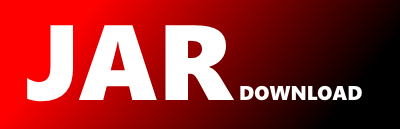
rapture.common.api.SeriesApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.common.api;
import rapture.common.exception.RaptureException;
import rapture.common.CallingContext;
import java.util.List;
import java.util.Map;
import rapture.common.SeriesDouble;
import rapture.common.RaptureFolderInfo;
import rapture.common.SeriesRepoConfig;
import rapture.common.SeriesPoint;
import rapture.common.SeriesString;
@SuppressWarnings("all")
public interface SeriesApi {
/**
* Creates a repository for series data.
*
*/
void createSeriesRepo(CallingContext context, String seriesRepoUri, String config);
/**
* Creates an empty series.
*
*/
void createSeries(CallingContext context, String seriesUri);
/**
* Check for the existence of a given repository
*
*/
Boolean seriesRepoExists(CallingContext context, String seriesRepoUri);
/**
* Check for the existence of a given series
*
*/
Boolean seriesExists(CallingContext context, String seriesUri);
/**
* Fetches the series repository config, or null if the repository is not found.
*
*/
SeriesRepoConfig getSeriesRepoConfig(CallingContext context, String seriesRepoUri);
/**
* Fetch a list of all series repositories.
*
*/
List getSeriesRepoConfigs(CallingContext context);
/**
* This method removes a Series Repository and its data from the Rapture system. There
* is no undo.
*
*/
void deleteSeriesRepo(CallingContext context, String seriesRepoUri);
/**
* This method removes a Series and its data from the Rapture system. There is no undo.
*
*/
void deleteSeries(CallingContext context, String seriesUri);
/**
* Recursively removes all series repositories that are children of the given Uri.
*
*/
List deleteSeriesByUriPrefix(CallingContext context, String seriesUri);
/**
* Adds one point of floating-point data to a given series.
*
*/
void addDoubleToSeries(CallingContext context, String seriesUri, String pointKey, Double pointValue);
/**
* Adds one point of type long to a given series.
*
*/
void addLongToSeries(CallingContext context, String seriesUri, String pointKey, Long pointValue);
/**
* Adds one point of string data to a given series.
*
*/
void addStringToSeries(CallingContext context, String seriesUri, String pointKey, String pointValue);
/**
* Adds one point containing a JSON-encoded structure to a given series.
*
*/
void addStructureToSeries(CallingContext context, String seriesUri, String pointKey, String pointValue);
/**
* Adds a list of points of floating-point data to a given series.
*
*/
void addDoublesToSeries(CallingContext context, String seriesUri, List pointKeys, List pointValues);
/**
* Adds a list of points of type long to a given series.
*
*/
void addLongsToSeries(CallingContext context, String seriesUri, List pointKeys, List pointValues);
/**
* Adds a list of string points to a given series.
*
*/
void addStringsToSeries(CallingContext context, String seriesUri, List pointKeys, List pointValues);
/**
* Adds a list of points containing JSON-encoded structures to a series.
*
*/
void addStructuresToSeries(CallingContext context, String seriesUri, List pointKeys, List pointValues);
/**
* Delete a list of points from a series.
*
*/
void deletePointsFromSeriesByPointKey(CallingContext context, String seriesUri, List pointKeys);
/**
* Removes all points in a series, then removes the series from the directory listing
* for its parent folder.
*
*/
void deletePointsFromSeries(CallingContext context, String seriesUri);
/**
* Retrieves the last point in a series.
*
*/
SeriesPoint getLastPoint(CallingContext context, String seriesUri);
/**
* If the series size is less than the maximum batch size (one million points by default),
* this returns all points in a list. If the series is larger, an exception is thrown.
*
*/
List getPoints(CallingContext context, String seriesUri);
/**
* Gets one page of data from a series
*
*/
List getPointsAfter(CallingContext context, String seriesUri, String startColumn, int maxNumber);
/**
* Gets one page of data and reverses the normal sort order
*
*/
List getPointsAfterReverse(CallingContext context, String seriesUri, String startColumn, int maxNumber);
/**
* Gets one page of data from a series range.
*
*/
List getPointsInRange(CallingContext context, String seriesUri, String startColumn, String endColumn, int maxNumber);
/**
* Gets the entire contents of a series and casts each value to type Double.
*
*/
List getPointsAsDoubles(CallingContext context, String seriesUri);
/**
* Gets one page of data from a series and casts each value to type Double.
*
*/
List getPointsAfterAsDoubles(CallingContext context, String seriesUri, String startColumn, int maxNumber);
/**
* Gets one page of data from a series range and casts each value to type Double.
*
*/
List getPointsInRangeAsDoubles(CallingContext context, String seriesUri, String startColumn, String endColumn, int maxNumber);
/**
* Gets the entire contents of a series and casts each value to type String.
*
*/
List getPointsAsStrings(CallingContext context, String seriesUri);
/**
* Gets one page of data from a series and casts each value to type String.
*
*/
List getPointsAfterAsStrings(CallingContext context, String seriesUri, String startColumn, int maxNumber);
/**
* Gets one page of data from a series range and casts each value to type String.
*
*/
List getPointsInRangeAsStrings(CallingContext context, String seriesUri, String startColumn, String endColumn, int maxNumber);
/**
* Executes a series function program and returns its default output.
*
*/
List runSeriesScript(CallingContext context, String scriptContent, List arguments);
/**
* Executes a series function program and returns success status only.
*
*/
void runSeriesScriptQuiet(CallingContext context, String scriptContent, List arguments);
/**
* Returns full pathnames for an entire subtree as a map of path to RFI.
*
*/
Map listSeriesByUriPrefix(CallingContext context, String seriesUri, int depth);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy