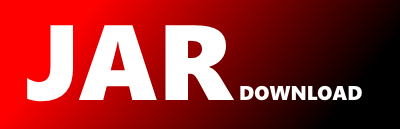
rapture.generated.RapGenLexer Maven / Gradle / Ivy
// $ANTLR 3.5.2 /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g 2016-03-01 15:52:39
package rapture.generated;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings("all")
public class RapGenLexer extends Lexer {
public static final int EOF=-1;
public static final int AWS=4;
public static final int CACHE=5;
public static final int CASSANDRA=6;
public static final int COMMA=7;
public static final int CSV=8;
public static final int EHCACHE=9;
public static final int EQUALS=10;
public static final int FILE=11;
public static final int ID=12;
public static final int JDBC=13;
public static final int LBRACE=14;
public static final int MEMCACHED=15;
public static final int MEMORY=16;
public static final int MONGODB=17;
public static final int NOTHING=18;
public static final int NREP=19;
public static final int ON=20;
public static final int POSTGRES=21;
public static final int QREP=22;
public static final int RBRACE=23;
public static final int READONLY=24;
public static final int REDIS=25;
public static final int REP=26;
public static final int RREP=27;
public static final int SHADOW=28;
public static final int STRING=29;
public static final int USING=30;
public static final int VREP=31;
public static final int VSHADOW=32;
public static final int WITH=33;
public static final int WS=34;
// delegates
// delegators
public Lexer[] getDelegates() {
return new Lexer[] {};
}
public RapGenLexer() {}
public RapGenLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public RapGenLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
@Override public String getGrammarFileName() { return "/Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g"; }
// $ANTLR start "REDIS"
public final void mREDIS() throws RecognitionException {
try {
int _type = REDIS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:18:7: ( 'REDIS' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:18:9: 'REDIS'
{
match("REDIS");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "REDIS"
// $ANTLR start "MEMORY"
public final void mMEMORY() throws RecognitionException {
try {
int _type = MEMORY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:19:8: ( 'MEMORY' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:19:10: 'MEMORY'
{
match("MEMORY");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MEMORY"
// $ANTLR start "MEMCACHED"
public final void mMEMCACHED() throws RecognitionException {
try {
int _type = MEMCACHED;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:20:11: ( 'MEMCACHED' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:20:13: 'MEMCACHED'
{
match("MEMCACHED");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MEMCACHED"
// $ANTLR start "AWS"
public final void mAWS() throws RecognitionException {
try {
int _type = AWS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:21:5: ( 'AWS' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:21:7: 'AWS'
{
match("AWS");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AWS"
// $ANTLR start "MONGODB"
public final void mMONGODB() throws RecognitionException {
try {
int _type = MONGODB;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:22:9: ( 'MONGODB' | 'MONGO' )
int alt1=2;
int LA1_0 = input.LA(1);
if ( (LA1_0=='M') ) {
int LA1_1 = input.LA(2);
if ( (LA1_1=='O') ) {
int LA1_2 = input.LA(3);
if ( (LA1_2=='N') ) {
int LA1_3 = input.LA(4);
if ( (LA1_3=='G') ) {
int LA1_4 = input.LA(5);
if ( (LA1_4=='O') ) {
int LA1_5 = input.LA(6);
if ( (LA1_5=='D') ) {
alt1=1;
}
else {
alt1=2;
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 5 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 1, 4, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 4 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 1, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 1, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 1, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 1, 0, input);
throw nvae;
}
switch (alt1) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:22:11: 'MONGODB'
{
match("MONGODB");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:22:23: 'MONGO'
{
match("MONGO");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MONGODB"
// $ANTLR start "FILE"
public final void mFILE() throws RecognitionException {
try {
int _type = FILE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:23:6: ( 'FILE' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:23:8: 'FILE'
{
match("FILE");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FILE"
// $ANTLR start "EHCACHE"
public final void mEHCACHE() throws RecognitionException {
try {
int _type = EHCACHE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:24:9: ( 'EHCACHE' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:24:11: 'EHCACHE'
{
match("EHCACHE");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "EHCACHE"
// $ANTLR start "JDBC"
public final void mJDBC() throws RecognitionException {
try {
int _type = JDBC;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:25:6: ( 'JDBC' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:25:8: 'JDBC'
{
match("JDBC");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "JDBC"
// $ANTLR start "CASSANDRA"
public final void mCASSANDRA() throws RecognitionException {
try {
int _type = CASSANDRA;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:26:11: ( 'CASSANDRA' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:26:13: 'CASSANDRA'
{
match("CASSANDRA");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "CASSANDRA"
// $ANTLR start "CSV"
public final void mCSV() throws RecognitionException {
try {
int _type = CSV;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:27:5: ( 'CSV' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:27:7: 'CSV'
{
match("CSV");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "CSV"
// $ANTLR start "POSTGRES"
public final void mPOSTGRES() throws RecognitionException {
try {
int _type = POSTGRES;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:28:10: ( 'POSTGRES' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:28:12: 'POSTGRES'
{
match("POSTGRES");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "POSTGRES"
// $ANTLR start "NOTHING"
public final void mNOTHING() throws RecognitionException {
try {
int _type = NOTHING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:29:9: ( 'NOTHING' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:29:11: 'NOTHING'
{
match("NOTHING");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NOTHING"
// $ANTLR start "VREP"
public final void mVREP() throws RecognitionException {
try {
int _type = VREP;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:31:6: ( 'VREP' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:31:8: 'VREP'
{
match("VREP");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "VREP"
// $ANTLR start "REP"
public final void mREP() throws RecognitionException {
try {
int _type = REP;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:32:6: ( 'REP' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:32:8: 'REP'
{
match("REP");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "REP"
// $ANTLR start "NREP"
public final void mNREP() throws RecognitionException {
try {
int _type = NREP;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:33:6: ( 'NREP' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:33:8: 'NREP'
{
match("NREP");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NREP"
// $ANTLR start "QREP"
public final void mQREP() throws RecognitionException {
try {
int _type = QREP;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:34:6: ( 'QREP' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:34:8: 'QREP'
{
match("QREP");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "QREP"
// $ANTLR start "RREP"
public final void mRREP() throws RecognitionException {
try {
int _type = RREP;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:35:6: ( 'RREP' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:35:8: 'RREP'
{
match("RREP");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "RREP"
// $ANTLR start "LBRACE"
public final void mLBRACE() throws RecognitionException {
try {
int _type = LBRACE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:37:8: ( '{' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:37:10: '{'
{
match('{');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LBRACE"
// $ANTLR start "RBRACE"
public final void mRBRACE() throws RecognitionException {
try {
int _type = RBRACE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:38:8: ( '}' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:38:10: '}'
{
match('}');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "RBRACE"
// $ANTLR start "COMMA"
public final void mCOMMA() throws RecognitionException {
try {
int _type = COMMA;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:39:7: ( ',' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:39:9: ','
{
match(',');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COMMA"
// $ANTLR start "EQUALS"
public final void mEQUALS() throws RecognitionException {
try {
int _type = EQUALS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:40:8: ( '=' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:40:10: '='
{
match('=');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "EQUALS"
// $ANTLR start "USING"
public final void mUSING() throws RecognitionException {
try {
int _type = USING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:41:7: ( 'using' | 'USING' )
int alt2=2;
int LA2_0 = input.LA(1);
if ( (LA2_0=='u') ) {
alt2=1;
}
else if ( (LA2_0=='U') ) {
alt2=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 2, 0, input);
throw nvae;
}
switch (alt2) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:41:9: 'using'
{
match("using");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:41:19: 'USING'
{
match("USING");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "USING"
// $ANTLR start "WITH"
public final void mWITH() throws RecognitionException {
try {
int _type = WITH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:42:6: ( 'with' | 'WITH' )
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0=='w') ) {
alt3=1;
}
else if ( (LA3_0=='W') ) {
alt3=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 3, 0, input);
throw nvae;
}
switch (alt3) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:42:8: 'with'
{
match("with");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:42:17: 'WITH'
{
match("WITH");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WITH"
// $ANTLR start "SHADOW"
public final void mSHADOW() throws RecognitionException {
try {
int _type = SHADOW;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:43:8: ( 'shadow' | 'SHADOW' )
int alt4=2;
int LA4_0 = input.LA(1);
if ( (LA4_0=='s') ) {
alt4=1;
}
else if ( (LA4_0=='S') ) {
alt4=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 4, 0, input);
throw nvae;
}
switch (alt4) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:43:10: 'shadow'
{
match("shadow");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:43:21: 'SHADOW'
{
match("SHADOW");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SHADOW"
// $ANTLR start "VSHADOW"
public final void mVSHADOW() throws RecognitionException {
try {
int _type = VSHADOW;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:44:9: ( 'vshadow' | 'VSHADOW' )
int alt5=2;
int LA5_0 = input.LA(1);
if ( (LA5_0=='v') ) {
alt5=1;
}
else if ( (LA5_0=='V') ) {
alt5=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 5, 0, input);
throw nvae;
}
switch (alt5) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:44:11: 'vshadow'
{
match("vshadow");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:44:23: 'VSHADOW'
{
match("VSHADOW");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "VSHADOW"
// $ANTLR start "READONLY"
public final void mREADONLY() throws RecognitionException {
try {
int _type = READONLY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:45:10: ( 'readonly' | 'READONLY' )
int alt6=2;
int LA6_0 = input.LA(1);
if ( (LA6_0=='r') ) {
alt6=1;
}
else if ( (LA6_0=='R') ) {
alt6=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 6, 0, input);
throw nvae;
}
switch (alt6) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:45:12: 'readonly'
{
match("readonly");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:45:25: 'READONLY'
{
match("READONLY");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "READONLY"
// $ANTLR start "CACHE"
public final void mCACHE() throws RecognitionException {
try {
int _type = CACHE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:46:7: ( 'cache' | 'CACHE' )
int alt7=2;
int LA7_0 = input.LA(1);
if ( (LA7_0=='c') ) {
alt7=1;
}
else if ( (LA7_0=='C') ) {
alt7=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 7, 0, input);
throw nvae;
}
switch (alt7) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:46:9: 'cache'
{
match("cache");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:46:19: 'CACHE'
{
match("CACHE");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "CACHE"
// $ANTLR start "ON"
public final void mON() throws RecognitionException {
try {
int _type = ON;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:47:3: ( 'on' | 'ON' )
int alt8=2;
int LA8_0 = input.LA(1);
if ( (LA8_0=='o') ) {
alt8=1;
}
else if ( (LA8_0=='O') ) {
alt8=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 8, 0, input);
throw nvae;
}
switch (alt8) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:47:5: 'on'
{
match("on");
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:47:12: 'ON'
{
match("ON");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ON"
// $ANTLR start "ID"
public final void mID() throws RecognitionException {
try {
int _type = ID;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:50:4: ( ( 'a' .. 'z' | 'A' .. 'Z' | '_' ) ( 'a' .. 'z' | 'A' .. 'Z' | '0' .. '9' | '_' )* )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:50:6: ( 'a' .. 'z' | 'A' .. 'Z' | '_' ) ( 'a' .. 'z' | 'A' .. 'Z' | '0' .. '9' | '_' )*
{
if ( (input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:50:30: ( 'a' .. 'z' | 'A' .. 'Z' | '0' .. '9' | '_' )*
loop9:
while (true) {
int alt9=2;
int LA9_0 = input.LA(1);
if ( ((LA9_0 >= '0' && LA9_0 <= '9')||(LA9_0 >= 'A' && LA9_0 <= 'Z')||LA9_0=='_'||(LA9_0 >= 'a' && LA9_0 <= 'z')) ) {
alt9=1;
}
switch (alt9) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9')||(input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop9;
}
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ID"
// $ANTLR start "STRING"
public final void mSTRING() throws RecognitionException {
try {
int _type = STRING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:51:8: ( '\"' (~ '\"' )* '\"' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:51:10: '\"' (~ '\"' )* '\"'
{
match('\"');
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:51:14: (~ '\"' )*
loop10:
while (true) {
int alt10=2;
int LA10_0 = input.LA(1);
if ( ((LA10_0 >= '\u0000' && LA10_0 <= '!')||(LA10_0 >= '#' && LA10_0 <= '\uFFFF')) ) {
alt10=1;
}
switch (alt10) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '!')||(input.LA(1) >= '#' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop10;
}
}
match('\"');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "STRING"
// $ANTLR start "WS"
public final void mWS() throws RecognitionException {
try {
int _type = WS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:53:4: ( ( ' ' ) )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:53:6: ( ' ' )
{
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:53:6: ( ' ' )
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:53:7: ' '
{
match(' ');
}
_channel=HIDDEN;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WS"
@Override
public void mTokens() throws RecognitionException {
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:8: ( REDIS | MEMORY | MEMCACHED | AWS | MONGODB | FILE | EHCACHE | JDBC | CASSANDRA | CSV | POSTGRES | NOTHING | VREP | REP | NREP | QREP | RREP | LBRACE | RBRACE | COMMA | EQUALS | USING | WITH | SHADOW | VSHADOW | READONLY | CACHE | ON | ID | STRING | WS )
int alt11=31;
alt11 = dfa11.predict(input);
switch (alt11) {
case 1 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:10: REDIS
{
mREDIS();
}
break;
case 2 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:16: MEMORY
{
mMEMORY();
}
break;
case 3 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:23: MEMCACHED
{
mMEMCACHED();
}
break;
case 4 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:33: AWS
{
mAWS();
}
break;
case 5 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:37: MONGODB
{
mMONGODB();
}
break;
case 6 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:45: FILE
{
mFILE();
}
break;
case 7 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:50: EHCACHE
{
mEHCACHE();
}
break;
case 8 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:58: JDBC
{
mJDBC();
}
break;
case 9 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:63: CASSANDRA
{
mCASSANDRA();
}
break;
case 10 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:73: CSV
{
mCSV();
}
break;
case 11 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:77: POSTGRES
{
mPOSTGRES();
}
break;
case 12 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:86: NOTHING
{
mNOTHING();
}
break;
case 13 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:94: VREP
{
mVREP();
}
break;
case 14 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:99: REP
{
mREP();
}
break;
case 15 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:103: NREP
{
mNREP();
}
break;
case 16 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:108: QREP
{
mQREP();
}
break;
case 17 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:113: RREP
{
mRREP();
}
break;
case 18 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:118: LBRACE
{
mLBRACE();
}
break;
case 19 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:125: RBRACE
{
mRBRACE();
}
break;
case 20 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:132: COMMA
{
mCOMMA();
}
break;
case 21 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:138: EQUALS
{
mEQUALS();
}
break;
case 22 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:145: USING
{
mUSING();
}
break;
case 23 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:151: WITH
{
mWITH();
}
break;
case 24 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:156: SHADOW
{
mSHADOW();
}
break;
case 25 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:163: VSHADOW
{
mVSHADOW();
}
break;
case 26 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:171: READONLY
{
mREADONLY();
}
break;
case 27 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:180: CACHE
{
mCACHE();
}
break;
case 28 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:186: ON
{
mON();
}
break;
case 29 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:189: ID
{
mID();
}
break;
case 30 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:192: STRING
{
mSTRING();
}
break;
case 31 :
// /Users/amkimian/Development/cloud/Rapture/Libs/RaptureCore/src/main/antlr3/rapture/dsl/repgen/RapGenLexer.g:1:199: WS
{
mWS();
}
break;
}
}
protected DFA11 dfa11 = new DFA11(this);
static final String DFA11_eotS =
"\1\uffff\13\33\4\uffff\13\33\3\uffff\31\33\2\125\1\33\1\127\4\33\1\135"+
"\5\33\1\143\17\33\1\uffff\1\33\1\uffff\1\33\1\165\3\33\1\uffff\1\171\1"+
"\33\1\173\2\33\1\uffff\2\33\1\u0080\1\u0081\1\33\1\u0083\2\33\2\u0086"+
"\5\33\1\u008c\1\33\1\uffff\2\33\1\u0091\1\uffff\1\33\1\uffff\1\33\1\u0094"+
"\2\33\2\uffff\1\33\1\uffff\2\u0098\1\uffff\4\33\1\u0094\1\uffff\1\33\1"+
"\u009e\2\33\1\uffff\2\33\1\uffff\3\33\1\uffff\2\u00a6\3\33\1\uffff\1\33"+
"\1\u0091\1\u00ab\2\33\1\u00ae\1\u00af\1\uffff\1\u00af\1\33\1\u00b1\1\33"+
"\1\uffff\1\33\1\u00b4\2\uffff\1\u00b1\1\uffff\1\u00b5\1\u00b6\3\uffff";
static final String DFA11_eofS =
"\u00b7\uffff";
static final String DFA11_minS =
"\1\40\2\105\1\127\1\111\1\110\1\104\1\101\2\117\2\122\4\uffff\1\163\1"+
"\123\1\151\1\111\1\150\1\110\1\163\1\145\1\141\1\156\1\116\3\uffff\1\101"+
"\1\105\1\115\1\116\1\123\1\114\1\103\1\102\1\103\1\126\1\123\1\124\2\105"+
"\1\110\1\105\1\151\1\111\1\164\1\124\1\141\1\101\1\150\1\141\1\143\2\60"+
"\1\111\1\60\1\104\1\120\1\103\1\107\1\60\1\105\1\101\1\103\1\123\1\110"+
"\1\60\1\124\1\110\2\120\1\101\1\120\1\156\1\116\1\150\1\110\1\144\1\104"+
"\1\141\1\144\1\150\1\uffff\1\123\1\uffff\1\117\1\60\1\122\1\101\1\117"+
"\1\uffff\1\60\1\103\1\60\1\101\1\105\1\uffff\1\107\1\111\2\60\1\104\1"+
"\60\1\147\1\107\2\60\1\157\1\117\1\144\1\157\1\145\1\60\1\116\1\uffff"+
"\1\131\1\103\1\60\1\uffff\1\110\1\uffff\1\116\1\60\1\122\1\116\2\uffff"+
"\1\117\1\uffff\2\60\1\uffff\1\167\1\127\1\157\1\156\1\60\1\uffff\1\114"+
"\1\60\1\110\1\102\1\uffff\1\105\1\104\1\uffff\1\105\1\107\1\127\1\uffff"+
"\2\60\1\167\1\154\1\131\1\uffff\1\105\2\60\1\122\1\123\2\60\1\uffff\1"+
"\60\1\171\1\60\1\104\1\uffff\1\101\1\60\2\uffff\1\60\1\uffff\2\60\3\uffff";
static final String DFA11_maxS =
"\1\175\1\122\1\117\1\127\1\111\1\110\1\104\1\123\1\117\1\122\1\123\1\122"+
"\4\uffff\1\163\1\123\1\151\1\111\1\150\1\110\1\163\1\145\1\141\1\156\1"+
"\116\3\uffff\1\120\1\105\1\115\1\116\1\123\1\114\1\103\1\102\1\123\1\126"+
"\1\123\1\124\2\105\1\110\1\105\1\151\1\111\1\164\1\124\1\141\1\101\1\150"+
"\1\141\1\143\2\172\1\111\1\172\1\104\1\120\1\117\1\107\1\172\1\105\1\101"+
"\1\103\1\123\1\110\1\172\1\124\1\110\2\120\1\101\1\120\1\156\1\116\1\150"+
"\1\110\1\144\1\104\1\141\1\144\1\150\1\uffff\1\123\1\uffff\1\117\1\172"+
"\1\122\1\101\1\117\1\uffff\1\172\1\103\1\172\1\101\1\105\1\uffff\1\107"+
"\1\111\2\172\1\104\1\172\1\147\1\107\2\172\1\157\1\117\1\144\1\157\1\145"+
"\1\172\1\116\1\uffff\1\131\1\103\1\172\1\uffff\1\110\1\uffff\1\116\1\172"+
"\1\122\1\116\2\uffff\1\117\1\uffff\2\172\1\uffff\1\167\1\127\1\157\1\156"+
"\1\172\1\uffff\1\114\1\172\1\110\1\102\1\uffff\1\105\1\104\1\uffff\1\105"+
"\1\107\1\127\1\uffff\2\172\1\167\1\154\1\131\1\uffff\1\105\2\172\1\122"+
"\1\123\2\172\1\uffff\1\172\1\171\1\172\1\104\1\uffff\1\101\1\172\2\uffff"+
"\1\172\1\uffff\2\172\3\uffff";
static final String DFA11_acceptS =
"\14\uffff\1\22\1\23\1\24\1\25\13\uffff\1\35\1\36\1\37\67\uffff\1\34\1"+
"\uffff\1\16\5\uffff\1\4\5\uffff\1\12\21\uffff\1\21\3\uffff\1\6\1\uffff"+
"\1\10\4\uffff\1\17\1\15\1\uffff\1\20\2\uffff\1\27\5\uffff\1\1\4\uffff"+
"\1\5\2\uffff\1\33\3\uffff\1\26\5\uffff\1\2\7\uffff\1\30\4\uffff\1\7\2"+
"\uffff\1\14\1\31\1\uffff\1\32\2\uffff\1\13\1\3\1\11";
static final String DFA11_specialS =
"\u00b7\uffff}>";
static final String[] DFA11_transitionS = {
"\1\35\1\uffff\1\34\11\uffff\1\16\20\uffff\1\17\3\uffff\1\3\1\33\1\7\1"+
"\33\1\5\1\4\3\33\1\6\2\33\1\2\1\11\1\32\1\10\1\13\1\1\1\25\1\33\1\21"+
"\1\12\1\23\3\33\4\uffff\1\33\1\uffff\2\33\1\30\13\33\1\31\2\33\1\27\1"+
"\24\1\33\1\20\1\26\1\22\3\33\1\14\1\uffff\1\15",
"\1\36\14\uffff\1\37",
"\1\40\11\uffff\1\41",
"\1\42",
"\1\43",
"\1\44",
"\1\45",
"\1\46\21\uffff\1\47",
"\1\50",
"\1\51\2\uffff\1\52",
"\1\53\1\54",
"\1\55",
"",
"",
"",
"",
"\1\56",
"\1\57",
"\1\60",
"\1\61",
"\1\62",
"\1\63",
"\1\64",
"\1\65",
"\1\66",
"\1\67",
"\1\70",
"",
"",
"",
"\1\73\2\uffff\1\71\13\uffff\1\72",
"\1\74",
"\1\75",
"\1\76",
"\1\77",
"\1\100",
"\1\101",
"\1\102",
"\1\104\17\uffff\1\103",
"\1\105",
"\1\106",
"\1\107",
"\1\110",
"\1\111",
"\1\112",
"\1\113",
"\1\114",
"\1\115",
"\1\116",
"\1\117",
"\1\120",
"\1\121",
"\1\122",
"\1\123",
"\1\124",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\126",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\130",
"\1\131",
"\1\133\13\uffff\1\132",
"\1\134",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\136",
"\1\137",
"\1\140",
"\1\141",
"\1\142",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\144",
"\1\145",
"\1\146",
"\1\147",
"\1\150",
"\1\151",
"\1\152",
"\1\153",
"\1\154",
"\1\155",
"\1\156",
"\1\157",
"\1\160",
"\1\161",
"\1\162",
"",
"\1\163",
"",
"\1\164",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\166",
"\1\167",
"\1\170",
"",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\172",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\174",
"\1\175",
"",
"\1\176",
"\1\177",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\u0082",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\u0084",
"\1\u0085",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\u0087",
"\1\u0088",
"\1\u0089",
"\1\u008a",
"\1\u008b",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\u008d",
"",
"\1\u008e",
"\1\u008f",
"\12\33\7\uffff\3\33\1\u0090\26\33\4\uffff\1\33\1\uffff\32\33",
"",
"\1\u0092",
"",
"\1\u0093",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\u0095",
"\1\u0096",
"",
"",
"\1\u0097",
"",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"",
"\1\u0099",
"\1\u009a",
"\1\u009b",
"\1\u009c",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"",
"\1\u009d",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\u009f",
"\1\u00a0",
"",
"\1\u00a1",
"\1\u00a2",
"",
"\1\u00a3",
"\1\u00a4",
"\1\u00a5",
"",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\u00a7",
"\1\u00a8",
"\1\u00a9",
"",
"\1\u00aa",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\u00ac",
"\1\u00ad",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\u00b0",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\1\u00b2",
"",
"\1\u00b3",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"",
"",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"\12\33\7\uffff\32\33\4\uffff\1\33\1\uffff\32\33",
"",
"",
""
};
static final short[] DFA11_eot = DFA.unpackEncodedString(DFA11_eotS);
static final short[] DFA11_eof = DFA.unpackEncodedString(DFA11_eofS);
static final char[] DFA11_min = DFA.unpackEncodedStringToUnsignedChars(DFA11_minS);
static final char[] DFA11_max = DFA.unpackEncodedStringToUnsignedChars(DFA11_maxS);
static final short[] DFA11_accept = DFA.unpackEncodedString(DFA11_acceptS);
static final short[] DFA11_special = DFA.unpackEncodedString(DFA11_specialS);
static final short[][] DFA11_transition;
static {
int numStates = DFA11_transitionS.length;
DFA11_transition = new short[numStates][];
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy