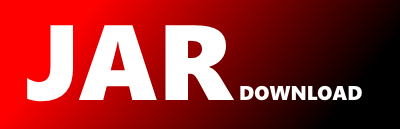
rapture.common.api.StructuredApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.common.api;
import rapture.common.exception.RaptureException;
import rapture.common.CallingContext;
import java.util.List;
import java.util.Map;
import rapture.common.StoredProcedureParams;
import rapture.common.StoredProcedureResponse;
import rapture.common.TableIndex;
import rapture.common.StructuredRepoConfig;
import rapture.common.ForeignKey;
@SuppressWarnings("all")
public interface StructuredApi {
/**
* Create a repository for structured data
*
*/
void createStructuredRepo(CallingContext context, String uri, String config);
/**
* Delete a repository for structured data
*
*/
void deleteStructuredRepo(CallingContext context, String uri);
/**
* check existence
*
*/
Boolean structuredRepoExists(CallingContext context, String uri);
/**
* get a specific structured repo config given a uri
*
*/
StructuredRepoConfig getStructuredRepoConfig(CallingContext context, String uri);
/**
* get list of all configurations
*
*/
List getStructuredRepoConfigs(CallingContext context);
/**
* create a structured table using raw sql
*
*/
void createTableUsingSql(CallingContext context, String schema, String rawSql);
/**
* create a structured table using a column name to SQL column type map
*
*/
void createTable(CallingContext context, String tableUri, Map columns);
/**
* drop a structured table and all of its data
*
*/
void dropTable(CallingContext context, String tableUri);
/**
* check if table exists
*
*/
Boolean tableExists(CallingContext context, String tableUri);
/**
* get all schemas
*
*/
List getSchemas(CallingContext context);
/**
* get all tables of a certain schema
*
*/
List getTables(CallingContext context, String repoUri);
/**
* get table description
*
*/
Map describeTable(CallingContext context, String tableUri);
/**
* add column(s) to an existing table. Table must exist beforehand
*
*/
void addTableColumns(CallingContext context, String tableUri, Map columns);
/**
* remove column(s) from an existing table. Table must exist beforehand
*
*/
void deleteTableColumns(CallingContext context, String tableUri, List columnNames);
/**
* update column(s) in an existing table. Table must exist beforehand
*
*/
void updateTableColumns(CallingContext context, String tableUri, Map columns);
/**
* rename column(s) in an existing table. Table must exist beforehand
*
*/
void renameTableColumns(CallingContext context, String tableUri, Map columnNames);
/**
* create an index on a structured table
*
*/
void createIndex(CallingContext context, String tableUri, String indexName, List columnNames);
/**
* remove an index that was previously created on a table
*
*/
void dropIndex(CallingContext context, String tableUri, String indexName);
/**
* get all indexes on a structured table
*
*/
List getIndexes(CallingContext context, String tableUri);
/**
* retrieve data from multiple tables
*
*/
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy