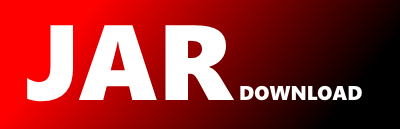
rapture.common.api.SearchApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.common.api;
import rapture.common.exception.RaptureException;
import rapture.common.CallingContext;
import java.util.List;
import java.util.Map;
import rapture.common.model.SearchRepoConfig;
import rapture.common.SearchResponse;
@SuppressWarnings("all")
public interface SearchApi {
/**
* Search for data using a Lucene compliant query string query. Default size of result
* set returned is 10
*
*/
SearchResponse search(CallingContext context, String query);
/**
* Search for data using a Lucene compliant query string query. This will return a
* cursor for scrolling thru the results. The initial call should pass in the cursor
* argument as null or empty, but subsequent calls should use the previously returned
* cursorId. The size parameter specifies how many results should be returned per
* call
*
*/
SearchResponse searchWithCursor(CallingContext context, String cursorId, int size, String query);
/**
* Search for data using a Lucene compliant query string query. Default size of result
* set returned is 10
*
*/
SearchResponse qualifiedSearch(CallingContext context, String searchRepo, List types, String query);
/**
* Search for data using a Lucene compliant query string query. This will return a
* cursor for scrolling thru the results. The initial call should pass in the cursor
* argument as null or empty, but subsequent calls should use the previously returned
* cursorId. The size parameter specifies how many results should be returned per
* call
*
*/
SearchResponse qualifiedSearchWithCursor(CallingContext context, String searchRepo, List types, String cursorId, int size, String query);
/**
* Validates repository; requires write permission because it can cause files/tables
* to be created on first use.
*
*/
Boolean validateSearchRepo(CallingContext context, String searchRepoUri);
/**
* A FTSearchRepository is used to store full text search repos.
*
*/
void createSearchRepo(CallingContext context, String searchRepoUri, String config);
/**
* This API call can be used to determine whether a given repository exists.
*
*/
Boolean searchRepoExists(CallingContext context, String searchRepoUri);
/**
* Retrieves the configuration string for the given search repository.
*
*/
SearchRepoConfig getSearchRepoConfig(CallingContext context, String searchRepoUri);
/**
* Retrieves search repositories
*
*/
List getSearchRepoConfigs(CallingContext context);
/**
* This method removes a search repository and its data from the Rapture system. There
* is no undo.
*
*/
void deleteSearchRepo(CallingContext context, String searchRepoUri);
/**
* This method rebuilds the search index associated with a repo (authority) by dropping
* it and recreating it (asynchronously). The repoUri passed in must have the scheme
* as the prefix e.g. document://myrepo or series://myrepo2
*
*/
void rebuildRepoIndex(CallingContext context, String repoUri);
/**
* This method just drops the search index associated with a repo (authority). The
* repoUri passed in must have the scheme as the prefix e.g. document://myrepo or series://myrepo2.
* It is done asynchronously.
*
*/
void dropRepoIndex(CallingContext context, String repoUri);
/**
* Initialize all search repos
*
*/
void startSearchRepos(CallingContext context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy