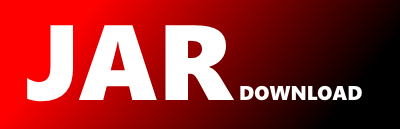
rapture.common.api.PluginApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.common.api;
import rapture.common.exception.RaptureException;
import rapture.common.CallingContext;
import java.util.List;
import java.util.Map;
import rapture.common.PluginConfig;
import rapture.common.PluginTransportItem;
import rapture.common.PluginManifest;
@SuppressWarnings("all")
public interface PluginApi {
/**
* Lists plugins in the system.
*
*/
List getInstalledPlugins(CallingContext context);
/**
* Retrieves the manifest for a plugin.
*
*/
PluginManifest getPluginManifest(CallingContext context, String manifestUri);
/**
* Retrieves the manifest for a plugin.
*
*/
void recordPlugin(CallingContext context, PluginConfig plugin);
/**
* Installs the plugin and updates the registry.
*
*/
void installPlugin(CallingContext context, PluginManifest manifest, Map payload);
/**
* Installs a single item from a plugin to allow clients to operate in a low-memory
* environment
*
*/
void installPluginItem(CallingContext context, String pluginName, PluginTransportItem item);
/**
* Removes a plugin.
*
*/
void uninstallPlugin(CallingContext context, String name);
/**
* Removes an item from a plugin.
*
*/
void uninstallPluginItem(CallingContext context, PluginTransportItem item);
/**
* Removes plugin Manifest but does not uninstall any referenced items.
*
*/
void deletePluginManifest(CallingContext context, String manifestUri);
/**
* Gets the encoding for a Rapture object given its URI.
*
*/
PluginTransportItem getPluginItem(CallingContext context, String uri);
/**
* Verifies that the contents of a plugin match the hashes in the manifest.
*
*/
Map verifyPlugin(CallingContext context, String plugin);
/**
* create an empty manifest on the server with version 0.0.0
*
*/
void createManifest(CallingContext context, String pluginName);
/**
* add an object on the server to a plugin manifest on the server
*
*/
void addManifestItem(CallingContext context, String pluginName, String uri);
/**
* add uris within the specified docpath root. If no type is specifed in the uri, use
* all four of doc, blob, series, and sheet. Example1: //myProject/myfolder adds all
* four types. Example2: blob://myproject/myfolder adds only blobs
*
*/
void addManifestDataFolder(CallingContext context, String pluginName, String uri);
/**
* remove uris within the specified path. If no type is specifed in the uri, use all
* four of doc, blob, series, and sheet.
*
*/
void removeManifestDataFolder(CallingContext context, String pluginName, String uri);
/**
* refresh the MD5 checksums in the manifest and set the version for a manifest on the
* server
*
*/
void setManifestVersion(CallingContext context, String pluginName, String version);
/**
* remove an item from the manifest of a plugin on the server
*
*/
void removeItemFromManifest(CallingContext context, String pluginName, String uri);
/**
* Export a plugin as a single blob. We pass in a parent path; the blob will be generated
* somewhere under that path, in a non-predictable location. The location is returned.
*
*/
String exportPlugin(CallingContext context, String pluginName, String path);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy