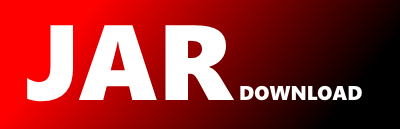
rapture.common.api.RunnerApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.common.api;
import rapture.common.exception.RaptureException;
import rapture.common.CallingContext;
import java.util.List;
import java.util.Map;
import rapture.common.RaptureLibraryDefinition;
import rapture.common.RaptureRunnerConfig;
import rapture.common.RaptureRunnerStatus;
import rapture.common.RaptureApplicationDefinition;
import rapture.common.RaptureServerGroup;
import rapture.common.RaptureInstanceCapabilities;
import rapture.common.model.RaptureApplicationStatus;
import rapture.common.RaptureApplicationInstance;
import rapture.common.model.RaptureApplicationStatusStep;
@SuppressWarnings("all")
public interface RunnerApi {
/**
* Creates a new server group.
*
*/
RaptureServerGroup createServerGroup(CallingContext context, String name, String description);
/**
* Remove a server group (and all of its application definitions )
*
*/
void deleteServerGroup(CallingContext context, String name);
/**
* Returns all server groups defined in Rapture.
*
*/
List getAllServerGroups(CallingContext context);
/**
* Returns a list of all the applications defined in Rapture, which Rapture Runner knows
* about, including their versions. This is the list of applications that Rapture is
* aware of, but it does not necessarily run everything. To get a list of what will
* be running, look at getAllApplicationInstances.
*
*/
List getAllApplicationDefinitions(CallingContext context);
/**
* Get a list of all libraries defined in Rapture. These are also known as Rapture add-ons,
* or plugins.
*
*/
List getAllLibraryDefinitions(CallingContext context);
/**
* Retrieves all the application instances defined in Rapture. This is really the list
* of schedule entries, meaning every application-server group combination that is
* scheduled to run.
*
*/
List getAllApplicationInstances(CallingContext context);
/**
* Retrieves a server group object, or null if no such object was found.
*
*/
RaptureServerGroup getServerGroup(CallingContext context, String name);
/**
* Add a server group inclusion. An inclusion is a hostname where this server group
* should run. By default, this is set to *, which means run everywhere. Adding an
* inclusion makes it so that this server group will run only on certain servers.
*
*/
RaptureServerGroup addGroupInclusion(CallingContext context, String name, String inclusion);
/**
* Removes a server group inclusion. Refer to AddGroupInclusion for more details.
*
*/
RaptureServerGroup removeGroupInclusion(CallingContext context, String name, String inclusion);
/**
* Add a server group exclusion. An exclusion is a hostname where this server group
* should not run. By default, this is set to empty, which means run on every host
* specified in inclusions. It makes more sense to add an exclusion if this server
* group has a wildcard (*) for inclusions. See also addGroupInclusion.
*
*/
RaptureServerGroup addGroupExclusion(CallingContext context, String name, String exclusion);
/**
* Removes a server group exclusion. Refer to AddGroupExclusion for more details.
*
*/
RaptureServerGroup removeGroupExclusion(CallingContext context, String name, String exclusion);
/**
* Remove an entry from either an exclusion or inclusion
*
*/
RaptureServerGroup removeGroupEntry(CallingContext context, String name, String entry);
/**
* Creates an application definition.
*
*/
RaptureApplicationDefinition createApplicationDefinition(CallingContext context, String name, String ver, String description);
/**
* Delete an application definition (and any references in server groups)
*
*/
void deleteApplicationDefinition(CallingContext context, String name);
/**
* Update a version of an application
*
*/
RaptureApplicationDefinition updateApplicationVersion(CallingContext context, String name, String ver);
/**
* Creates an application library. See also getAllLibraryDefinitions.
*
*/
RaptureLibraryDefinition createLibraryDefinition(CallingContext context, String name, String ver, String description);
/**
* Remove a library definition (and any references in server groups)
*
*/
void deleteLibraryDefinition(CallingContext context, String name);
/**
* Retrieve an library definition
*
*/
RaptureLibraryDefinition getLibraryDefinition(CallingContext context, String name);
/**
* Update a version of a library
*
*/
RaptureLibraryDefinition updateLibraryVersion(CallingContext context, String name, String ver);
/**
* Associates a library with a server group.
*
*/
RaptureServerGroup addLibraryToGroup(CallingContext context, String serverGroup, String libraryName);
/**
* Remove the association between a library and a server group
*
*/
RaptureServerGroup removeLibraryFromGroup(CallingContext context, String serverGroup, String libraryName);
/**
* Adds an association between an application and a server group. This is the way to
* tell Rapture that a certain application needs to run (or be scheduled to run at
* given hours) as part of a server group.
*
*/
RaptureApplicationInstance createApplicationInstance(CallingContext context, String name, String description, String serverGroup, String appName, String timeRange, int retryCount, String parameters, String apiUser);
/**
* Start a batch/single process (ultimately to replace the oneshot calls).
*
*/
RaptureApplicationStatus runApplication(CallingContext context, String appName, String queueName, Map parameterInput, Map parameterOutput);
/**
* Start a batch/single process (ultimately to replace the oneshot calls)s.
*
*/
RaptureApplicationStatus runCustomApplication(CallingContext context, String appName, String queueName, Map parameterInput, Map parameterOutput, String customApplicationPath);
/**
* Returns a status object that shows the current state of the app.
*
*/
RaptureApplicationStatus getApplicationStatus(CallingContext context, String applicationStatusURI);
/**
* Lists the apps that are interesting, given a QBE template (empty strings have default
* behavior).
*
*/
List getApplicationStatuses(CallingContext context, String date);
/**
* Lists the dates for which statuses exist.
*
*/
List getApplicationStatusDates(CallingContext context);
/**
* Tidy up old status invocations
*
*/
void archiveApplicationStatuses(CallingContext context);
/**
* Update the status of an application instance.
*
*/
RaptureApplicationStatus changeApplicationStatus(CallingContext context, String applicationStatusURI, RaptureApplicationStatusStep statusCode, String message);
/**
* Adds messages to a running application instance.
*
*/
void recordStatusMessages(CallingContext context, String applicationStatusURI, List messages);
/**
* Attempts to cancel the execution of an application.
*
*/
RaptureApplicationStatus terminateApplication(CallingContext context, String applicationStatusURI, String reasonMessage);
/**
* Delete an application instance
*
*/
void deleteApplicationInstance(CallingContext context, String name, String serverGroup);
/**
* Retrieve an application instance
*
*/
RaptureApplicationInstance getApplicationInstance(CallingContext context, String name, String serverGroup);
/**
* Update the status of a one shot execution, potentially marking it as finished
*
*/
void updateStatus(CallingContext context, String name, String serverGroup, String myServer, String status, Boolean finished);
/**
* Returns a list of application instance (aka schedule) names that are configured to
* run as part of a specific server group.
*
*/
List getApplicationsForServerGroup(CallingContext context, String serverGroup);
/**
* Returns a list of applications that should run on a specific host (aka server). Servers
* are defined in inclusions; see addGroupInclusion for more details. All applications
* that will run on a given server will be returned. Applications belonging to a server
* group that includes all servers via the * wildcard will also be returned.
*
*/
List getApplicationsForServer(CallingContext context, String serverName);
/**
* Retrieve an application definition
*
*/
RaptureApplicationDefinition getApplicationDefinition(CallingContext context, String name);
/**
* Set a config variable available in RaptureRunner. The config variables understood
* are APPSOURCE and MODSOURCE, and they specify the location of the apps and libraries
* controlled by RaptureRunner.
*
*/
void setRunnerConfig(CallingContext context, String name, String value);
/**
* Removes a variable from the Runner config.
*
*/
void deleteRunnerConfig(CallingContext context, String name);
/**
* Returns the RaptureRunnerConfig object, which contains the values of the variables
* configured via setRunnerConfig.
*
*/
RaptureRunnerConfig getRunnerConfig(CallingContext context);
/**
* Records the status of an application instance by acquiring a lock based on the server
* name, similar to the behavior of cleanRunnerStatus and markForRestart.
*
*/
void recordRunnerStatus(CallingContext context, String serverName, String serverGroup, String appInstance, String appName, String status);
/**
* Each RaptureApplicationInstance has certain capabilities associated with it. These
* could be queried by other apps if necessary (see getCapabilities). For example,
* the RaptureAPIServer has a capability to handle api calls, and it posts its api
* uri, including port, as a capability, that other apps can retrieve if they want
* to contact the api directly. This method will record capabilities for a given instance.
*
*/
void recordInstanceCapabilities(CallingContext context, String serverName, String instanceName, Map capabilities);
/**
* Returns the capabilities for one or more instance running on the specified host.
* See also recordInstanceCapabilities.
*
*/
Map getCapabilities(CallingContext context, String serverName, List instanceNames);
/**
* Gets a list of all the known server names (aka hostnames). This is determined by
* finding where a RaptureRunner is currently running or has run in the past and recorded
* a status (which has not been deleted), whether it be up or down.
*
*/
List getRunnerServers(CallingContext context);
/**
* Get a RaptureRunnerStatus object for one specific host, which is a map of the statuses
* of all instances on a specific host.
*
*/
RaptureRunnerStatus getRunnerStatus(CallingContext context, String serverName);
/**
* Cleans out old status information, older than the passed parameter in minutes. It
* acquires a lock based on the server name, same as recordRunnerStatus and markForRestart.
*
*/
void cleanRunnerStatus(CallingContext context, int ageInMinutes);
/**
* Marks a running instance as needing reboot. If an application is not found as running
* on the specified server, nothing is done. This will not start a server that is not
* running. This acquires a lock based on the server name, same as recordRunnerStatus
* and cleanRunnerStatus.
*
*/
void markForRestart(CallingContext context, String serverName, String name);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy