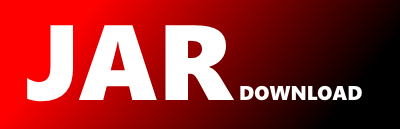
rapture.common.client.HttpSysApi Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This file is autogenerated and any changes will be overwritten.
*/
package rapture.common.client;
import java.util.List;
import java.util.Map;
import rapture.common.api.SysApi;
import rapture.common.api.ScriptSysApi;
import rapture.common.CallingContext;
import rapture.common.model.GeneralResponse;
import rapture.common.model.BasePayload;
import rapture.common.exception.RaptureException;
import rapture.common.impl.jackson.JacksonUtil;
import com.fasterxml.jackson.core.type.TypeReference;
import org.apache.log4j.Logger;
import rapture.common.RaptureFolderInfo;
import rapture.common.NodeEnum;
import rapture.common.ChildrenTransferObject;
import rapture.common.model.DocumentWithMeta;
import rapture.common.ConnectionInfo;
import rapture.common.shared.sys.RetrieveSystemConfigPayload;
import rapture.common.shared.sys.WriteSystemConfigPayload;
import rapture.common.shared.sys.RemoveSystemConfigPayload;
import rapture.common.shared.sys.GetSystemFoldersPayload;
import rapture.common.shared.sys.GetAllTopLevelReposPayload;
import rapture.common.shared.sys.ListByUriPrefixPayload;
import rapture.common.shared.sys.GetChildrenPayload;
import rapture.common.shared.sys.GetAllChildrenPayload;
import rapture.common.shared.sys.GetFolderInfoPayload;
import rapture.common.shared.sys.GetConnectionInfoPayload;
import rapture.common.shared.sys.PutConnectionInfoPayload;
import rapture.common.shared.sys.SetConnectionInfoPayload;
import rapture.common.shared.sys.GetSysDocumentMetaPayload;
@SuppressWarnings("all")
public class HttpSysApi extends BaseHttpApi implements SysApi, ScriptSysApi {
private static final Logger log = Logger.getLogger(HttpSysApi.class);
public HttpSysApi(HttpLoginApi login) {
super(login, "sys");
}
private static final class RetrieveSystemConfigTypeReference extends TypeReference {
}
@Override
public String retrieveSystemConfig(CallingContext context, String area, String path) {
RetrieveSystemConfigPayload requestObj = new RetrieveSystemConfigPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setArea(area);
requestObj.setPath(path);
return doRequest(requestObj, "RETRIEVESYSTEMCONFIG", new RetrieveSystemConfigTypeReference()); }
private static final class WriteSystemConfigTypeReference extends TypeReference {
}
@Override
public String writeSystemConfig(CallingContext context, String area, String path, String content) {
WriteSystemConfigPayload requestObj = new WriteSystemConfigPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setArea(area);
requestObj.setPath(path);
requestObj.setContent(content);
return doRequest(requestObj, "WRITESYSTEMCONFIG", new WriteSystemConfigTypeReference()); }
@Override
public void removeSystemConfig(CallingContext context, String area, String path) {
RemoveSystemConfigPayload requestObj = new RemoveSystemConfigPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setArea(area);
requestObj.setPath(path);
doRequest(requestObj, "REMOVESYSTEMCONFIG", null); }
private static final class GetSystemFoldersTypeReference extends TypeReference> {
}
@Override
public List getSystemFolders(CallingContext context, String area, String path) {
GetSystemFoldersPayload requestObj = new GetSystemFoldersPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setArea(area);
requestObj.setPath(path);
return doRequest(requestObj, "GETSYSTEMFOLDERS", new GetSystemFoldersTypeReference()); }
private static final class GetAllTopLevelReposTypeReference extends TypeReference> {
}
@Override
public List getAllTopLevelRepos(CallingContext context) {
GetAllTopLevelReposPayload requestObj = new GetAllTopLevelReposPayload();
requestObj.setContext(context == null ? this.getContext() : context);
return doRequest(requestObj, "GETALLTOPLEVELREPOS", new GetAllTopLevelReposTypeReference()); }
private static final class ListByUriPrefixTypeReference extends TypeReference {
}
@Override
public ChildrenTransferObject listByUriPrefix(CallingContext context, String raptureURI, String marker, int depth, Long maximum, Long millisUntilCacheExpiry) {
ListByUriPrefixPayload requestObj = new ListByUriPrefixPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setRaptureURI(raptureURI);
requestObj.setMarker(marker);
requestObj.setDepth(depth);
requestObj.setMaximum(maximum);
requestObj.setMillisUntilCacheExpiry(millisUntilCacheExpiry);
return doRequest(requestObj, "LISTBYURIPREFIX", new ListByUriPrefixTypeReference()); }
private static final class GetChildrenTypeReference extends TypeReference {
}
@Override
public ChildrenTransferObject getChildren(CallingContext context, String raptureURI) {
GetChildrenPayload requestObj = new GetChildrenPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setRaptureURI(raptureURI);
return doRequest(requestObj, "GETCHILDREN", new GetChildrenTypeReference()); }
private static final class GetAllChildrenTypeReference extends TypeReference {
}
@Override
public ChildrenTransferObject getAllChildren(CallingContext context, String raptureURI, String marker, Long maximum) {
GetAllChildrenPayload requestObj = new GetAllChildrenPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setRaptureURI(raptureURI);
requestObj.setMarker(marker);
requestObj.setMaximum(maximum);
return doRequest(requestObj, "GETALLCHILDREN", new GetAllChildrenTypeReference()); }
private static final class GetFolderInfoTypeReference extends TypeReference {
}
@Override
public NodeEnum getFolderInfo(CallingContext context, String raptureURI) {
GetFolderInfoPayload requestObj = new GetFolderInfoPayload();
requestObj.setContext(context == null ? this.getContext() : context);
requestObj.setRaptureURI(raptureURI);
return doRequest(requestObj, "GETFOLDERINFO", new GetFolderInfoTypeReference()); }
private static final class GetConnectionInfoTypeReference extends TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy