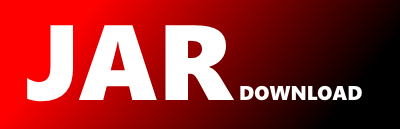
rapture.common.client.HttpBlobApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This file is autogenerated and any changes will be overwritten.
*/
package rapture.common.client;
import java.util.List;
import java.util.Map;
import rapture.common.api.BlobApi;
import rapture.common.api.ScriptBlobApi;
import rapture.common.CallingContext;
import rapture.common.model.GeneralResponse;
import rapture.common.model.BasePayload;
import rapture.common.exception.RaptureException;
import rapture.common.impl.jackson.JacksonUtil;
import com.fasterxml.jackson.core.type.TypeReference;
import org.apache.log4j.Logger;
import rapture.common.BlobContainer;
import rapture.common.RaptureFolderInfo;
import rapture.common.model.BlobRepoConfig;
import rapture.common.shared.blob.CreateBlobRepoPayload;
import rapture.common.shared.blob.GetBlobRepoConfigPayload;
import rapture.common.shared.blob.GetBlobRepoConfigsPayload;
import rapture.common.shared.blob.DeleteBlobRepoPayload;
import rapture.common.shared.blob.BlobRepoExistsPayload;
import rapture.common.shared.blob.BlobExistsPayload;
import rapture.common.shared.blob.AddBlobContentPayload;
import rapture.common.shared.blob.PutBlobPayload;
import rapture.common.shared.blob.GetBlobPayload;
import rapture.common.shared.blob.DeleteBlobPayload;
import rapture.common.shared.blob.GetBlobSizePayload;
import rapture.common.shared.blob.PutBlobMetaDataPayload;
import rapture.common.shared.blob.GetBlobMetaDataPayload;
import rapture.common.shared.blob.ListBlobsByUriPrefixPayload;
import rapture.common.shared.blob.DeleteBlobsByUriPrefixPayload;
@SuppressWarnings("all")
public class HttpBlobApi extends BaseHttpApi implements BlobApi, ScriptBlobApi {
private static final Logger log = Logger.getLogger(HttpBlobApi.class);
public HttpBlobApi(HttpLoginApi login) {
super(login, "blob");
}
@Override
public void createBlobRepo(CallingContext context, String blobRepoUri, String config, String metaConfig) {
CreateBlobRepoPayload requestObj = new CreateBlobRepoPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setBlobRepoUri(blobRepoUri);
requestObj.setConfig(config);
requestObj.setMetaConfig(metaConfig);
doRequest(requestObj, "CREATEBLOBREPO", null); }
private static final class GetBlobRepoConfigTypeReference extends TypeReference {
}
@Override
public BlobRepoConfig getBlobRepoConfig(CallingContext context, String blobRepoUri) {
GetBlobRepoConfigPayload requestObj = new GetBlobRepoConfigPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setBlobRepoUri(blobRepoUri);
return doRequest(requestObj, "GETBLOBREPOCONFIG", new GetBlobRepoConfigTypeReference()); }
private static final class GetBlobRepoConfigsTypeReference extends TypeReference> {
}
@Override
public List getBlobRepoConfigs(CallingContext context) {
GetBlobRepoConfigsPayload requestObj = new GetBlobRepoConfigsPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETBLOBREPOCONFIGS", new GetBlobRepoConfigsTypeReference()); }
@Override
public void deleteBlobRepo(CallingContext context, String repoUri) {
DeleteBlobRepoPayload requestObj = new DeleteBlobRepoPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setRepoUri(repoUri);
doRequest(requestObj, "DELETEBLOBREPO", null); }
private static final class BlobRepoExistsTypeReference extends TypeReference {
}
@Override
public Boolean blobRepoExists(CallingContext context, String repoUri) {
BlobRepoExistsPayload requestObj = new BlobRepoExistsPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setRepoUri(repoUri);
return doRequest(requestObj, "BLOBREPOEXISTS", new BlobRepoExistsTypeReference()); }
private static final class BlobExistsTypeReference extends TypeReference {
}
@Override
public Boolean blobExists(CallingContext context, String blobUri) {
BlobExistsPayload requestObj = new BlobExistsPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setBlobUri(blobUri);
return doRequest(requestObj, "BLOBEXISTS", new BlobExistsTypeReference()); }
@Override
public void addBlobContent(CallingContext context, String blobUri, byte[] content) {
AddBlobContentPayload requestObj = new AddBlobContentPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setBlobUri(blobUri);
requestObj.setContent(content);
doRequest(requestObj, "ADDBLOBCONTENT", null); }
@Override
public void putBlob(CallingContext context, String blobUri, byte[] content, String contentType) {
PutBlobPayload requestObj = new PutBlobPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setBlobUri(blobUri);
requestObj.setContent(content);
requestObj.setContentType(contentType);
doRequest(requestObj, "PUTBLOB", null); }
private static final class GetBlobTypeReference extends TypeReference {
}
@Override
public BlobContainer getBlob(CallingContext context, String blobUri) {
GetBlobPayload requestObj = new GetBlobPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setBlobUri(blobUri);
return doRequest(requestObj, "GETBLOB", new GetBlobTypeReference()); }
@Override
public void deleteBlob(CallingContext context, String blobUri) {
DeleteBlobPayload requestObj = new DeleteBlobPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setBlobUri(blobUri);
doRequest(requestObj, "DELETEBLOB", null); }
private static final class GetBlobSizeTypeReference extends TypeReference {
}
@Override
public Long getBlobSize(CallingContext context, String blobUri) {
GetBlobSizePayload requestObj = new GetBlobSizePayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setBlobUri(blobUri);
return doRequest(requestObj, "GETBLOBSIZE", new GetBlobSizeTypeReference()); }
@Override
public void putBlobMetaData(CallingContext context, String blobUri, Map metadata) {
PutBlobMetaDataPayload requestObj = new PutBlobMetaDataPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setBlobUri(blobUri);
requestObj.setMetadata(metadata);
doRequest(requestObj, "PUTBLOBMETADATA", null); }
private static final class GetBlobMetaDataTypeReference extends TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy