
*/
LogQueryResponse queryLogs(CallingContext context, String workOrderURI, Long startTime, Long endTime, Long keepAlive, Long bufferSize, String nextBatchId, String stepName, String stepStartTime);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy