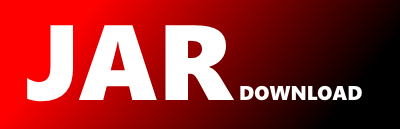
rapture.common.client.HttpPipelineApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This file is autogenerated and any changes will be overwritten.
*/
package rapture.common.client;
import java.util.List;
import java.util.Map;
import rapture.common.api.PipelineApi;
import rapture.common.api.ScriptPipelineApi;
import rapture.common.CallingContext;
import rapture.common.model.GeneralResponse;
import rapture.common.model.BasePayload;
import rapture.common.exception.RaptureException;
import rapture.common.impl.jackson.JacksonUtil;
import com.fasterxml.jackson.core.type.TypeReference;
import org.apache.log4j.Logger;
import rapture.common.RapturePipelineTask;
import rapture.common.CategoryQueueBindings;
import rapture.common.PipelineTaskStatus;
import rapture.common.model.RaptureExchange;
import rapture.common.TableQuery;
import rapture.common.shared.pipeline.RemoveServerCategoryPayload;
import rapture.common.shared.pipeline.GetServerCategoriesPayload;
import rapture.common.shared.pipeline.GetBoundExchangesPayload;
import rapture.common.shared.pipeline.DeregisterPipelineExchangePayload;
import rapture.common.shared.pipeline.GetExchangesPayload;
import rapture.common.shared.pipeline.GetExchangePayload;
import rapture.common.shared.pipeline.PublishMessageToCategoryPayload;
import rapture.common.shared.pipeline.BroadcastMessageToCategoryPayload;
import rapture.common.shared.pipeline.BroadcastMessageToAllPayload;
import rapture.common.shared.pipeline.GetStatusPayload;
import rapture.common.shared.pipeline.QueryTasksPayload;
import rapture.common.shared.pipeline.QueryTasksOldPayload;
import rapture.common.shared.pipeline.GetLatestTaskEpochPayload;
import rapture.common.shared.pipeline.DrainPipelinePayload;
import rapture.common.shared.pipeline.RegisterExchangeDomainPayload;
import rapture.common.shared.pipeline.DeregisterExchangeDomainPayload;
import rapture.common.shared.pipeline.GetExchangeDomainsPayload;
import rapture.common.shared.pipeline.SetupStandardCategoryPayload;
import rapture.common.shared.pipeline.MakeRPCPayload;
import rapture.common.shared.pipeline.CreateTopicExchangePayload;
import rapture.common.shared.pipeline.PublishTopicMessagePayload;
@SuppressWarnings("all")
public class HttpPipelineApi extends BaseHttpApi implements PipelineApi, ScriptPipelineApi {
private static final Logger log = Logger.getLogger(HttpPipelineApi.class);
public HttpPipelineApi(HttpLoginApi login) {
super(login, "pipeline");
}
@Override
public void removeServerCategory(CallingContext context, String category) {
RemoveServerCategoryPayload requestObj = new RemoveServerCategoryPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setCategory(category);
doRequest(requestObj, "REMOVESERVERCATEGORY", null); }
private static final class GetServerCategoriesTypeReference extends TypeReference> {
}
@Override
public List getServerCategories(CallingContext context) {
GetServerCategoriesPayload requestObj = new GetServerCategoriesPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETSERVERCATEGORIES", new GetServerCategoriesTypeReference()); }
private static final class GetBoundExchangesTypeReference extends TypeReference> {
}
@Override
public List getBoundExchanges(CallingContext context, String category) {
GetBoundExchangesPayload requestObj = new GetBoundExchangesPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setCategory(category);
return doRequest(requestObj, "GETBOUNDEXCHANGES", new GetBoundExchangesTypeReference()); }
@Override
public void deregisterPipelineExchange(CallingContext context, String name) {
DeregisterPipelineExchangePayload requestObj = new DeregisterPipelineExchangePayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setName(name);
doRequest(requestObj, "DEREGISTERPIPELINEEXCHANGE", null); }
private static final class GetExchangesTypeReference extends TypeReference> {
}
@Override
public List getExchanges(CallingContext context) {
GetExchangesPayload requestObj = new GetExchangesPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETEXCHANGES", new GetExchangesTypeReference()); }
private static final class GetExchangeTypeReference extends TypeReference {
}
@Override
public RaptureExchange getExchange(CallingContext context, String name) {
GetExchangePayload requestObj = new GetExchangePayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setName(name);
return doRequest(requestObj, "GETEXCHANGE", new GetExchangeTypeReference()); }
@Override
public void publishMessageToCategory(CallingContext context, RapturePipelineTask task) {
PublishMessageToCategoryPayload requestObj = new PublishMessageToCategoryPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setTask(task);
doRequest(requestObj, "PUBLISHMESSAGETOCATEGORY", null); }
@Override
public void broadcastMessageToCategory(CallingContext context, RapturePipelineTask task) {
BroadcastMessageToCategoryPayload requestObj = new BroadcastMessageToCategoryPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setTask(task);
doRequest(requestObj, "BROADCASTMESSAGETOCATEGORY", null); }
@Override
public void broadcastMessageToAll(CallingContext context, RapturePipelineTask task) {
BroadcastMessageToAllPayload requestObj = new BroadcastMessageToAllPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setTask(task);
doRequest(requestObj, "BROADCASTMESSAGETOALL", null); }
private static final class GetStatusTypeReference extends TypeReference {
}
@Override
public PipelineTaskStatus getStatus(CallingContext context, String taskId) {
GetStatusPayload requestObj = new GetStatusPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setTaskId(taskId);
return doRequest(requestObj, "GETSTATUS", new GetStatusTypeReference()); }
private static final class QueryTasksTypeReference extends TypeReference> {
}
@Override
public List queryTasks(CallingContext context, String query) {
QueryTasksPayload requestObj = new QueryTasksPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setQuery(query);
return doRequest(requestObj, "QUERYTASKS", new QueryTasksTypeReference()); }
private static final class QueryTasksOldTypeReference extends TypeReference> {
}
@Override
public List queryTasksOld(CallingContext context, TableQuery query) {
QueryTasksOldPayload requestObj = new QueryTasksOldPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setQuery(query);
return doRequest(requestObj, "QUERYTASKSOLD", new QueryTasksOldTypeReference()); }
private static final class GetLatestTaskEpochTypeReference extends TypeReference {
}
@Override
public Long getLatestTaskEpoch(CallingContext context) {
GetLatestTaskEpochPayload requestObj = new GetLatestTaskEpochPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETLATESTTASKEPOCH", new GetLatestTaskEpochTypeReference()); }
@Override
public void drainPipeline(CallingContext context, String exchange) {
DrainPipelinePayload requestObj = new DrainPipelinePayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setExchange(exchange);
doRequest(requestObj, "DRAINPIPELINE", null); }
@Override
public void registerExchangeDomain(CallingContext context, String domainURI, String config) {
RegisterExchangeDomainPayload requestObj = new RegisterExchangeDomainPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setDomainURI(domainURI);
requestObj.setConfig(config);
doRequest(requestObj, "REGISTEREXCHANGEDOMAIN", null); }
@Override
public void deregisterExchangeDomain(CallingContext context, String domainURI) {
DeregisterExchangeDomainPayload requestObj = new DeregisterExchangeDomainPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setDomainURI(domainURI);
doRequest(requestObj, "DEREGISTEREXCHANGEDOMAIN", null); }
private static final class GetExchangeDomainsTypeReference extends TypeReference> {
}
@Override
public List getExchangeDomains(CallingContext context) {
GetExchangeDomainsPayload requestObj = new GetExchangeDomainsPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETEXCHANGEDOMAINS", new GetExchangeDomainsTypeReference()); }
@Override
public void setupStandardCategory(CallingContext context, String category) {
SetupStandardCategoryPayload requestObj = new SetupStandardCategoryPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setCategory(category);
doRequest(requestObj, "SETUPSTANDARDCATEGORY", null); }
private static final class MakeRPCTypeReference extends TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy