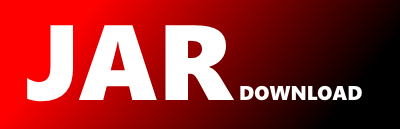
rapture.common.api.ScriptApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.common.api;
import rapture.common.exception.RaptureException;
import rapture.common.CallingContext;
import java.util.List;
import java.util.Map;
import rapture.common.ScriptResult;
import rapture.common.RaptureScriptLanguage;
import rapture.common.ScriptInterface;
import rapture.common.RaptureFolderInfo;
import rapture.common.RaptureSnippet;
import rapture.common.RaptureScript;
import rapture.common.RaptureScriptPurpose;
@SuppressWarnings("all")
public interface ScriptApi {
/**
* Creates a script in the system.
*
*/
RaptureScript createScript(CallingContext context, String scriptURI, RaptureScriptLanguage language, RaptureScriptPurpose purpose, String script);
/**
* Creates a symbolic link to a script in the system.
*
*/
void createScriptLink(CallingContext context, String fromScriptURI, String toScriptURI);
/**
* Removes a symbolic link to a script in the system.
*
*/
void removeScriptLink(CallingContext context, String fromScriptURI);
/**
* Returns true the given script was found.
*
*/
Boolean doesScriptExist(CallingContext context, String scriptURI);
/**
* Removes the script from the system.
*
*/
void deleteScript(CallingContext context, String scriptUri);
/**
* Retrieves all of the scripts within a authority.
*
*/
List getScriptNames(CallingContext context, String scriptURI);
/**
* Retrieves the contents of a script.
*
*/
RaptureScript getScript(CallingContext context, String scriptURI);
/**
* Retrieves the parameter information for a script.
*
*/
ScriptInterface getInterface(CallingContext context, String scriptURI);
/**
* Stores a script in the system using a RaptureScript object.TODO is there really any
* point in passing the URI? The storage location is based on RaptureScript. All
* we do is extract the Authority from the URI; the caller can do that.
*
*/
RaptureScript putScript(CallingContext context, String scriptURI, RaptureScript script);
/**
* Store a script in the system using raw inputs. Most users will want the value PROGRAM
* for purpose.
*
*/
RaptureScript putRawScript(CallingContext context, String scriptURI, String content, String language, String purpose, List param_types, List param_names);
/**
* Run a script in the Rapture environment.
*
*/
String runScript(CallingContext context, String scriptURI, Map parameters);
/**
* Runs a script in the Rapture environment.
*
*/
ScriptResult runScriptExtended(CallingContext context, String scriptURI, Map parameters);
/**
* Parses the script and returns any error messages from the parsing process. If the
* String returned is empty the script is valid Reflex.
*
*/
String checkScript(CallingContext context, String scriptURI);
/**
* Creates a Reflex REPL session that can be written to. These sessions eventually die
* if not used or killed
*
*/
String createREPLSession(CallingContext context);
/**
* Kills an existing Reflex REPL session.
*
*/
void destroyREPLSession(CallingContext context, String sessionId);
/**
* Adds a line to the current Reflex session, returns back what the parser/evaluator
* says
*
*/
String evaluateREPL(CallingContext context, String sessionId, String line);
/**
* Archive/delete old REPL sessions
*
*/
void archiveOldREPLSessions(CallingContext context, Long ageInMinutes);
/**
* Creates a code snippet and stores it in Rapture.
*
*/
RaptureSnippet createSnippet(CallingContext context, String snippetURI, String snippet);
/**
* Returns all children snippets with a given prefix.
*
*/
List getSnippetChildren(CallingContext context, String prefix);
/**
* Deletes a snippet by its URI.
*
*/
void deleteSnippet(CallingContext context, String snippetURI);
/**
* Retrieves a snippet by its URI.
*
*/
RaptureSnippet getSnippet(CallingContext context, String snippetURI);
/**
* Returns full pathnames for an entire subtree as a map of path to RFI.
*
*/
Map listScriptsByUriPrefix(CallingContext context, String scriptUri, int depth);
/**
* Removes a folder and its contents recursively, including empty subfolders. Returns
* a list of the scripts and folders removed.
*
*/
List deleteScriptsByUriPrefix(CallingContext context, String scriptUri);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy