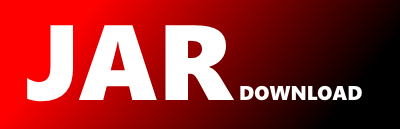
rapture.common.client.HttpEnvironmentApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This file is autogenerated and any changes will be overwritten.
*/
package rapture.common.client;
import java.util.List;
import java.util.Map;
import rapture.common.api.EnvironmentApi;
import rapture.common.api.ScriptEnvironmentApi;
import rapture.common.CallingContext;
import rapture.common.model.GeneralResponse;
import rapture.common.model.BasePayload;
import rapture.common.exception.RaptureException;
import rapture.common.impl.jackson.JacksonUtil;
import com.fasterxml.jackson.core.type.TypeReference;
import org.apache.log4j.Logger;
import rapture.common.model.RaptureServerStatus;
import rapture.common.model.RaptureServerInfo;
import rapture.common.shared.environment.GetThisServerPayload;
import rapture.common.shared.environment.GetServersPayload;
import rapture.common.shared.environment.SetThisServerPayload;
import rapture.common.shared.environment.SetApplianceModePayload;
import rapture.common.shared.environment.GetApplianceModePayload;
import rapture.common.shared.environment.GetServerStatusPayload;
import rapture.common.shared.environment.GetAppNamesPayload;
import rapture.common.shared.environment.GetMemoryInfoPayload;
import rapture.common.shared.environment.GetOperatingSystemInfoPayload;
import rapture.common.shared.environment.GetThreadInfoPayload;
import rapture.common.shared.environment.ReadByPathPayload;
import rapture.common.shared.environment.WriteByPathPayload;
import rapture.common.shared.environment.ExecByPathPayload;
import rapture.common.shared.environment.ReadByJsonPayload;
import rapture.common.shared.environment.WriteByJsonPayload;
import rapture.common.shared.environment.ExecByJsonPayload;
@SuppressWarnings("all")
public class HttpEnvironmentApi extends BaseHttpApi implements EnvironmentApi, ScriptEnvironmentApi {
private static final Logger log = Logger.getLogger(HttpEnvironmentApi.class);
public HttpEnvironmentApi(HttpLoginApi login) {
super(login, "environment");
}
private static final class GetThisServerTypeReference extends TypeReference {
}
@Override
public RaptureServerInfo getThisServer(CallingContext context) {
GetThisServerPayload requestObj = new GetThisServerPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETTHISSERVER", new GetThisServerTypeReference()); }
private static final class GetServersTypeReference extends TypeReference> {
}
@Override
public List getServers(CallingContext context) {
GetServersPayload requestObj = new GetServersPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETSERVERS", new GetServersTypeReference()); }
private static final class SetThisServerTypeReference extends TypeReference {
}
@Override
public RaptureServerInfo setThisServer(CallingContext context, RaptureServerInfo info) {
SetThisServerPayload requestObj = new SetThisServerPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setInfo(info);
return doRequest(requestObj, "SETTHISSERVER", new SetThisServerTypeReference()); }
@Override
public void setApplianceMode(CallingContext context, Boolean mode) {
SetApplianceModePayload requestObj = new SetApplianceModePayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setMode(mode);
doRequest(requestObj, "SETAPPLIANCEMODE", null); }
private static final class GetApplianceModeTypeReference extends TypeReference {
}
@Override
public Boolean getApplianceMode(CallingContext context) {
GetApplianceModePayload requestObj = new GetApplianceModePayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETAPPLIANCEMODE", new GetApplianceModeTypeReference()); }
private static final class GetServerStatusTypeReference extends TypeReference> {
}
@Override
public List getServerStatus(CallingContext context) {
GetServerStatusPayload requestObj = new GetServerStatusPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETSERVERSTATUS", new GetServerStatusTypeReference()); }
private static final class GetAppNamesTypeReference extends TypeReference> {
}
@Override
public List getAppNames(CallingContext context) {
GetAppNamesPayload requestObj = new GetAppNamesPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETAPPNAMES", new GetAppNamesTypeReference()); }
private static final class GetMemoryInfoTypeReference extends TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy