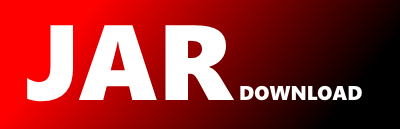
rapture.common.api.DocApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.common.api;
import rapture.common.exception.RaptureException;
import rapture.common.CallingContext;
import java.util.List;
import java.util.Map;
import rapture.common.model.DocumentRepoConfig;
import rapture.common.model.DocumentMetadata;
import rapture.common.RaptureFolderInfo;
import rapture.common.RaptureIdGenConfig;
import rapture.common.model.DocumentWithMeta;
import rapture.common.XferDocumentAttribute;
import rapture.common.model.DocWriteHandle;
@SuppressWarnings("all")
public interface DocApi {
/**
* Validates repository; requires write permission because it can cause files/tables
* to be created on first use.
*
*/
Boolean validateDocRepo(CallingContext context, String docRepoUri);
/**
* A DocumentRepository is used to store JSON docs. This method creates and configures
* the repository for an authority.
*
*/
void createDocRepo(CallingContext context, String docRepoUri, String config);
/**
* This API call can be used to determine whether a given repository exists.
*
*/
Boolean docRepoExists(CallingContext context, String docRepoUri);
/**
* This api call can be used to determine whether a given type exists in a given authority.
*
*/
Boolean docExists(CallingContext context, String docUri);
/**
* Retrieves the configuration string for the given document repository.
*
*/
DocumentRepoConfig getDocRepoConfig(CallingContext context, String docRepoUri);
/**
* Gets any available information about a repository.
*
*/
Map getDocRepoStatus(CallingContext context, String docRepoUri);
/**
* Retrieves document repositories
*
*/
List getDocRepoConfigs(CallingContext context);
/**
* This method removes a documentRepository and its data from the Rapture system. There
* is no undo.
*
*/
void deleteDocRepo(CallingContext context, String docRepoUri);
/**
* This method archives older versions of a documentRepository.
*
*/
void archiveRepoDocs(CallingContext context, String docRepoUri, int versionLimit, long timeLimit, Boolean ensureVersionLimit);
/**
* Retrieves the content and the meta data associated with a document, including version
* and user information. If the storage does not support metadata, this method returns
* a dummy object.
*
*/
DocumentWithMeta getDocAndMeta(CallingContext context, String docUri);
/**
* Retrieves only the meta data associated with a document, including version and user
* information. If the storage does not support metadata, this method returns a dummy
* object.
*
*/
DocumentMetadata getDocMeta(CallingContext context, String docUri);
/**
* Reverts this document back to the previous version by taking the previous version
* and making a new version.
*
*/
DocumentWithMeta revertDoc(CallingContext context, String docUri);
/**
* Retrieves the content of a document.
* @params docUri a string of characters used to identify a document
*
*/
String getDoc(CallingContext context, String docUri);
/**
* Stores a document in the Rapture system.
* @params docUri a string of characters used to identify a document
* @params content the information to be stored within the document
* @since 1.0.0
*
*/
String putDoc(CallingContext context, String docUri, String content);
/**
* Attempts to put the content into the repository, but fails if the repository supports
* versioning and the current version of the document stored does not match the version
* passed. A version of zero implies that the document should not exist. The purpose
* of this call is for a client to be able to call getDocAndMeta to retrieve an existing
* document, modify it, and save the content back, using the version number in the
* metadata of the document. If another client has modified the data since it was loaded,
* this call will return false, indicating that the save was not possible.
*
*/
Boolean putDocWithVersion(CallingContext context, String docUri, String content, int currentVersion);
/**
* Store a document in the Rapture system, passing in an event context to be added to
* any events spawned off by this put. Parts of the uri could be automatically generated
* @return A DocWriteHandle object that contains a value on whether the write was successful, the uri of the document that was written, and a handle to the event uri that was fired, if any
*
*/
DocWriteHandle putDocWithEventContext(CallingContext context, String docUri, String content, Map eventContext);
/**
* Removes a document attribute. Can be used to remove all attributes for a given type
* as well if the key argument is null.
*
*/
Boolean deleteDoc(CallingContext context, String docUri);
/**
* Renames a document, by getting and putting it on the system without transferring
* the data back to the client.
*
*/
String renameDoc(CallingContext context, String fromDocUri, String toDocUri);
/**
* Returns a map of contents where the key is the normalized input Uri and the value
* is the document retrieved
*
*/
Map getDocs(CallingContext context, List docUris);
/**
* Returns a list of documents and their associated meta data - the meta data includes
* version and user information
*
*/
List getDocAndMetas(CallingContext context, List docUris);
/**
* Returns a list of true/false statements on whether the listed Uris refer to a valid
* document. Note: a folder is not considered to be a document.
*
*/
List docsExist(CallingContext context, List docUris);
/**
* Put a series of documents in a batch form. Refer to putDoc for details.
*
*/
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy