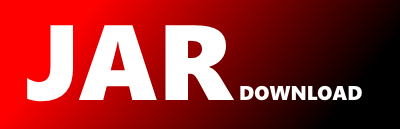
rapture.common.client.HttpEventApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This file is autogenerated and any changes will be overwritten.
*/
package rapture.common.client;
import java.util.List;
import java.util.Map;
import rapture.common.api.EventApi;
import rapture.common.api.ScriptEventApi;
import rapture.common.CallingContext;
import rapture.common.model.GeneralResponse;
import rapture.common.model.BasePayload;
import rapture.common.exception.RaptureException;
import rapture.common.impl.jackson.JacksonUtil;
import com.fasterxml.jackson.core.type.TypeReference;
import org.apache.log4j.Logger;
import rapture.common.RaptureFolderInfo;
import rapture.common.model.RaptureEvent;
import rapture.common.model.RunEventHandle;
import rapture.common.shared.event.GetEventPayload;
import rapture.common.shared.event.PutEventPayload;
import rapture.common.shared.event.DeleteEventPayload;
import rapture.common.shared.event.ListEventsByUriPrefixPayload;
import rapture.common.shared.event.AddEventScriptPayload;
import rapture.common.shared.event.DeleteEventScriptPayload;
import rapture.common.shared.event.AddEventMessagePayload;
import rapture.common.shared.event.DeleteEventMessagePayload;
import rapture.common.shared.event.AddEventNotificationPayload;
import rapture.common.shared.event.DeleteEventNotificationPayload;
import rapture.common.shared.event.AddEventWorkflowPayload;
import rapture.common.shared.event.DeleteEventWorkflowPayload;
import rapture.common.shared.event.RunEventPayload;
import rapture.common.shared.event.RunEventWithContextPayload;
import rapture.common.shared.event.EventExistsPayload;
import rapture.common.shared.event.DeleteEventsByUriPrefixPayload;
@SuppressWarnings("all")
public class HttpEventApi extends BaseHttpApi implements EventApi, ScriptEventApi {
private static final Logger log = Logger.getLogger(HttpEventApi.class);
public HttpEventApi(HttpLoginApi login) {
super(login, "event");
}
private static final class GetEventTypeReference extends TypeReference {
}
@Override
public RaptureEvent getEvent(CallingContext context, String eventUri) {
GetEventPayload requestObj = new GetEventPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
return doRequest(requestObj, "GETEVENT", new GetEventTypeReference()); }
@Override
public void putEvent(CallingContext context, RaptureEvent event) {
PutEventPayload requestObj = new PutEventPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEvent(event);
doRequest(requestObj, "PUTEVENT", null); }
@Override
public void deleteEvent(CallingContext context, String eventUri) {
DeleteEventPayload requestObj = new DeleteEventPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
doRequest(requestObj, "DELETEEVENT", null); }
private static final class ListEventsByUriPrefixTypeReference extends TypeReference> {
}
@Override
public List listEventsByUriPrefix(CallingContext context, String eventUriPrefix) {
ListEventsByUriPrefixPayload requestObj = new ListEventsByUriPrefixPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUriPrefix(eventUriPrefix);
return doRequest(requestObj, "LISTEVENTSBYURIPREFIX", new ListEventsByUriPrefixTypeReference()); }
@Override
public void addEventScript(CallingContext context, String eventUri, String scriptUri, Boolean performOnce) {
AddEventScriptPayload requestObj = new AddEventScriptPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
requestObj.setScriptUri(scriptUri);
requestObj.setPerformOnce(performOnce);
doRequest(requestObj, "ADDEVENTSCRIPT", null); }
@Override
public void deleteEventScript(CallingContext context, String eventUri, String scriptUri) {
DeleteEventScriptPayload requestObj = new DeleteEventScriptPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
requestObj.setScriptUri(scriptUri);
doRequest(requestObj, "DELETEEVENTSCRIPT", null); }
@Override
public void addEventMessage(CallingContext context, String eventUri, String name, String pipeline, Map params) {
AddEventMessagePayload requestObj = new AddEventMessagePayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
requestObj.setName(name);
requestObj.setPipeline(pipeline);
requestObj.setParams(params);
doRequest(requestObj, "ADDEVENTMESSAGE", null); }
@Override
public void deleteEventMessage(CallingContext context, String eventUri, String name) {
DeleteEventMessagePayload requestObj = new DeleteEventMessagePayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
requestObj.setName(name);
doRequest(requestObj, "DELETEEVENTMESSAGE", null); }
@Override
public void addEventNotification(CallingContext context, String eventUri, String name, String notification, Map params) {
AddEventNotificationPayload requestObj = new AddEventNotificationPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
requestObj.setName(name);
requestObj.setNotification(notification);
requestObj.setParams(params);
doRequest(requestObj, "ADDEVENTNOTIFICATION", null); }
@Override
public void deleteEventNotification(CallingContext context, String eventUri, String name) {
DeleteEventNotificationPayload requestObj = new DeleteEventNotificationPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
requestObj.setName(name);
doRequest(requestObj, "DELETEEVENTNOTIFICATION", null); }
@Override
public void addEventWorkflow(CallingContext context, String eventUri, String name, String workflowUri, Map params) {
AddEventWorkflowPayload requestObj = new AddEventWorkflowPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
requestObj.setName(name);
requestObj.setWorkflowUri(workflowUri);
requestObj.setParams(params);
doRequest(requestObj, "ADDEVENTWORKFLOW", null); }
@Override
public void deleteEventWorkflow(CallingContext context, String eventUri, String name) {
DeleteEventWorkflowPayload requestObj = new DeleteEventWorkflowPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
requestObj.setName(name);
doRequest(requestObj, "DELETEEVENTWORKFLOW", null); }
private static final class RunEventTypeReference extends TypeReference {
}
@Override
public Boolean runEvent(CallingContext context, String eventUri, String associatedUri, String eventContext) {
RunEventPayload requestObj = new RunEventPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
requestObj.setAssociatedUri(associatedUri);
requestObj.setEventContext(eventContext);
return doRequest(requestObj, "RUNEVENT", new RunEventTypeReference()); }
private static final class RunEventWithContextTypeReference extends TypeReference {
}
@Override
public RunEventHandle runEventWithContext(CallingContext context, String eventUri, String associatedUri, Map eventContextMap) {
RunEventWithContextPayload requestObj = new RunEventWithContextPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
requestObj.setAssociatedUri(associatedUri);
requestObj.setEventContextMap(eventContextMap);
return doRequest(requestObj, "RUNEVENTWITHCONTEXT", new RunEventWithContextTypeReference()); }
private static final class EventExistsTypeReference extends TypeReference {
}
@Override
public Boolean eventExists(CallingContext context, String eventUri) {
EventExistsPayload requestObj = new EventExistsPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setEventUri(eventUri);
return doRequest(requestObj, "EVENTEXISTS", new EventExistsTypeReference()); }
private static final class DeleteEventsByUriPrefixTypeReference extends TypeReference> {
}
@Override
public List deleteEventsByUriPrefix(CallingContext context, String uriPrefix) {
DeleteEventsByUriPrefixPayload requestObj = new DeleteEventsByUriPrefixPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setUriPrefix(uriPrefix);
return doRequest(requestObj, "DELETEEVENTSBYURIPREFIX", new DeleteEventsByUriPrefixTypeReference()); }
@Override
public RaptureEvent getEvent(String eventUri) {
return getEvent(null,eventUri); }
@Override
public void putEvent(RaptureEvent event) {
putEvent(null,event); }
@Override
public void deleteEvent(String eventUri) {
deleteEvent(null,eventUri); }
@Override
public List listEventsByUriPrefix(String eventUriPrefix) {
return listEventsByUriPrefix(null,eventUriPrefix); }
@Override
public void addEventScript(String eventUri, String scriptUri, Boolean performOnce) {
addEventScript(null,eventUri,scriptUri,performOnce); }
@Override
public void deleteEventScript(String eventUri, String scriptUri) {
deleteEventScript(null,eventUri,scriptUri); }
@Override
public void addEventMessage(String eventUri, String name, String pipeline, Map params) {
addEventMessage(null,eventUri,name,pipeline,params); }
@Override
public void deleteEventMessage(String eventUri, String name) {
deleteEventMessage(null,eventUri,name); }
@Override
public void addEventNotification(String eventUri, String name, String notification, Map params) {
addEventNotification(null,eventUri,name,notification,params); }
@Override
public void deleteEventNotification(String eventUri, String name) {
deleteEventNotification(null,eventUri,name); }
@Override
public void addEventWorkflow(String eventUri, String name, String workflowUri, Map params) {
addEventWorkflow(null,eventUri,name,workflowUri,params); }
@Override
public void deleteEventWorkflow(String eventUri, String name) {
deleteEventWorkflow(null,eventUri,name); }
@Override
public Boolean runEvent(String eventUri, String associatedUri, String eventContext) {
return runEvent(null,eventUri,associatedUri,eventContext); }
@Override
public RunEventHandle runEventWithContext(String eventUri, String associatedUri, Map eventContextMap) {
return runEventWithContext(null,eventUri,associatedUri,eventContextMap); }
@Override
public Boolean eventExists(String eventUri) {
return eventExists(null,eventUri); }
@Override
public List deleteEventsByUriPrefix(String uriPrefix) {
return deleteEventsByUriPrefix(null,uriPrefix); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy