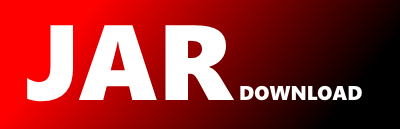
rapture.common.api.ScheduleApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.common.api;
import rapture.common.exception.RaptureException;
import rapture.common.CallingContext;
import java.util.List;
import java.util.Map;
import rapture.common.JobErrorAck;
import rapture.common.TimedEventRecord;
import rapture.common.RaptureJobExec;
import rapture.common.RaptureJob;
import rapture.common.WorkflowExecsStatus;
@SuppressWarnings("all")
public interface ScheduleApi {
/**
* Creates a new job. The executableURI should point to a RaptureScript. A job needs
* to be activated for it be available for execution. A job can be either auto-activate
* (i.e. it is activated, then de-activated while it runs, then activated on completion.
* OR it can be not-auto-activate, whereupon it needs to be activated manually, by
* either a predecessor job (a job that has this job as a dependency) or manually via
* the activate schedule API call.
*
*/
RaptureJob createJob(CallingContext context, String jobURI, String description, String scriptURI, String cronExpression, String timeZone, Map jobParams, Boolean autoActivate);
/**
* Creates a new Workflow-based job. The executableURI should point to a Workflow. A
* WorkOrder will be created when the job is executed. The jobParams will be passed
* in to the Workflow as the contextMap. The maxRuntimeMinutes will be used to throw
* alerts when the job runs longer than expected. A job needs to be activated for
* it be available for execution. A job can be either auto-activate (i.e. it is activated,
* then de-activated while it runs, then activated on completion. OR it can be not-auto-activate,
* whereupon it needs to be activated manually, by either a predecessor job (a job
* that has this job as a dependency) or manually via the activate schedule API call.
*
*/
RaptureJob createWorkflowJob(CallingContext context, String jobURI, String description, String workflowURI, String cronExpression, String timeZone, Map jobParams, Boolean autoActivate, int maxRuntimeMinutes, String appStatusNamePattern);
/**
* Activate a job (usually that is not auto-activate). This means that the job will
* now be picked up by the scheduler and executed at whatever time it is configured
* to run.
*
*/
void activateJob(CallingContext context, String jobURI, Map extraParams);
/**
* Turns off a job's schedule-based execution.
*
*/
void deactivateJob(CallingContext context, String jobURI);
/**
* Retrieve the definition of a job given its URI.
*
*/
RaptureJob retrieveJob(CallingContext context, String jobURI);
/**
* Retrieve the definition of all jobs in the system whose uri starts with a certain
* prefix (e.g job://my/jobs/date1)
*
*/
List retrieveJobs(CallingContext context, String uriPrefix);
/**
* Try to schedule this job to run as soon as possible.
*
*/
void runJobNow(CallingContext context, String jobURI, Map extraParams);
/**
* Removes the upcoming scheduled execution of this job and schedules it to run according
* to the cron in the job configuration.
*
*/
void resetJob(CallingContext context, String jobURI);
/**
* Retrieves the execution of a job.
*
*/
RaptureJobExec retrieveJobExec(CallingContext context, String jobURI, Long execTime);
/**
* Removes a job from the system.
*
*/
void deleteJob(CallingContext context, String jobURI);
/**
* Retrieves all of the JobURI addresses of the jobs in the system.
*
*/
List getJobs(CallingContext context);
/**
* Retrieves all of the upcoming jobs in the system.
*
*/
List getUpcomingJobs(CallingContext context);
/**
* Retrieves the status of all current workflow-based job executions. This looks into
* the last execution as well as upcoming execution for all scheduled jobs. The return
* object contains a list of jobs that succeeded, failed, are overrun, or are ok (i.e.
* either scheduled to start in the future or currently running but not overrun). For
* failed or overrun jobs, information is also returned as to whether the failure/overrun
* has been acknowledged. See also ackJobError.
*
*/
WorkflowExecsStatus getWorkflowExecsStatus(CallingContext context);
/**
* Acknowledges a job failure, storing the acknowledgment in Rapture. This information
* is returned when retrieving job statuses. See also getWorkflowExecsStatus.
*
*/
JobErrorAck ackJobError(CallingContext context, String jobURI, Long execTime, String jobErrorType);
/**
* Gets the next execution time for a given job.
*
*/
RaptureJobExec getNextExec(CallingContext context, String jobURI);
/**
* Retrieves a list of job executions in a given range.
*
*/
List getJobExecs(CallingContext context, String jobURI, int start, int count, Boolean reversed);
/**
* Retrieve a list of job executions for a list of jobs. This will return the job executions
* starting at index start (inclusive), and going on for count. If reverse is true,
* it starts from the end.
*
*/
List batchGetJobExecs(CallingContext context, List jobURI, int start, int count, Boolean reversed);
/**
* Return whether the given job is ready to run.
*
*/
Boolean isJobReadyToRun(CallingContext context, String toJobURI);
/**
* For TimeServer, get a list of scheduled events for this week (starts on Sunday, use
* offset to look at next week)
*
*/
List getCurrentWeekTimeRecords(CallingContext context, int weekOffsetfromNow);
/**
* For TimeServer, get a list of scheduled jobs for the current day
*
*/
List getCurrentDayJobs(CallingContext context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy