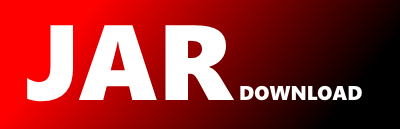
rapture.common.client.HttpScheduleApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This file is autogenerated and any changes will be overwritten.
*/
package rapture.common.client;
import java.util.List;
import java.util.Map;
import rapture.common.api.ScheduleApi;
import rapture.common.api.ScriptScheduleApi;
import rapture.common.CallingContext;
import rapture.common.model.GeneralResponse;
import rapture.common.model.BasePayload;
import rapture.common.exception.RaptureException;
import rapture.common.impl.jackson.JacksonUtil;
import com.fasterxml.jackson.core.type.TypeReference;
import org.apache.log4j.Logger;
import rapture.common.JobErrorAck;
import rapture.common.TimedEventRecord;
import rapture.common.RaptureJobExec;
import rapture.common.RaptureJob;
import rapture.common.WorkflowExecsStatus;
import rapture.common.shared.schedule.CreateJobPayload;
import rapture.common.shared.schedule.CreateWorkflowJobPayload;
import rapture.common.shared.schedule.ActivateJobPayload;
import rapture.common.shared.schedule.DeactivateJobPayload;
import rapture.common.shared.schedule.RetrieveJobPayload;
import rapture.common.shared.schedule.RetrieveJobsPayload;
import rapture.common.shared.schedule.RunJobNowPayload;
import rapture.common.shared.schedule.ResetJobPayload;
import rapture.common.shared.schedule.RetrieveJobExecPayload;
import rapture.common.shared.schedule.DeleteJobPayload;
import rapture.common.shared.schedule.GetJobsPayload;
import rapture.common.shared.schedule.GetUpcomingJobsPayload;
import rapture.common.shared.schedule.GetWorkflowExecsStatusPayload;
import rapture.common.shared.schedule.AckJobErrorPayload;
import rapture.common.shared.schedule.GetNextExecPayload;
import rapture.common.shared.schedule.GetJobExecsPayload;
import rapture.common.shared.schedule.BatchGetJobExecsPayload;
import rapture.common.shared.schedule.IsJobReadyToRunPayload;
import rapture.common.shared.schedule.GetCurrentWeekTimeRecordsPayload;
import rapture.common.shared.schedule.GetCurrentDayJobsPayload;
@SuppressWarnings("all")
public class HttpScheduleApi extends BaseHttpApi implements ScheduleApi, ScriptScheduleApi {
private static final Logger log = Logger.getLogger(HttpScheduleApi.class);
public HttpScheduleApi(HttpLoginApi login) {
super(login, "schedule");
}
private static final class CreateJobTypeReference extends TypeReference {
}
@Override
public RaptureJob createJob(CallingContext context, String jobURI, String description, String scriptURI, String cronExpression, String timeZone, Map jobParams, Boolean autoActivate) {
CreateJobPayload requestObj = new CreateJobPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
requestObj.setDescription(description);
requestObj.setScriptURI(scriptURI);
requestObj.setCronExpression(cronExpression);
requestObj.setTimeZone(timeZone);
requestObj.setJobParams(jobParams);
requestObj.setAutoActivate(autoActivate);
return doRequest(requestObj, "CREATEJOB", new CreateJobTypeReference()); }
private static final class CreateWorkflowJobTypeReference extends TypeReference {
}
@Override
public RaptureJob createWorkflowJob(CallingContext context, String jobURI, String description, String workflowURI, String cronExpression, String timeZone, Map jobParams, Boolean autoActivate, int maxRuntimeMinutes, String appStatusNamePattern) {
CreateWorkflowJobPayload requestObj = new CreateWorkflowJobPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
requestObj.setDescription(description);
requestObj.setWorkflowURI(workflowURI);
requestObj.setCronExpression(cronExpression);
requestObj.setTimeZone(timeZone);
requestObj.setJobParams(jobParams);
requestObj.setAutoActivate(autoActivate);
requestObj.setMaxRuntimeMinutes(maxRuntimeMinutes);
requestObj.setAppStatusNamePattern(appStatusNamePattern);
return doRequest(requestObj, "CREATEWORKFLOWJOB", new CreateWorkflowJobTypeReference()); }
@Override
public void activateJob(CallingContext context, String jobURI, Map extraParams) {
ActivateJobPayload requestObj = new ActivateJobPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
requestObj.setExtraParams(extraParams);
doRequest(requestObj, "ACTIVATEJOB", null); }
@Override
public void deactivateJob(CallingContext context, String jobURI) {
DeactivateJobPayload requestObj = new DeactivateJobPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
doRequest(requestObj, "DEACTIVATEJOB", null); }
private static final class RetrieveJobTypeReference extends TypeReference {
}
@Override
public RaptureJob retrieveJob(CallingContext context, String jobURI) {
RetrieveJobPayload requestObj = new RetrieveJobPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
return doRequest(requestObj, "RETRIEVEJOB", new RetrieveJobTypeReference()); }
private static final class RetrieveJobsTypeReference extends TypeReference> {
}
@Override
public List retrieveJobs(CallingContext context, String uriPrefix) {
RetrieveJobsPayload requestObj = new RetrieveJobsPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setUriPrefix(uriPrefix);
return doRequest(requestObj, "RETRIEVEJOBS", new RetrieveJobsTypeReference()); }
@Override
public void runJobNow(CallingContext context, String jobURI, Map extraParams) {
RunJobNowPayload requestObj = new RunJobNowPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
requestObj.setExtraParams(extraParams);
doRequest(requestObj, "RUNJOBNOW", null); }
@Override
public void resetJob(CallingContext context, String jobURI) {
ResetJobPayload requestObj = new ResetJobPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
doRequest(requestObj, "RESETJOB", null); }
private static final class RetrieveJobExecTypeReference extends TypeReference {
}
@Override
public RaptureJobExec retrieveJobExec(CallingContext context, String jobURI, Long execTime) {
RetrieveJobExecPayload requestObj = new RetrieveJobExecPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
requestObj.setExecTime(execTime);
return doRequest(requestObj, "RETRIEVEJOBEXEC", new RetrieveJobExecTypeReference()); }
@Override
public void deleteJob(CallingContext context, String jobURI) {
DeleteJobPayload requestObj = new DeleteJobPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
doRequest(requestObj, "DELETEJOB", null); }
private static final class GetJobsTypeReference extends TypeReference> {
}
@Override
public List getJobs(CallingContext context) {
GetJobsPayload requestObj = new GetJobsPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETJOBS", new GetJobsTypeReference()); }
private static final class GetUpcomingJobsTypeReference extends TypeReference> {
}
@Override
public List getUpcomingJobs(CallingContext context) {
GetUpcomingJobsPayload requestObj = new GetUpcomingJobsPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETUPCOMINGJOBS", new GetUpcomingJobsTypeReference()); }
private static final class GetWorkflowExecsStatusTypeReference extends TypeReference {
}
@Override
public WorkflowExecsStatus getWorkflowExecsStatus(CallingContext context) {
GetWorkflowExecsStatusPayload requestObj = new GetWorkflowExecsStatusPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETWORKFLOWEXECSSTATUS", new GetWorkflowExecsStatusTypeReference()); }
private static final class AckJobErrorTypeReference extends TypeReference {
}
@Override
public JobErrorAck ackJobError(CallingContext context, String jobURI, Long execTime, String jobErrorType) {
AckJobErrorPayload requestObj = new AckJobErrorPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
requestObj.setExecTime(execTime);
requestObj.setJobErrorType(jobErrorType);
return doRequest(requestObj, "ACKJOBERROR", new AckJobErrorTypeReference()); }
private static final class GetNextExecTypeReference extends TypeReference {
}
@Override
public RaptureJobExec getNextExec(CallingContext context, String jobURI) {
GetNextExecPayload requestObj = new GetNextExecPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
return doRequest(requestObj, "GETNEXTEXEC", new GetNextExecTypeReference()); }
private static final class GetJobExecsTypeReference extends TypeReference> {
}
@Override
public List getJobExecs(CallingContext context, String jobURI, int start, int count, Boolean reversed) {
GetJobExecsPayload requestObj = new GetJobExecsPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
requestObj.setStart(start);
requestObj.setCount(count);
requestObj.setReversed(reversed);
return doRequest(requestObj, "GETJOBEXECS", new GetJobExecsTypeReference()); }
private static final class BatchGetJobExecsTypeReference extends TypeReference> {
}
@Override
public List batchGetJobExecs(CallingContext context, List jobURI, int start, int count, Boolean reversed) {
BatchGetJobExecsPayload requestObj = new BatchGetJobExecsPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setJobURI(jobURI);
requestObj.setStart(start);
requestObj.setCount(count);
requestObj.setReversed(reversed);
return doRequest(requestObj, "BATCHGETJOBEXECS", new BatchGetJobExecsTypeReference()); }
private static final class IsJobReadyToRunTypeReference extends TypeReference {
}
@Override
public Boolean isJobReadyToRun(CallingContext context, String toJobURI) {
IsJobReadyToRunPayload requestObj = new IsJobReadyToRunPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setToJobURI(toJobURI);
return doRequest(requestObj, "ISJOBREADYTORUN", new IsJobReadyToRunTypeReference()); }
private static final class GetCurrentWeekTimeRecordsTypeReference extends TypeReference> {
}
@Override
public List getCurrentWeekTimeRecords(CallingContext context, int weekOffsetfromNow) {
GetCurrentWeekTimeRecordsPayload requestObj = new GetCurrentWeekTimeRecordsPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setWeekOffsetfromNow(weekOffsetfromNow);
return doRequest(requestObj, "GETCURRENTWEEKTIMERECORDS", new GetCurrentWeekTimeRecordsTypeReference()); }
private static final class GetCurrentDayJobsTypeReference extends TypeReference> {
}
@Override
public List getCurrentDayJobs(CallingContext context) {
GetCurrentDayJobsPayload requestObj = new GetCurrentDayJobsPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETCURRENTDAYJOBS", new GetCurrentDayJobsTypeReference()); }
@Override
public RaptureJob createJob(String jobURI, String description, String scriptURI, String cronExpression, String timeZone, Map jobParams, Boolean autoActivate) {
return createJob(null,jobURI,description,scriptURI,cronExpression,timeZone,jobParams,autoActivate); }
@Override
public RaptureJob createWorkflowJob(String jobURI, String description, String workflowURI, String cronExpression, String timeZone, Map jobParams, Boolean autoActivate, int maxRuntimeMinutes, String appStatusNamePattern) {
return createWorkflowJob(null,jobURI,description,workflowURI,cronExpression,timeZone,jobParams,autoActivate,maxRuntimeMinutes,appStatusNamePattern); }
@Override
public void activateJob(String jobURI, Map extraParams) {
activateJob(null,jobURI,extraParams); }
@Override
public void deactivateJob(String jobURI) {
deactivateJob(null,jobURI); }
@Override
public RaptureJob retrieveJob(String jobURI) {
return retrieveJob(null,jobURI); }
@Override
public List retrieveJobs(String uriPrefix) {
return retrieveJobs(null,uriPrefix); }
@Override
public void runJobNow(String jobURI, Map extraParams) {
runJobNow(null,jobURI,extraParams); }
@Override
public void resetJob(String jobURI) {
resetJob(null,jobURI); }
@Override
public RaptureJobExec retrieveJobExec(String jobURI, Long execTime) {
return retrieveJobExec(null,jobURI,execTime); }
@Override
public void deleteJob(String jobURI) {
deleteJob(null,jobURI); }
@Override
public List getJobs() {
return getJobs(null); }
@Override
public List getUpcomingJobs() {
return getUpcomingJobs(null); }
@Override
public WorkflowExecsStatus getWorkflowExecsStatus() {
return getWorkflowExecsStatus(null); }
@Override
public JobErrorAck ackJobError(String jobURI, Long execTime, String jobErrorType) {
return ackJobError(null,jobURI,execTime,jobErrorType); }
@Override
public RaptureJobExec getNextExec(String jobURI) {
return getNextExec(null,jobURI); }
@Override
public List getJobExecs(String jobURI, int start, int count, Boolean reversed) {
return getJobExecs(null,jobURI,start,count,reversed); }
@Override
public List batchGetJobExecs(List jobURI, int start, int count, Boolean reversed) {
return batchGetJobExecs(null,jobURI,start,count,reversed); }
@Override
public Boolean isJobReadyToRun(String toJobURI) {
return isJobReadyToRun(null,toJobURI); }
@Override
public List getCurrentWeekTimeRecords(int weekOffsetfromNow) {
return getCurrentWeekTimeRecords(null,weekOffsetfromNow); }
@Override
public List getCurrentDayJobs() {
return getCurrentDayJobs(null); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy