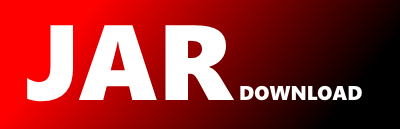
rapture.generated.IdGenLexer Maven / Gradle / Ivy
// $ANTLR 3.5.2 /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g 2016-03-01 15:52:39
package rapture.generated;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings("all")
public class IdGenLexer extends Lexer {
public static final int EOF=-1;
public static final int AWS=4;
public static final int COMMA=5;
public static final int EQUALS=6;
public static final int FILE=7;
public static final int ID=8;
public static final int IDGEN=9;
public static final int LBRACE=10;
public static final int MEMORY=11;
public static final int MONGO=12;
public static final int ON=13;
public static final int RBRACE=14;
public static final int STRING=15;
public static final int USING=16;
public static final int WS=17;
// delegates
// delegators
public Lexer[] getDelegates() {
return new Lexer[] {};
}
public IdGenLexer() {}
public IdGenLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public IdGenLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
@Override public String getGrammarFileName() { return "/Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g"; }
// $ANTLR start "IDGEN"
public final void mIDGEN() throws RecognitionException {
try {
int _type = IDGEN;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:18:7: ( 'IDGEN' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:18:9: 'IDGEN'
{
match("IDGEN");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "IDGEN"
// $ANTLR start "MEMORY"
public final void mMEMORY() throws RecognitionException {
try {
int _type = MEMORY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:20:8: ( 'MEMORY' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:20:10: 'MEMORY'
{
match("MEMORY");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MEMORY"
// $ANTLR start "FILE"
public final void mFILE() throws RecognitionException {
try {
int _type = FILE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:21:6: ( 'FILE' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:21:8: 'FILE'
{
match("FILE");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FILE"
// $ANTLR start "AWS"
public final void mAWS() throws RecognitionException {
try {
int _type = AWS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:22:5: ( 'AWS' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:22:7: 'AWS'
{
match("AWS");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AWS"
// $ANTLR start "MONGO"
public final void mMONGO() throws RecognitionException {
try {
int _type = MONGO;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:23:7: ( 'MONGO' | 'MONGODB' )
int alt1=2;
int LA1_0 = input.LA(1);
if ( (LA1_0=='M') ) {
int LA1_1 = input.LA(2);
if ( (LA1_1=='O') ) {
int LA1_2 = input.LA(3);
if ( (LA1_2=='N') ) {
int LA1_3 = input.LA(4);
if ( (LA1_3=='G') ) {
int LA1_4 = input.LA(5);
if ( (LA1_4=='O') ) {
int LA1_5 = input.LA(6);
if ( (LA1_5=='D') ) {
alt1=2;
}
else {
alt1=1;
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 5 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 1, 4, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 4 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 1, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 1, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 1, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 1, 0, input);
throw nvae;
}
switch (alt1) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:23:9: 'MONGO'
{
match("MONGO");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:23:19: 'MONGODB'
{
match("MONGODB");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MONGO"
// $ANTLR start "LBRACE"
public final void mLBRACE() throws RecognitionException {
try {
int _type = LBRACE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:25:8: ( '{' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:25:10: '{'
{
match('{');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LBRACE"
// $ANTLR start "RBRACE"
public final void mRBRACE() throws RecognitionException {
try {
int _type = RBRACE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:26:8: ( '}' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:26:10: '}'
{
match('}');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "RBRACE"
// $ANTLR start "COMMA"
public final void mCOMMA() throws RecognitionException {
try {
int _type = COMMA;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:27:7: ( ',' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:27:9: ','
{
match(',');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COMMA"
// $ANTLR start "EQUALS"
public final void mEQUALS() throws RecognitionException {
try {
int _type = EQUALS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:28:8: ( '=' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:28:10: '='
{
match('=');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "EQUALS"
// $ANTLR start "USING"
public final void mUSING() throws RecognitionException {
try {
int _type = USING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:29:7: ( 'USING' | 'using' )
int alt2=2;
int LA2_0 = input.LA(1);
if ( (LA2_0=='U') ) {
alt2=1;
}
else if ( (LA2_0=='u') ) {
alt2=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 2, 0, input);
throw nvae;
}
switch (alt2) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:29:9: 'USING'
{
match("USING");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:29:19: 'using'
{
match("using");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "USING"
// $ANTLR start "ON"
public final void mON() throws RecognitionException {
try {
int _type = ON;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:30:3: ( 'ON' | 'on' )
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0=='O') ) {
alt3=1;
}
else if ( (LA3_0=='o') ) {
alt3=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 3, 0, input);
throw nvae;
}
switch (alt3) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:30:5: 'ON'
{
match("ON");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:30:12: 'on'
{
match("on");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ON"
// $ANTLR start "ID"
public final void mID() throws RecognitionException {
try {
int _type = ID;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:32:4: ( ( 'a' .. 'z' | 'A' .. 'Z' | '_' ) ( 'a' .. 'z' | 'A' .. 'Z' | '0' .. '9' | '_' )* )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:32:6: ( 'a' .. 'z' | 'A' .. 'Z' | '_' ) ( 'a' .. 'z' | 'A' .. 'Z' | '0' .. '9' | '_' )*
{
if ( (input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:32:30: ( 'a' .. 'z' | 'A' .. 'Z' | '0' .. '9' | '_' )*
loop4:
while (true) {
int alt4=2;
int LA4_0 = input.LA(1);
if ( ((LA4_0 >= '0' && LA4_0 <= '9')||(LA4_0 >= 'A' && LA4_0 <= 'Z')||LA4_0=='_'||(LA4_0 >= 'a' && LA4_0 <= 'z')) ) {
alt4=1;
}
switch (alt4) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9')||(input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop4;
}
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ID"
// $ANTLR start "STRING"
public final void mSTRING() throws RecognitionException {
try {
int _type = STRING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:33:8: ( '\"' (~ '\"' )* '\"' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:33:10: '\"' (~ '\"' )* '\"'
{
match('\"');
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:33:14: (~ '\"' )*
loop5:
while (true) {
int alt5=2;
int LA5_0 = input.LA(1);
if ( ((LA5_0 >= '\u0000' && LA5_0 <= '!')||(LA5_0 >= '#' && LA5_0 <= '\uFFFF')) ) {
alt5=1;
}
switch (alt5) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '!')||(input.LA(1) >= '#' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop5;
}
}
match('\"');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "STRING"
// $ANTLR start "WS"
public final void mWS() throws RecognitionException {
try {
int _type = WS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:35:4: ( ( ' ' ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:35:6: ( ' ' )
{
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:35:6: ( ' ' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:35:7: ' '
{
match(' ');
}
_channel=HIDDEN;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WS"
@Override
public void mTokens() throws RecognitionException {
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:8: ( IDGEN | MEMORY | FILE | AWS | MONGO | LBRACE | RBRACE | COMMA | EQUALS | USING | ON | ID | STRING | WS )
int alt6=14;
switch ( input.LA(1) ) {
case 'I':
{
int LA6_1 = input.LA(2);
if ( (LA6_1=='D') ) {
int LA6_16 = input.LA(3);
if ( (LA6_16=='G') ) {
int LA6_25 = input.LA(4);
if ( (LA6_25=='E') ) {
int LA6_33 = input.LA(5);
if ( (LA6_33=='N') ) {
int LA6_40 = input.LA(6);
if ( ((LA6_40 >= '0' && LA6_40 <= '9')||(LA6_40 >= 'A' && LA6_40 <= 'Z')||LA6_40=='_'||(LA6_40 >= 'a' && LA6_40 <= 'z')) ) {
alt6=12;
}
else {
alt6=1;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
break;
case 'M':
{
switch ( input.LA(2) ) {
case 'E':
{
int LA6_17 = input.LA(3);
if ( (LA6_17=='M') ) {
int LA6_26 = input.LA(4);
if ( (LA6_26=='O') ) {
int LA6_34 = input.LA(5);
if ( (LA6_34=='R') ) {
int LA6_41 = input.LA(6);
if ( (LA6_41=='Y') ) {
int LA6_47 = input.LA(7);
if ( ((LA6_47 >= '0' && LA6_47 <= '9')||(LA6_47 >= 'A' && LA6_47 <= 'Z')||LA6_47=='_'||(LA6_47 >= 'a' && LA6_47 <= 'z')) ) {
alt6=12;
}
else {
alt6=2;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
break;
case 'O':
{
int LA6_18 = input.LA(3);
if ( (LA6_18=='N') ) {
int LA6_27 = input.LA(4);
if ( (LA6_27=='G') ) {
int LA6_35 = input.LA(5);
if ( (LA6_35=='O') ) {
switch ( input.LA(6) ) {
case 'D':
{
int LA6_48 = input.LA(7);
if ( (LA6_48=='B') ) {
int LA6_52 = input.LA(8);
if ( ((LA6_52 >= '0' && LA6_52 <= '9')||(LA6_52 >= 'A' && LA6_52 <= 'Z')||LA6_52=='_'||(LA6_52 >= 'a' && LA6_52 <= 'z')) ) {
alt6=12;
}
else {
alt6=5;
}
}
else {
alt6=12;
}
}
break;
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
case 'A':
case 'B':
case 'C':
case 'E':
case 'F':
case 'G':
case 'H':
case 'I':
case 'J':
case 'K':
case 'L':
case 'M':
case 'N':
case 'O':
case 'P':
case 'Q':
case 'R':
case 'S':
case 'T':
case 'U':
case 'V':
case 'W':
case 'X':
case 'Y':
case 'Z':
case '_':
case 'a':
case 'b':
case 'c':
case 'd':
case 'e':
case 'f':
case 'g':
case 'h':
case 'i':
case 'j':
case 'k':
case 'l':
case 'm':
case 'n':
case 'o':
case 'p':
case 'q':
case 'r':
case 's':
case 't':
case 'u':
case 'v':
case 'w':
case 'x':
case 'y':
case 'z':
{
alt6=12;
}
break;
default:
alt6=5;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
break;
default:
alt6=12;
}
}
break;
case 'F':
{
int LA6_3 = input.LA(2);
if ( (LA6_3=='I') ) {
int LA6_19 = input.LA(3);
if ( (LA6_19=='L') ) {
int LA6_28 = input.LA(4);
if ( (LA6_28=='E') ) {
int LA6_36 = input.LA(5);
if ( ((LA6_36 >= '0' && LA6_36 <= '9')||(LA6_36 >= 'A' && LA6_36 <= 'Z')||LA6_36=='_'||(LA6_36 >= 'a' && LA6_36 <= 'z')) ) {
alt6=12;
}
else {
alt6=3;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
break;
case 'A':
{
int LA6_4 = input.LA(2);
if ( (LA6_4=='W') ) {
int LA6_20 = input.LA(3);
if ( (LA6_20=='S') ) {
int LA6_29 = input.LA(4);
if ( ((LA6_29 >= '0' && LA6_29 <= '9')||(LA6_29 >= 'A' && LA6_29 <= 'Z')||LA6_29=='_'||(LA6_29 >= 'a' && LA6_29 <= 'z')) ) {
alt6=12;
}
else {
alt6=4;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
break;
case '{':
{
alt6=6;
}
break;
case '}':
{
alt6=7;
}
break;
case ',':
{
alt6=8;
}
break;
case '=':
{
alt6=9;
}
break;
case 'U':
{
int LA6_9 = input.LA(2);
if ( (LA6_9=='S') ) {
int LA6_21 = input.LA(3);
if ( (LA6_21=='I') ) {
int LA6_30 = input.LA(4);
if ( (LA6_30=='N') ) {
int LA6_38 = input.LA(5);
if ( (LA6_38=='G') ) {
int LA6_44 = input.LA(6);
if ( ((LA6_44 >= '0' && LA6_44 <= '9')||(LA6_44 >= 'A' && LA6_44 <= 'Z')||LA6_44=='_'||(LA6_44 >= 'a' && LA6_44 <= 'z')) ) {
alt6=12;
}
else {
alt6=10;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
break;
case 'u':
{
int LA6_10 = input.LA(2);
if ( (LA6_10=='s') ) {
int LA6_22 = input.LA(3);
if ( (LA6_22=='i') ) {
int LA6_31 = input.LA(4);
if ( (LA6_31=='n') ) {
int LA6_39 = input.LA(5);
if ( (LA6_39=='g') ) {
int LA6_45 = input.LA(6);
if ( ((LA6_45 >= '0' && LA6_45 <= '9')||(LA6_45 >= 'A' && LA6_45 <= 'Z')||LA6_45=='_'||(LA6_45 >= 'a' && LA6_45 <= 'z')) ) {
alt6=12;
}
else {
alt6=10;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
else {
alt6=12;
}
}
break;
case 'O':
{
int LA6_11 = input.LA(2);
if ( (LA6_11=='N') ) {
int LA6_23 = input.LA(3);
if ( ((LA6_23 >= '0' && LA6_23 <= '9')||(LA6_23 >= 'A' && LA6_23 <= 'Z')||LA6_23=='_'||(LA6_23 >= 'a' && LA6_23 <= 'z')) ) {
alt6=12;
}
else {
alt6=11;
}
}
else {
alt6=12;
}
}
break;
case 'o':
{
int LA6_12 = input.LA(2);
if ( (LA6_12=='n') ) {
int LA6_24 = input.LA(3);
if ( ((LA6_24 >= '0' && LA6_24 <= '9')||(LA6_24 >= 'A' && LA6_24 <= 'Z')||LA6_24=='_'||(LA6_24 >= 'a' && LA6_24 <= 'z')) ) {
alt6=12;
}
else {
alt6=11;
}
}
else {
alt6=12;
}
}
break;
case 'B':
case 'C':
case 'D':
case 'E':
case 'G':
case 'H':
case 'J':
case 'K':
case 'L':
case 'N':
case 'P':
case 'Q':
case 'R':
case 'S':
case 'T':
case 'V':
case 'W':
case 'X':
case 'Y':
case 'Z':
case '_':
case 'a':
case 'b':
case 'c':
case 'd':
case 'e':
case 'f':
case 'g':
case 'h':
case 'i':
case 'j':
case 'k':
case 'l':
case 'm':
case 'n':
case 'p':
case 'q':
case 'r':
case 's':
case 't':
case 'v':
case 'w':
case 'x':
case 'y':
case 'z':
{
alt6=12;
}
break;
case '\"':
{
alt6=13;
}
break;
case ' ':
{
alt6=14;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 6, 0, input);
throw nvae;
}
switch (alt6) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:10: IDGEN
{
mIDGEN();
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:16: MEMORY
{
mMEMORY();
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:23: FILE
{
mFILE();
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:28: AWS
{
mAWS();
}
break;
case 5 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:32: MONGO
{
mMONGO();
}
break;
case 6 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:38: LBRACE
{
mLBRACE();
}
break;
case 7 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:45: RBRACE
{
mRBRACE();
}
break;
case 8 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:52: COMMA
{
mCOMMA();
}
break;
case 9 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:58: EQUALS
{
mEQUALS();
}
break;
case 10 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:65: USING
{
mUSING();
}
break;
case 11 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:71: ON
{
mON();
}
break;
case 12 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:74: ID
{
mID();
}
break;
case 13 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:77: STRING
{
mSTRING();
}
break;
case 14 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/idgen/IdGenLexer.g:1:84: WS
{
mWS();
}
break;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy