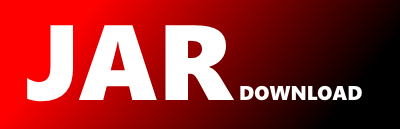
rapture.kernel.ScheduleApiImplWrapper Maven / Gradle / Ivy
/**
* The MIT License (MIT)
*
* Copyright (C) 2011-2016 Incapture Technologies LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* This is an autogenerated file. You should not edit this file as any changes
* will be overwritten.
*/
package rapture.kernel;
import org.apache.log4j.Logger;
import java.util.List;
import java.util.Map;
import rapture.common.api.ScheduleApi;
import rapture.common.CallingContext;
import rapture.common.RaptureEntitlementsContext;
import rapture.common.hooks.CallName;
import rapture.common.model.BasePayload;
import rapture.common.EntitlementSet;
import rapture.kernel.context.ContextValidator;
import rapture.common.RaptureJobExec;
import rapture.common.WorkflowExecsStatus;
import rapture.common.TimedEventRecord;
import rapture.common.JobErrorAck;
import rapture.common.RaptureJob;
import rapture.common.shared.schedule.CreateJobPayload;
import rapture.common.shared.schedule.CreateWorkflowJobPayload;
import rapture.common.shared.schedule.ActivateJobPayload;
import rapture.common.shared.schedule.DeactivateJobPayload;
import rapture.common.shared.schedule.RetrieveJobPayload;
import rapture.common.shared.schedule.RetrieveJobsPayload;
import rapture.common.shared.schedule.RunJobNowPayload;
import rapture.common.shared.schedule.ResetJobPayload;
import rapture.common.shared.schedule.RetrieveJobExecPayload;
import rapture.common.shared.schedule.DeleteJobPayload;
import rapture.common.shared.schedule.GetJobsPayload;
import rapture.common.shared.schedule.GetUpcomingJobsPayload;
import rapture.common.shared.schedule.GetWorkflowExecsStatusPayload;
import rapture.common.shared.schedule.AckJobErrorPayload;
import rapture.common.shared.schedule.GetNextExecPayload;
import rapture.common.shared.schedule.GetJobExecsPayload;
import rapture.common.shared.schedule.BatchGetJobExecsPayload;
import rapture.common.shared.schedule.IsJobReadyToRunPayload;
import rapture.common.shared.schedule.GetCurrentWeekTimeRecordsPayload;
import rapture.common.shared.schedule.GetCurrentDayJobsPayload;
/**
* This class is a wrapper around the class {@link ScheduleApiImpl}. This is an auto-generated class that gives us the ability to add hooks such as entitlmeent
* checks before each function call in the implementation. Since we always want to call these hooks, an instance of this class should be used
* (in {@link Kernel}) instead of using the implementation directly.
* implementation directly.
*
*/
@SuppressWarnings("all")
public class ScheduleApiImplWrapper implements ScheduleApi, KernelApi {
private static final Logger log = Logger.getLogger(ScheduleApiImplWrapper.class);
private ScheduleApiImpl apiImpl;
public ScheduleApiImplWrapper(Kernel kernel) {
apiImpl = new ScheduleApiImpl(kernel);
}
/**
* Returns the underlying implementation object. This should be used when a call is deliberately bypassing entitlement checks.
* @return {@link Schedule}
*/
public ScheduleApiImpl getTrusted() {
return apiImpl;
}
@Override
public void start() {
apiImpl.start();
}
/**
*
*/
@Override
public RaptureJob createJob(CallingContext context, String jobURI, String description, String scriptURI, String cronExpression, String timeZone, Map jobParams, Boolean autoActivate) {
long functionStartTime = System.currentTimeMillis();
CreateJobPayload requestObj = new CreateJobPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
requestObj.setDescription(description);
requestObj.setScriptURI(scriptURI);
requestObj.setCronExpression(cronExpression);
requestObj.setTimeZone(timeZone);
requestObj.setJobParams(jobParams);
requestObj.setAutoActivate(autoActivate);
ContextValidator.validateContext(context, EntitlementSet.Schedule_createJob, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_createJob);
RaptureJob returnValue = apiImpl.createJob(context, jobURI, description, scriptURI, cronExpression, timeZone, jobParams, autoActivate); Kernel.getApiHooksService().post(context, CallName.Schedule_createJob);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.createJob.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.createJob.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public RaptureJob createWorkflowJob(CallingContext context, String jobURI, String description, String workflowURI, String cronExpression, String timeZone, Map jobParams, Boolean autoActivate, int maxRuntimeMinutes, String appStatusNamePattern) {
long functionStartTime = System.currentTimeMillis();
CreateWorkflowJobPayload requestObj = new CreateWorkflowJobPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
requestObj.setDescription(description);
requestObj.setWorkflowURI(workflowURI);
requestObj.setCronExpression(cronExpression);
requestObj.setTimeZone(timeZone);
requestObj.setJobParams(jobParams);
requestObj.setAutoActivate(autoActivate);
requestObj.setMaxRuntimeMinutes(maxRuntimeMinutes);
requestObj.setAppStatusNamePattern(appStatusNamePattern);
ContextValidator.validateContext(context, EntitlementSet.Schedule_createWorkflowJob, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_createWorkflowJob);
RaptureJob returnValue = apiImpl.createWorkflowJob(context, jobURI, description, workflowURI, cronExpression, timeZone, jobParams, autoActivate, maxRuntimeMinutes, appStatusNamePattern); Kernel.getApiHooksService().post(context, CallName.Schedule_createWorkflowJob);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.createWorkflowJob.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.createWorkflowJob.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public void activateJob(CallingContext context, String jobURI, Map extraParams) {
long functionStartTime = System.currentTimeMillis();
ActivateJobPayload requestObj = new ActivateJobPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
requestObj.setExtraParams(extraParams);
ContextValidator.validateContext(context, EntitlementSet.Schedule_activateJob, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_activateJob);
apiImpl.activateJob(context, jobURI, extraParams); Kernel.getApiHooksService().post(context, CallName.Schedule_activateJob);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.activateJob.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.activateJob.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
}
/**
*
*/
@Override
public void deactivateJob(CallingContext context, String jobURI) {
long functionStartTime = System.currentTimeMillis();
DeactivateJobPayload requestObj = new DeactivateJobPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
ContextValidator.validateContext(context, EntitlementSet.Schedule_deactivateJob, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_deactivateJob);
apiImpl.deactivateJob(context, jobURI); Kernel.getApiHooksService().post(context, CallName.Schedule_deactivateJob);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.deactivateJob.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.deactivateJob.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
}
/**
*
*/
@Override
public RaptureJob retrieveJob(CallingContext context, String jobURI) {
long functionStartTime = System.currentTimeMillis();
RetrieveJobPayload requestObj = new RetrieveJobPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
ContextValidator.validateContext(context, EntitlementSet.Schedule_retrieveJob, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_retrieveJob);
RaptureJob returnValue = apiImpl.retrieveJob(context, jobURI); Kernel.getApiHooksService().post(context, CallName.Schedule_retrieveJob);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.retrieveJob.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.retrieveJob.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public List retrieveJobs(CallingContext context, String uriPrefix) {
long functionStartTime = System.currentTimeMillis();
RetrieveJobsPayload requestObj = new RetrieveJobsPayload();
requestObj.setContext(context);
requestObj.setUriPrefix(uriPrefix);
ContextValidator.validateContext(context, EntitlementSet.Schedule_retrieveJobs, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_retrieveJobs);
List returnValue = apiImpl.retrieveJobs(context, uriPrefix); Kernel.getApiHooksService().post(context, CallName.Schedule_retrieveJobs);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.retrieveJobs.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.retrieveJobs.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public void runJobNow(CallingContext context, String jobURI, Map extraParams) {
long functionStartTime = System.currentTimeMillis();
RunJobNowPayload requestObj = new RunJobNowPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
requestObj.setExtraParams(extraParams);
ContextValidator.validateContext(context, EntitlementSet.Schedule_runJobNow, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_runJobNow);
apiImpl.runJobNow(context, jobURI, extraParams); Kernel.getApiHooksService().post(context, CallName.Schedule_runJobNow);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.runJobNow.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.runJobNow.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
}
/**
*
*/
@Override
public void resetJob(CallingContext context, String jobURI) {
long functionStartTime = System.currentTimeMillis();
ResetJobPayload requestObj = new ResetJobPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
ContextValidator.validateContext(context, EntitlementSet.Schedule_resetJob, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_resetJob);
apiImpl.resetJob(context, jobURI); Kernel.getApiHooksService().post(context, CallName.Schedule_resetJob);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.resetJob.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.resetJob.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
}
/**
*
*/
@Override
public RaptureJobExec retrieveJobExec(CallingContext context, String jobURI, Long execTime) {
long functionStartTime = System.currentTimeMillis();
RetrieveJobExecPayload requestObj = new RetrieveJobExecPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
requestObj.setExecTime(execTime);
ContextValidator.validateContext(context, EntitlementSet.Schedule_retrieveJobExec, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_retrieveJobExec);
RaptureJobExec returnValue = apiImpl.retrieveJobExec(context, jobURI, execTime); Kernel.getApiHooksService().post(context, CallName.Schedule_retrieveJobExec);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.retrieveJobExec.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.retrieveJobExec.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public void deleteJob(CallingContext context, String jobURI) {
long functionStartTime = System.currentTimeMillis();
DeleteJobPayload requestObj = new DeleteJobPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
ContextValidator.validateContext(context, EntitlementSet.Schedule_deleteJob, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_deleteJob);
apiImpl.deleteJob(context, jobURI); Kernel.getApiHooksService().post(context, CallName.Schedule_deleteJob);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.deleteJob.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.deleteJob.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
}
/**
*
*/
@Override
public List getJobs(CallingContext context) {
long functionStartTime = System.currentTimeMillis();
GetJobsPayload requestObj = new GetJobsPayload();
requestObj.setContext(context);
ContextValidator.validateContext(context, EntitlementSet.Schedule_getJobs, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_getJobs);
List returnValue = apiImpl.getJobs(context); Kernel.getApiHooksService().post(context, CallName.Schedule_getJobs);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getJobs.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getJobs.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public List getUpcomingJobs(CallingContext context) {
long functionStartTime = System.currentTimeMillis();
GetUpcomingJobsPayload requestObj = new GetUpcomingJobsPayload();
requestObj.setContext(context);
ContextValidator.validateContext(context, EntitlementSet.Schedule_getUpcomingJobs, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_getUpcomingJobs);
List returnValue = apiImpl.getUpcomingJobs(context); Kernel.getApiHooksService().post(context, CallName.Schedule_getUpcomingJobs);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getUpcomingJobs.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getUpcomingJobs.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public WorkflowExecsStatus getWorkflowExecsStatus(CallingContext context) {
long functionStartTime = System.currentTimeMillis();
GetWorkflowExecsStatusPayload requestObj = new GetWorkflowExecsStatusPayload();
requestObj.setContext(context);
ContextValidator.validateContext(context, EntitlementSet.Schedule_getWorkflowExecsStatus, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_getWorkflowExecsStatus);
WorkflowExecsStatus returnValue = apiImpl.getWorkflowExecsStatus(context); Kernel.getApiHooksService().post(context, CallName.Schedule_getWorkflowExecsStatus);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getWorkflowExecsStatus.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getWorkflowExecsStatus.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public JobErrorAck ackJobError(CallingContext context, String jobURI, Long execTime, String jobErrorType) {
long functionStartTime = System.currentTimeMillis();
AckJobErrorPayload requestObj = new AckJobErrorPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
requestObj.setExecTime(execTime);
requestObj.setJobErrorType(jobErrorType);
ContextValidator.validateContext(context, EntitlementSet.Schedule_ackJobError, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_ackJobError);
JobErrorAck returnValue = apiImpl.ackJobError(context, jobURI, execTime, jobErrorType); Kernel.getApiHooksService().post(context, CallName.Schedule_ackJobError);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.ackJobError.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.ackJobError.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public RaptureJobExec getNextExec(CallingContext context, String jobURI) {
long functionStartTime = System.currentTimeMillis();
GetNextExecPayload requestObj = new GetNextExecPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
ContextValidator.validateContext(context, EntitlementSet.Schedule_getNextExec, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_getNextExec);
RaptureJobExec returnValue = apiImpl.getNextExec(context, jobURI); Kernel.getApiHooksService().post(context, CallName.Schedule_getNextExec);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getNextExec.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getNextExec.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public List getJobExecs(CallingContext context, String jobURI, int start, int count, Boolean reversed) {
long functionStartTime = System.currentTimeMillis();
GetJobExecsPayload requestObj = new GetJobExecsPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
requestObj.setStart(start);
requestObj.setCount(count);
requestObj.setReversed(reversed);
ContextValidator.validateContext(context, EntitlementSet.Schedule_getJobExecs, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_getJobExecs);
List returnValue = apiImpl.getJobExecs(context, jobURI, start, count, reversed); Kernel.getApiHooksService().post(context, CallName.Schedule_getJobExecs);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getJobExecs.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getJobExecs.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public List batchGetJobExecs(CallingContext context, List jobURI, int start, int count, Boolean reversed) {
long functionStartTime = System.currentTimeMillis();
BatchGetJobExecsPayload requestObj = new BatchGetJobExecsPayload();
requestObj.setContext(context);
requestObj.setJobURI(jobURI);
requestObj.setStart(start);
requestObj.setCount(count);
requestObj.setReversed(reversed);
ContextValidator.validateContext(context, EntitlementSet.Schedule_batchGetJobExecs, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_batchGetJobExecs);
List returnValue = apiImpl.batchGetJobExecs(context, jobURI, start, count, reversed); Kernel.getApiHooksService().post(context, CallName.Schedule_batchGetJobExecs);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.batchGetJobExecs.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.batchGetJobExecs.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public Boolean isJobReadyToRun(CallingContext context, String toJobURI) {
long functionStartTime = System.currentTimeMillis();
IsJobReadyToRunPayload requestObj = new IsJobReadyToRunPayload();
requestObj.setContext(context);
requestObj.setToJobURI(toJobURI);
ContextValidator.validateContext(context, EntitlementSet.Schedule_isJobReadyToRun, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_isJobReadyToRun);
Boolean returnValue = apiImpl.isJobReadyToRun(context, toJobURI); Kernel.getApiHooksService().post(context, CallName.Schedule_isJobReadyToRun);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.isJobReadyToRun.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.isJobReadyToRun.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public List getCurrentWeekTimeRecords(CallingContext context, int weekOffsetfromNow) {
long functionStartTime = System.currentTimeMillis();
GetCurrentWeekTimeRecordsPayload requestObj = new GetCurrentWeekTimeRecordsPayload();
requestObj.setContext(context);
requestObj.setWeekOffsetfromNow(weekOffsetfromNow);
ContextValidator.validateContext(context, EntitlementSet.Schedule_getCurrentWeekTimeRecords, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_getCurrentWeekTimeRecords);
List returnValue = apiImpl.getCurrentWeekTimeRecords(context, weekOffsetfromNow); Kernel.getApiHooksService().post(context, CallName.Schedule_getCurrentWeekTimeRecords);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getCurrentWeekTimeRecords.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getCurrentWeekTimeRecords.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
/**
*
*/
@Override
public List getCurrentDayJobs(CallingContext context) {
long functionStartTime = System.currentTimeMillis();
GetCurrentDayJobsPayload requestObj = new GetCurrentDayJobsPayload();
requestObj.setContext(context);
ContextValidator.validateContext(context, EntitlementSet.Schedule_getCurrentDayJobs, requestObj);
long preToPostStartTime = System.currentTimeMillis();
Kernel.getApiHooksService().pre(context, CallName.Schedule_getCurrentDayJobs);
List returnValue = apiImpl.getCurrentDayJobs(context); Kernel.getApiHooksService().post(context, CallName.Schedule_getCurrentDayJobs);
long endFunctionTime = System.currentTimeMillis();
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getCurrentDayJobs.fullFunctionTime.succeeded", (endFunctionTime-functionStartTime));
Kernel.getMetricsService().recordTimeDifference("apiMetrics.scheduleApi.getCurrentDayJobs.preToPostTime.succeeded", (endFunctionTime-preToPostStartTime));
return returnValue;}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy